Question
Please provide the implementation of the following #ifndef LIST_H #define LIST_H #include using namespace std; template class List { private: // The basic doubly linked
Please provide the implementation of the following
#ifndef LIST_H
#define LIST_H
#include
using namespace std;
template
class List
{
private:
// The basic doubly linked list node.
// Nested inside of List, can be public
// because the Node is itself private
struct Node
{
Object data;
Node *prev;
Node *next;
Node(const Object &d = Object{}, Node *p = nullptr, Node *n = nullptr)
: data{d}, prev{p}, next{n} {}
Node(Object &&d, Node *p = nullptr, Node *n = nullptr)
: data{std::move(d)}, prev{p}, next{n} {}
};
public:
class const_iterator;
class iterator;
public:
List();
~List();
List(const List &rhs);
List &operator=(const List &rhs);
List(List &&rhs);
List &operator=(List &&rhs);
// Return iterator representing beginning of list.
// Mutator version is first, then accessor version.
iterator begin();
const_iterator begin() const;
// Return iterator representing endmarker of list.
// Mutator version is first, then accessor version.
iterator end();
const_iterator end() const;
// Return number of elements currently in the list.
int size() const;
// Return true if the list is empty, false otherwise.
bool empty() const;
void clear();
// front, back, push_front, push_back, pop_front, and pop_back
// are the basic double-ended queue operations.
Object &front();
const Object &front() const;
Object &back();
const Object &back() const;
void push_front(const Object &x);
void push_back(const Object &x);
void push_front(Object &&x);
void push_back(Object &&x);
void pop_front();
void pop_back();
// Insert x before itr.
iterator insert(iterator itr, const Object &x);
// Insert x before itr.
iterator insert(iterator itr, Object &&x);
// Erase item at itr.
iterator erase(iterator itr);
iterator erase(iterator from, iterator to);
// assignment 01: question 01
iterator find(iterator start, iterator end, const Object &x);
private:
int theSize;
Node *head;
Node *tail;
void init();
};
#endif
// Implement the find function that returns the iterator containing the first occurrence
// of x in the range that begins at start and extends up to but not including end.
// If x is not found, end is returned.
ADD THE CODE HERE FOR THE ABOVE COMMENTS
template
typename List
#include "List.h"
#include "List.cpp"
#include
#include
using namespace std;
template
void printList(List
{
typename List
for (pIter = pList.begin(); pIter != pList.end(); pIter++)
{
cout << *pIter << " ";
}
}
int main()
{
cout << "--------------------------------------------" << endl;
// Add your code here for the implementation
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
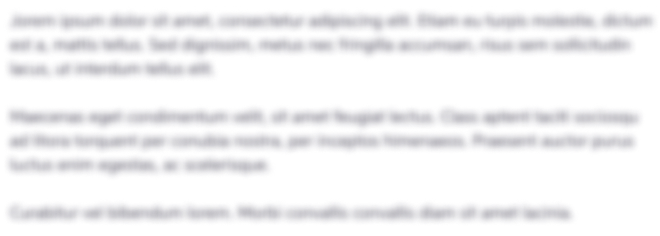
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started