Question
Please show/write step by step on how to set up and connect to database on NETBEANS using JDBC with the following code. I have tried
Please show/write step by step on how to set up and connect to database on NETBEANS using JDBC with the following code.
I have tried but can not connect or set up the database.
I also tried putting in the JAR into the library.
I tried this post suggestion - https://www.chegg.com/homework-help/questions-and-answers/please-show-write-step-step-set-connect-database-netbeans-using-jdbc-following-code-tried--q25326758
This is my output -
--------------------------------------------------------------------
package Database;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Scanner;
public class AnimalDb{
private String name;
private String color;
private String swim;
private String vertibrate;
//creating instance variable
public static Connection getConnection(){
// here we creating static connection method
// when we required connection object that time just call this method
Connection connection=null;
try {
String driver ="com.mysql.jdbc.Driver";
// for mysql driver
String url ="jdbc:mysql://localhost:3306/mydb";
// mydb is my database name
//NOTE:whene you run this you should import jar of mysql-connection
String user ="root";
String password = "root";
// my username and password
Class.forName(driver);
connection = DriverManager.getConnection(url, user, password);
// System.out.println(connection);// driver type
} catch (ClassNotFoundException e)
{
System.out.println("Dao MSG:Excetopn occur at DbConnection class:Class not found exception ");
e.printStackTrace();
} catch (SQLException e) {
System.out.println("Dao MSG:Excetopn occur at DbConnection class:SQL exception ");
e.printStackTrace();
}
return connection;
}
// method for insert record
public void insertData(){
Scanner scan=new Scanner(System.in);
System.out.println("Enter Animal Name");
name=scan.next();
System.out.println("Enter Animal Color");
color=scan.next();
System.out.println("Can This Animal Swim");
swim=scan.next();
System.out.println("Does It is Have a Vertibrate");
vertibrate=scan.next();
try {
final String sql="insert into animal values(?,?,?,?,?)";
// query for insert record
Connection connection=AnimalDb.getConnection();
// calling getconnection() method which is static for get connection object
PreparedStatement ps=connection.prepareStatement(sql); // passing sql query to statement
ps.setInt(1,0);
ps.setString(2,name);
ps.setString(3,color);
ps.setString(4,swim);
ps.setString(5,vertibrate);
int i=ps.executeUpdate();
if(i==1) {
System.out.println("Data Inserted Sucesfully");
}
else {
System.out.println("Data Not Inserted ");
}
}
catch(SQLException ee) {
// for exception handling
ee.getStackTrace();
}
catch (Exception e) {
e.printStackTrace();
// TODO: handle exception
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
Scanner scan = new Scanner(System.in);
char ch;
AnimalDb db=new AnimalDb();
// creating object of class
/* Perform menu operations */
do
{
System.out.println("--------------Animal Database--------------------------");
System.out.println("1. Insert Animal ");
System.out.println("2. Dispay Animal Record ");
System.out.println("3. Search Animal Record ");
System.out.println("4. Update Animal Record ");
System.out.println("5. Delete Animal Record ");
System.out.println("6. Exit");
System.out.println("Enter Your choice");
System.out.println("------------------------------------------------------------");
int choice = scan.nextInt();
switch (choice)
{
case 1 :
//calling method to insert data into db
db.insertData();
break;
case 2 :
// calling method to display record
db.showData();
break;
case 3 :
System.out.println("Enter Name of Animal to Search record");
// asking user to enter record for search
String animalName=scan.next();
boolean result= db.searchData(animalName);
// calling search method
if(result!=true){
System.out.println("Rocord Not found");
}
break;
case 4 :
System.out.println("Enter Name of Animal to update record");
String animalName1=scan.next();
boolean result1= db.searchData(animalName1);
// calling method to update record ,first checking data is present or not
// using search method if present then update
if(result1!=true){
System.out.println("Rocord Not found Update found");
}else{
db.updateData(animalName1);
}
break;
case 5 :
System.out.println("Enter Name of Animal to Delete record");
String animalName2=scan.next();
boolean result2= db.searchData(animalName2);
if(result2!=true){
System.out.println("Rocord Not Found To Delete found");
