Question
Please read carefully and answer the following questions using the supplied starter code. Please do not use different code. Be sure to match output to
Please read carefully and answer the following questions using the supplied starter code. Please do not use different code. Be sure to match output to the attached sample output as seen below.
Question: Implement your own version of the queue ADT using a circular array. Implement all necessary public methods according to the interface. Additionally, override the toString method. Design and implement a simple pokemon card game.In the game, the player gets 10 random cards and the computer/opponent gets random 10 cards - which are then stored in queues.
Each card's information should be imported from the pokemon.csv file.
In each round, the player and computer's front card in the queue will be compared.
If the player's pokemon's attack value is greater than the computer's pokemon's defense value, then player wins. Otherwise, the computer/opponent wins.
The winner of the round will "win" both cards, so the two cards are inserted back into the winner's queue.
The game should ask for how many rounds to play or end as soon as one of the player's card queue is empty.
SAMPLE CODE:
Queue.java:
public interface Queue{ /** * Place an element at the rear of the queue. * * @param it The element being enqueued. * @throws IllegalStateException- if the element cannot be added at this time due to capacity restrictions */ public void enqueue(E it) throws IllegalStateException;; /** * Remove and return element at the front of the queue. * * @return The element at the front of the queue. * @return null if the queue is empty */ public E dequeue(); /** * @return The front element. * @return null if the queue is empty */ public E frontValue(); /** * Detects whether this queue is empty. * * @return True if the queue is empty. * @return False if the queue has one or more items in it. */ public boolean isEmpty(); /** * @return The number of elements in the queue. */ public int length(); }
Main.java:
import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.Collections; import java.util.List; import java.util.Scanner; public class Main { public static void main(String[] args) { ListallCards = readCSV("pokemon.csv"); Collections.shuffle(allCards); Stack player = new LinkedStack(); Stack computer = new LinkedStack(); for (int i = 0; i readCSV(String filename) { File file = new File(filename); List pokemonList = new ArrayList(); try { Scanner scan = new Scanner(file); scan.nextLine(); while (scan.hasNextLine()) { String[] info = scan.nextLine().split(","); // #,Name,Type 1,Type 2,Total,HP,Attack,Defense,Sp. Atk,Sp. Def,Speed,Generation,Legendary Pokemon poke = new Pokemon(info[1], Integer.parseInt(info[6]),Integer.parseInt(info[7])); pokemonList.add(poke); } } catch (FileNotFoundException e) { System.out.println("File not found!"); e.printStackTrace(); } return pokemonList; } }
ArrayQueue.java:
public class ArrayQueueimplements Queue { private final int DEFAULT_CAPACITY = 7; private T[] queue; private int front, back, count; // front of the queue is left // back of the queue is right public ArrayQueue() { queue = (T[])(new Object[DEFAULT_CAPACITY]); } public ArrayQueue(int size) { queue = (T[])(new Object[size]); } /* What is the big o runtime efficiency/complexity of enqueue in a circular array queue? */ @Override public void enqueue(T it) throws IllegalStateException { if (count
pokemon.csv:
https://gist.github.com/armgilles/194bcff35001e7eb53a2a8b441e8b2c6
SAMPLE OUTPUT (please match):
