Question
please rewrite the correct code and do not use set ! there are many error in the given code below there is a tester code
please rewrite the correct code and do not use set ! there are many error in the given code below there is a tester code given in python please use visual studio code copy the tester code as tester.py in the code and save the tester code and call the main given code from racket to check whether it is passing all the test cases. Given code: #lang racket ;; Exercises 3: rectangle library, power-set, and inclusion-exclusion principle
(define (rect-x r) (car r)) (define (rect-y r) (car r))
(define (rect-intersect? r1 r2) (and (<= (rect-x r1) (+ (rect-x r2) (rect-width r2))) (<= (rect-y r1) (+ (rect-y r2) (rect-height r2))) (<= (rect-x r2) (+ (rect-x r1) (rect-width r1))) (<= (rect-y r2) (+ (rect-y r1) (rect-height r1)))))
; rect-height: Given a rect?, yield its height (define (rect-height rect) (- (caddr rect) (cadddr rect)))
; rect-width: Given a rect?, yield its width (define (rect-width rect) (- (cadr rect) (car rect)))
(provide rect rect? rect-area rect-intersect rect-list-intersect all-sub-rectangles total-rect-area power-set)
; power-set: takes an arbitrary Racket (set) and returns its power-set, the set of all its subsets (define (power-set st) (if (null? st) (list '()) (let ((rest (power-set (cdr st)))) (append rest (map (lambda (subset) (cons (car st) subset)) rest)))))
; any two opposing corners of a grid-aligned rectangle as pairs (x0,y0), (x1,y1) ; --> `(rect ,lower-left-x ,lower-left-y ,upper-right-x ,upper-right-y) (define (rect x0 y0 x1 y1) (list 'rect (min x0 x1) (min y0 y1) (max x0 x1) (max y0 y1)))
; Predicate defining a rectangle (define (rect? r) (match r [`(rect ,x0 ,y0 ,x1 ,y1) (and (andmap integer? `(,x0 ,x1 ,y0 ,y1)) (<= x0 x1) (<= y0 y1))] [else #f]))
; Given a rect?, yield its (integer?) area (define (rect-area rect) (* (- (caddr rect) (car rect)) (- (cadddr rect) (cadr rect))))
; Compute the rectangular intersection of any two rectangles ; If there is no intersection, return a rectangle with 0 area. (define (make-rect x y width height) (list x y width height))
(define (rect-intersect r1 r2) (make-rect (max (rect-x r1) (rect-x r2)) (max (rect-y r1) (rect-y r2)) (min (+ (rect-x r1) (rect-width r1)) (+ (rect-x r2) (rect-width r2))) (min (+ (rect-y r1) (rect-height r1)) (+ (rect-y r2) (rect-height r2)))))
; Compute the intersection of a list of one or more rectangles ; E.g., the list ((rect 0 0 10 10) (rect 0 -5 10 1) (rect -5 -5 2 5)) ; has intersection (rect 0 0 2 1) (define (rect-list-intersect rect-list) (foldl rect-intersect (car rect-list) (cdr rect-list)))
; Compute a Racket (set) of all sub-rectangles in the given rectangle ; We will call any rectangle r', with integer side-lengths of at least 1, a "sub-rectangle" of r iff r fully contains r' ; E.g., (all-sub-rectangles (rect 0 0 0 0)) => (set) ; E.g., (all-sub-rectangles (rect 0 0 1 1)) => (set `(rect 0 0 1 1)) ; E.g., (all--sub-rectangles (rect 10 5 11 7)) => (set `(rect 10 5 11 7) `(rect 10 5 11 6) `(rect 10 6 11 7)) ; Hint: can you solve this using the `foldl` and `range` functions? (define (all-sub-rectangles r) (define (sub-rectangles-helper i j k l lst) (cond [(> i k) lst] [(>= j l) (sub-rectangles-helper (add1 i) 0 k l lst)] [else (sub-rectangles-helper i (add1 j) k l (cons (rect i j k l) lst))])) (sub-rectangles-helper 0 0 (rect-width r) (rect-height r) empty))
; total-rect-area: takes a list of rectangles (defined in e2) and returns the total covered area ; Note: do not double-count area covered by >1 rectangles ; E.g., (total-rect-area '((rect 0 0 2 2) (rect 1 1 3 3))) => 7 ; Hint: use the power-set function and the inclusion-exclusion principle; review your functions from e2 (define (total-rect-area rect-list) (define (power-set lst) (cond [(empty? lst) (list empty)] [else (let ([rest-set (power-set (rest lst))]) (append rest-set (map (lambda (set) (cons (first lst) set)) rest-set)))])) (define (rect-area r) (* (rect-width r) (rect-height r))) (define (intersection-area r1 r2) (if (rect-intersect? r1 r2) (rect-area (rect-intersect r1 r2)) 0)) (define (inclusion-exclusion areas) (for/sum ([i (in-naturals (length areas))]) (for/sum ([comb (combinations areas i)]) (if (even? i) (apply + comb) (- (apply + comb)))))) (inclusion-exclusion (map rect-area (power-set rect-list)))) ################################################################################################################################################ tester code
