Question
Please read and complete tasks as requested. Task 1: You will modify the add method in the LinkedBag class. Add a second parameter to the
Please read and complete tasks as requested.
Task 1:
You will modify the add method in the LinkedBag class. Add a second parameter to the method header that will be a boolean variable:
public boolean add(T newEntry, boolean sorted)
The modification to the add method will make it possible to add new entries to the beginning of the list, as it does now, but also to add new entries in sorted order. The sorted parameter if set to false will result in the existing functionality being executed (it will add the new element to the beginning of the list), if set to true the new element will be inserted into the list such that the list is in sorted order.
So for example if these are the current entries in the list: 5 -> 8 -> 10 -> 16 -> 25
And the new entry that I want to put into the list is 14, it will put it into the list between 10 and 16: 5 -> 8 -> 10 -> 14 -> 16 -> 25
Remember, this is a generic class so it needs to be able to process any kind of object, just as it does now.
Also, you will need the compareTo method in the Comparable class to compare data items in the nodes when determining where in the list to put new items.
Task 2:
You will need to modify the add method header in the BagInterface class also to include the new parameter.
Task 3:
Create a test program to thoroughly test the add method. Name this file LinkedBagTester.java.
Part of this assignment is to create test cases that test all possible conditions of adding data into the list to verify that the new entry will get placed into the correct place in the list. Also, you need to test the conditions of adding elements only to the beginning of the list so that you verify that your changes did not break the existing functionality.
Submit all three files to the dropbox. Remember, do not zip the files, just upload the three .java files.
LinkedBag class.
/** A class of bags whose entries are stored in a chain of linked nodes. The bag is never full. @author Frank M. Carrano @author Timothy M. Henry @version 4.1 */ public final class LinkedBag
public LinkedBag() { firstNode = null; numberOfEntries = 0; } // end default constructor
/** Sees whether this bag is empty. @return True if this bag is empty, or false if not. */ public boolean isEmpty() { return numberOfEntries == 0; } // end isEmpty
/** Gets the number of entries currently in this bag. @return The integer number of entries currently in this bag. */ public int getCurrentSize() { return numberOfEntries; } // end getCurrentSize
/** Adds a new entry to this bag. @param newEntry The object to be added as a new entry @return True if the addition is successful, or false if not. */ public boolean add(T newEntry) // OutOfMemoryError possible { // Add to beginning of chain: Node newNode = new Node(newEntry); newNode.next = firstNode; // Make new node reference rest of chain // (firstNode is null if chain is empty) firstNode = newNode; // New node is at beginning of chain numberOfEntries++; return true; } // end add
/** Retrieves all entries that are in this bag. @return A newly allocated array of all the entries in this bag. */ public T[] toArray() { // The cast is safe because the new array contains null entries @SuppressWarnings("unchecked") T[] result = (T[])new Object[numberOfEntries]; // Unchecked cast
int index = 0; Node currentNode = firstNode; while ((index < numberOfEntries) && (currentNode != null)) { result[index] = currentNode.getData(); index++; currentNode = currentNode.getNextNode(); } // end while return result; } // end toArray
/** Counts the number of times a given entry appears in this bag. @param anEntry The entry to be counted. @return The number of times anEntry appears in this bag. */ public int getFrequencyOf(T anEntry) { int frequency = 0;
int counter = 0; Node currentNode = firstNode; while ((counter < numberOfEntries) && (currentNode != null)) { if (anEntry.equals(currentNode.getData())) { frequency++; } // end if counter++; currentNode = currentNode.getNextNode(); } // end while
return frequency; } // end getFrequencyOf
/** Tests whether this bag contains a given entry. @param anEntry The entry to locate. @return True if the bag contains anEntry, or false otherwise. */ public boolean contains(T anEntry) { boolean found = false; Node currentNode = firstNode; while (!found && (currentNode != null)) { if (anEntry.equals(currentNode.getData())) found = true; else currentNode = currentNode.getNextNode(); } // end while return found; } // end contains
// Locates a given entry within this bag. // Returns a reference to the node containing the entry, if located, // or null otherwise. private Node getReferenceTo(T anEntry) { boolean found = false; Node currentNode = firstNode; while (!found && (currentNode != null)) { if (anEntry.equals(currentNode.getData())) found = true; else currentNode = currentNode.getNextNode(); } // end while return currentNode; } // end getReferenceTo
/** Removes all entries from this bag. */ public void clear() { while (!isEmpty()) remove(); } // end clear
/** Removes one unspecified entry from this bag, if possible. @return Either the removed entry, if the removal was successful, or null. */ public T remove() { T result = null; if (firstNode != null) { result = firstNode.getData(); firstNode = firstNode.getNextNode(); // Remove first node from chain numberOfEntries--; } // end if
return result; } // end remove