}else{
db.deleteData(animalName2);
}
break;
case 6:
System.exit(0); // for exit
break;
default :
System.out.println("Wrong Entry ");
// if wrong input
break;
}
System.out.println(" Do you want to continue (Type y or n) ");
ch = scan.next().charAt(0);
} while (ch == 'Y'|| ch == 'y');
}
//method for delete record
public void deleteData(String animalName ) {
Connection conn=null;
try {
final String sql="delete from animal where name='"+animalName+"'";
conn=AnimalDb.getConnection();
PreparedStatement ps=conn.prepareStatement(sql);
int rs=ps.executeUpdate();
if(rs!=0){
System.out.println("Rocord Deleted Successfully");
}
else{
System.out.println("Rocord not Deleted ");
}
}
catch (Exception e) {
e.printStackTrace();
}
}
// method for update records
public void updateData(String animalName) {
Scanner scan=new Scanner(System.in);
// asking user for reenter data for update
System.out.println("ReEnter Animal Color");
color=scan.next();
System.out.println("ReEnter Can This Animal Swim");
swim=scan.next();
System.out.println("ReEnter Does It is Have a Vertibrate");
vertibrate=scan.next();
try {
final String sql="update animal set color=?,swim=?,vertibrate=? where name='"+animalName+"'";
// query for update record
Connection connection=AnimalDb.getConnection();
PreparedStatement ps=connection.prepareStatement(sql);
ps.setString(1,color);
ps.setString(2,swim);
ps.setString(3,vertibrate);
int i=ps.executeUpdate();
if(i!=0) {
System.out.println("Data Updated Sucesfully");
}
else {
System.out.println("Data Not Inserted ");
}
}
catch(Exception ee) {
ee.getStackTrace();
}
}
// method for show records
public void showData() {
Connection conn=null;
try {
final String sql="select * from animal";
// query for select all records
conn=AnimalDb.getConnection();
PreparedStatement ps=conn.prepareStatement(sql);
ResultSet rs=ps.executeQuery();
System.out.println("ID\tName\tColor\tSwim\tvertibrate");
while (rs.next()) {
// display all record one by one
System.out.print(rs.getInt("id")+"\t"+rs.getString("name")+"\t"+rs.getString("color")+"\t"+rs.getString("swim")+"\t"+rs.getString("vertibrate"));
System.out.println();
}
}
catch (SQLException e) {
e.printStackTrace();
}
catch(Exception e) {
e.getStackTrace();
}
}
// metod for search record
public boolean searchData(String animalName) {
boolean result=false;
Connection conn=null;
try {
final String sql="select * from animal where name='"+animalName+"'";
// query for select record by name
conn=AnimalDb.getConnection();// calling connection method to get connection object
PreparedStatement ps=conn.prepareStatement(sql);
ResultSet rs=ps.executeQuery();
if(rs.next()){
// display record from db to prompt
System.out.println("ID\tName\tColor\tSwim\tvertibrate");
System.out.print(rs.getInt("id")+"\t"+rs.getString("name")+"\t"+rs.getString("color")+"\t"+rs.getString("swim")+"\t"+rs.getString("vertibrate"));
System.out.println();
result=true;
}
else{
result=false;
}
}
catch (Exception e) {
e.printStackTrace();
}
return result;
}
}
/* table creation script and output
CREATE TABLE animal (
`id` INT NOT NULL AUTO_INCREMENT,
`name` VARCHAR(45) NULL,
`color` VARCHAR(45) NULL,
`swim` VARCHAR(45) NULL,
`vertibrate` VARCHAR(45) NULL,
PRIMARY KEY (`id`));
*/
To change this license header, choose License Headers in Project Properties To change thia template file, choose ToolaTemplates source Packages database and open the enplae in the edicr imelDb.java Libraries mysdeonnectorjava-5.1-44on.jar R- JDK 1.8 (Default package databaac: e | in port java . sql . Connection; 101mport java.aql. DriverManager: 2mport java.sq1.PreparedStatement: elo World myNumber Project420 ER Singleton database .AnmaDb > name > Output-Database (run) Testrg :D week! weFour - weekoneTest weekThree T1ger Enter Anaral Color Can Thi Animal Suin name-Navigator Animabb Do you want to contine Type y or nl sun. reflect.NetiveConstructorAcces5or Impl .nevInstence(Kst1veConstructorAcce 5 50r Impl. Jara :62) sun 'reflect Del t j.lang.reflect. Consrutor newInstance Constructor.jva:423 t com.my gl jdbe.Ui1 handl-NxIn tance (Util.jus 425) serchDSting animal eme): booleen ssor Impl.nevInstancetDe sorImpl :45) color : String name : String swim String ] veitute : String - e co.ay gi.jdbe.Mys4110.initr Myagiio-j v:841 con.ayaqi.1dbc.Connectionil.connectoaeTryonly IConnectionIpi2-3ava:2222) com.mysq1.1dbc.connection2tp1.1n1>Step by Step Solution
There are 3 Steps involved in it
Step: 1
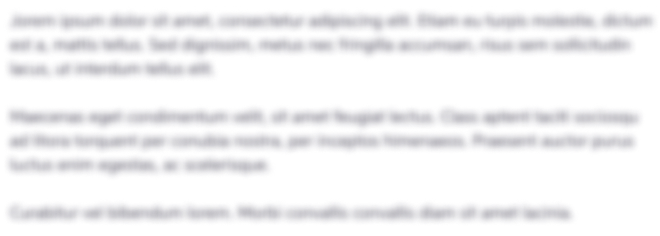
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started