#!/usr/bin/python3
# #######################
#
# This file runs tests for this coding assignment.
# Read the file but do not modify.
#
# #######################
#
# Autograde test.py runner
# Some code taken from test.py from submit
#
import os, sys, subprocess, json, argparse, signal
from subprocess import Popen, PIPE, STDOUT, TimeoutExpired
TIMEOUT = 15
#####################
# Start Test Utilities
#####################
def preparefile(file):
pass
def runcmdsafe(binfile):
b_stdout, b_stderr, b_exitcode = runcmd(binfile)
return b_stdout, b_stderr, b_exitcode
def runcmd(cmd):
#executed = Popen(cmd, shell=True, stdin=PIPE, stdout=PIPE, stderr=STDOUT, close_fds=True)
#stdout, stderr = executed.communicate()
#return stdout, stderr, executed.returncode
stdout, stderr = None, None
if os.name != 'nt':
cmd = "exec " + cmd
with Popen(cmd, shell=True, stdin=PIPE, stdout=PIPE, stderr=STDOUT, close_fds=True) as process:
try:
stdout, stderr = process.communicate(timeout=TIMEOUT)
except TimeoutExpired:
if os.name == 'nt':
Popen("TASKKILL /F /PID {pid} /T".format(pid=process.pid))
else:
process.kill()
exit()
return stdout, stderr, process.returncode
def assertequals(expected, actual, info=''):
if expected == actual:
passtest('')
else:
if (info): info = f' {info}'
failtest(f'Expected {expected}, but got {actual}.{info}')
def failtest(message):
testmsg('failed', message)
def passtest(message):
testmsg('passed', message)
def testmsg(status, message):
x = {
"status": status,
"message": message
}
print(json.dumps(x))
sys.exit()
#####################
# End Test Utilities
#####################
verbose = False
def runtest(name):
global verbose
print('---------------------')
print(f' Running test: {name}')
try:
python_bin = sys.executable
output = subprocess.check_output(f'{python_bin} driver.py', cwd=f'test/{name}', shell=True)
y = json.loads(output)
status = y["status"]
message = y["message"]
stdout = output
if verbose and len(message) > 0:
print(" STDOUT: ")
print(message)
if status == "failed":
print(' FAILED')
return False
if status == "passed":
print(' PASSED')
return True
except:
print(' TIMED OUT')
return False
def runtests():
tests = listtests()
num_passed = 0
for test in tests:
if runtest(test):
num_passed += 1
print(' ===========================')
print(f'Summary: {num_passed} / {len(tests)} tests passed')
print('===========================')
def listtests():
tests = [test for test in os.listdir("test/") if not test.startswith(".")]
tests.sort()
return tests
def main():
global verbose
parser = argparse.ArgumentParser()
parser.add_argument('--list', '-l', help='List available tests', action='store_true')
parser.add_argument("--all", "-a", help='Perform all tests', action='store_true')
parser.add_argument('--verbose', '-v', help='View test stdout, verbose output', action='store_true')
parser.add_argument('--test', '-t', help='Perform a specific testname (case sensitive)')
args = parser.parse_args()
if args.verbose:
verbose = True
if args.all:
runtests()
return
if args.test:
if not os.path.exists(f'test/{args.test}'):
print(f'Test "{args.test}" not found')
return
runtest(args.test)
return
if args.list:
print("Available tests: ")
print(*listtests(), sep=' ')
return
parser.print_help()
if __name__ == "__main__": main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
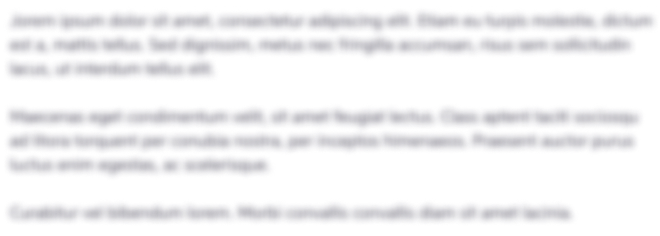
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started