/** Removes one occurrence of a given entry from this bag, if possible. @param anEntry The entry to be removed. @return True if the removal was successful, or false otherwise. */ public boolean remove(T anEntry) { boolean result = false; Node nodeN = getReferenceTo(anEntry); if (nodeN != null) { // Replace located entry with entry in first node nodeN.setData(firstNode.getData());
firstNode = firstNode.getNextNode(); // Remove first node numberOfEntries--; result = true; } // end if return result; } // end remove
private class Node { private T data; // Entry in bag private Node next; // Link to next node
private Node(T dataPortion) { this(dataPortion, null); } // end constructor
private Node(T dataPortion, Node nextNode) { data = dataPortion; next = nextNode; } // end constructor
private T getData() { return data; } // end getData
private void setData(T newData) { data = newData; } // end setData
private Node getNextNode() { return next; } // end getNextNode
private void setNextNode(Node nextNode) { next = nextNode; } // end setNextNode } // end Node } // end LinkedBag
BagInterface class
/** An interface that describes the operations of a bag of objects. @author Frank M. Carrano @author Timothy M. Henry @version 4.1 */ public interface BagInterface
/** Removes one unspecified entry from this bag, if possible. @return Either the removed entry, if the removal. was successful, or null. */ public T remove(); /** Removes one occurrence of a given entry from this bag. @param anEntry The entry to be removed. @return True if the removal was successful, or false if not. */ public boolean remove(T anEntry); /** Removes all entries from this bag. */ public void clear(); /** Counts the number of times a given entry appears in this bag. @param anEntry The entry to be counted. @return The number of times anEntry appears in the bag. */ public int getFrequencyOf(T anEntry); /** Tests whether this bag contains a given entry. @param anEntry The entry to locate. @return True if the bag contains anEntry, or false if not. */ public boolean contains(T anEntry); /** Retrieves all entries that are in this bag. @return A newly allocated array of all the entries in the bag. Note: If the bag is empty, the returned array is empty. */ public T[] toArray(); // public
/** Creates a new bag that combines the contents of this bag and anotherBag. @param anotherBag The bag that is to be added. @return A combined bag. */ // public BagInterface
/** A demonstration of the class LinkedBag. @author Frank M. Carrano @author Timothy M. Henry @version 4.0 */ public class LinkedBagDemo { public static void main(String[] args) { // Tests on a bag that is empty System.out.println("Creating an empty bag."); BagInterface
// Adding strings String[] contentsOfBag = {"A", "D", "B", "A", "C", "A", "D"}; testAdd(aBag, contentsOfBag); // Tests on a bag that is not empty testIsEmpty(aBag, false); String[] testStrings2 = {"A", "B", "C", "D", "Z"}; testFrequency(aBag, testStrings2); testContains(aBag, testStrings2); // Removing strings String[] testStrings3 = {"", "B", "A", "C", "Z"}; testRemove(aBag, testStrings3);
System.out.println(" Clearing the bag:"); aBag.clear(); testIsEmpty(aBag, true); displayBag(aBag); } // end main
// Tests the method add. private static void testAdd(BagInterface
// Tests the two remove methods. private static void testRemove(BagInterface
// Tests the method isEmpty. // correctResult indicates what isEmpty should return. private static void testIsEmpty(BagInterface
// Tests the method getFrequencyOf. private static void testFrequency(BagInterface
// Tests the method contains. private static void testContains(BagInterface
// Tests the method toArray while displaying the bag. private static void displayBag(BagInterface
LinkedBagDemo
/*
Creating an empty bag. The bag contains 0 string(s), as follows: Testing isEmpty with an empty bag: isEmpty finds the bag empty: OK. Testing the method getFrequencyOf: In this bag, the count of is 0 In this bag, the count of B is 0 Testing the method contains: Does this bag contain ? false Does this bag contain B? false Removing a string from the bag: remove() returns null The bag contains 0 string(s), as follows: Removing "B" from the bag: remove("B") returns false The bag contains 0 string(s), as follows: Adding to the bag: A D B A C A D The bag contains 7 string(s), as follows: D A C A B D A Testing isEmpty with a bag that is not empty: isEmpty finds the bag not empty: OK. Testing the method getFrequencyOf: In this bag, the count of A is 3 In this bag, the count of B is 1 In this bag, the count of C is 1 In this bag, the count of D is 2 In this bag, the count of Z is 0 Testing the method contains: Does this bag contain A? true Does this bag contain B? true Does this bag contain C? true Does this bag contain D? true Does this bag contain Z? false Removing a string from the bag: remove() returns D The bag contains 6 string(s), as follows: A C A B D A Removing "B" from the bag: remove("B") returns true The bag contains 5 string(s), as follows: C A A D A Removing "A" from the bag: remove("A") returns true The bag contains 4 string(s), as follows: C A D A Removing "C" from the bag: remove("C") returns true The bag contains 3 string(s), as follows: A D A Removing "Z" from the bag: remove("Z") returns false The bag contains 3 string(s), as follows: A D A Clearing the bag: Testing isEmpty with an empty bag: isEmpty finds the bag empty: OK. The bag contains 0 string(s), as follows:
*/
Step by Step Solution
There are 3 Steps involved in it
Step: 1
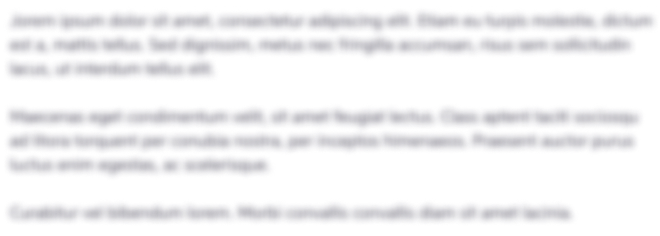
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started