Welcome to the pokemon battle ground. How many rounds will this battle be? Round \#: 1 Player: Pokemon { name='Electabuzz', attack =83, defense =57} Computer: Pokemon { name='Latias', attack =80, defense =90} \#\#\#\#\# fight! \#\#\#\#\#\# Computer wins this round. Player has 9 cards left. Computer has 11 cards left. Player has 9 cards left. Computer has 11 cards left. Computer wins! Do you want another battle? (1 for yes, any other input will exit the battle.): Round \#: 1 Player: Pokemon { name = Electabuzz', attack =83, defense =57} \#\#\#\#\# fight! \#\#\#\#\#\# Computer wins this round. Player has 9 cards left. Computer has 11 cards left. Player has 9 cards left. Computer has 11 cards left. Computer wins! Do you want another battle? ( 1 for yes, any other input will exit the battle.): Welcome to the pokemon battle ground. How many rounds will this battle be? Round \#: 1 Player: Pokemon { name=' Nidoking', attack=102, defense =77} Computer: Pokemon { name='Pelipper', attack =50, defense =100} \#\#\#\#\# fight! \#\#\#\#\#\# Player wins this round. Player has 11 cards left. Computer has 9 cards left. Round \#: 2 Player: Pokemon \{name='RotomMow Rotom', attack=65, defense=107\} Computer: Pokemon:name='Shiftry', attack=100, defense=60\} \#\#\#\#\# fight! \#\#\#\#\#\# Player wins this round. Player has 12 cards left. Computer has 8 cards left. Player has 12 cards left. Computer has 8 cards left. plaman juincl Round \#: 1 Player: Pokemon { name='Raichu', attack =90, defense =55} Computer: Pokemon { name='Magmar', attack =95, defense =57} \#\#\#\# fight! \#\#\#\#\#\# Player wins this round. Player has 11 cards left. Computer has 9 cards left. Round \#: 2 Player: Pokemon \{name='Plusle', attack=50, defense =40} Computer: Pokemon { name = 'Combee', at tack=30, defense =42} \#\#\#\#\# fight! \#\#\#\#\#\# Player wins this round. Player has 12 cards left. Computer has 8 cards left. Round \#: 3 Player: Pokenon \{nane='Kakuna', attack=25, defense =50} Computer: Pokemon\{name='Floatzel', attack=105, defense=55 Main (3) \#Hfff fight! \#\#\#\#\#\# Computer wins this nound. Player has 11 cards left. Computer has 9 cards left. Round \#: 4 Player: Pokemon \{name= 'Dewgong', attack=70, defense=80\} Computer: Pokemon { name = 'Absol', attack =130, defense =60} \#\#\#\#\# fight! \#\#\#\#\#\# Player wins this round. Player has 12 cards left. Computer has 8 cands left. Round \#: 5 Player: Pokemon \{name='Rhydon', attack=130, defense =120} Computer: Pokemon { name = 'Timbure', attack =80, defense =55} \#\#\#\#\# fight! \#\#\#\#\#\# Player wins this nound. Player has 13 cards left. Computer has 7 cards left. Computer: Pokemontname='Uritblim', attack=80, de+ense =44} \#\#\#\# fight! \#\#\#\#\#\# Player wins this round. Player has 19 cards left. Computer has 1 cards left. Round \#: 26 Player: Pokemon { name='Ariados', attack =90, defense =70} Computer: Pokemon\{name='Drifblim', attack=80, defense =44} \#\#\#\#\# fight! \#\#\#\#\#\# Player wins this round. Player has 20 cards left. Computer has cards left. Player has 20 cards left. Computer has cards left. Player wins! Do you want another battle? (1 for yes, any other input will exit the battle.): Round \#: 26 Player: Pokemon { name='Ariados', attack =90, defense =7} Computer: Pokemon { name = 'Drifblim', attack =80, defense =44} \#\#\#\# fight! \#\#\#\#\#\# Player wins this round. Player has 20 cards left. Computer has cards left. Player has 20 cards left. Computer has 0 cards left. Player wins! Do you want another battle? (1 for yes, any other input will exit the battle.): Welcome to the pokemon battle ground. How many rounds will this battle be? 1000 Main (3) Player hs 1 cards lett. Computer has 19 cards left. Round \#: 85 Player: Pokemon\{name=' Swablu', attack =40, defense =60} Computer: Pokemon { name=' Manectric', attack =75, defense =60} \#\#\#\#\# fight! \#\#\#\#\#\# Computer wins this round. Player has 0 cards left. Computer has 20 cards left. Player has cards left. Computer has 20 cards left. Computer wins! Do you want another battle? (1 for yes, any other input will exit the battle.): Process finished with exit code
Step by Step Solution
There are 3 Steps involved in it
Step: 1
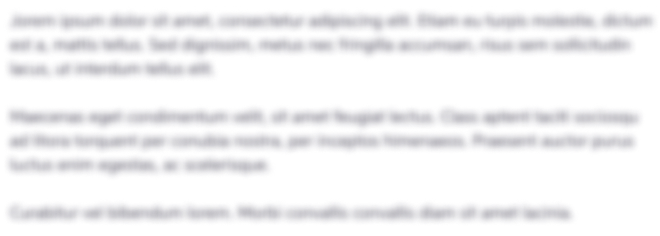
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started