Question
PLEASE SOLVE BOTH OF THESE PROBLEMS AND CODE THEM ACCORDING TO THE STATEMENTS AND THE TEMPLATE FILES. THANK YOU! for problem A this is the
PLEASE SOLVE BOTH OF THESE PROBLEMS AND CODE THEM ACCORDING TO THE STATEMENTS AND THE TEMPLATE FILES. THANK YOU!
for problem A this is the template file they are referring to. "
# -*- coding: utf-8 -*- """ # Programming Assignment 4, Problem A, CSE30-02, Winter 2023 ## README * Please **carefully** follow the instructions given in this template code * Only edit within the allowed sections as instructed by the comments; you are risking your own assignment grade if you make edits outside those sections or deviate from the instructions * The testing script provided at the end only performs some basic tests; it DOES NOT imply anything about your assignment grade * You may add your own tesing code in the main function while working on your assignment * **However**, you will need to remove your own testing code, and re-run the original testing script right before you submit the code, to ensure your code is free from syntax errors """ #===== Import section begins # In this assignment, external libraries are NOT allowed # DO NOT ADD any import statements # keep this section as is #===== Import section ends #===== Existing classes section begins # These class(es) are provided as part of the assignment # DO NOT change or add anything in this section # keep this section as is class ListNode: def __init__(self, val: int, next: 'Union[ListNode, None]'=None) -> None: ''' Initialize the instance of ListNode - Parameters: - val: this value of this node - next: it's either the next node, or None representing the end of the list ''' self.val = val self.next = next def __str__(self): ''' string representation of the instance of ListNode ''' return '{} -> {}'.format(self.val, self.next) #===== Existing classes section ends #===== Helper classes/functions section begins # You may add your own classes or functions within this section # **class** and **function** only! # any statement that is not encapsulated inside a class or function # may result in 0 grade #===== Helper classes/functions section ends
#===== Problem A function begins # follow the instruction below # DO NOT change the function signature! def merge_two_lists(linked_list_1: 'Union[ListNode, None]', linked_list_2: 'Union[ListNode, None]') -> ListNode: ''' Merge two linked list - Parameters: - linked_list_1: the first sorted linked list; None if it's empty - linked_list_2: the second sorted linked list; None if it's empty - Returns: - ListNode: A sorted linked list merged from linked_list_1 and linked_list_2 ''' #===== Your implementation begins here #===== Your implementation ends here pass #===== Problem A function ends #===== Testing scripts main function section begins # follow the instruction below # DO NOT add any statement outside of main() function def main() -> None: # you may add your own testing code within this function while you're # working on your assignment; # however, please remember to remove them, and re-run this testing script # right before you submit your work, in order to ensure your code is # free from syntax error linked_list_1 = ListNode(1, ListNode(3, ListNode(5))) linked_list_2 = ListNode(2, ListNode(4, ListNode(6))) linked_list_12 = ListNode(1, ListNode(2, ListNode(3, ListNode(4, ListNode(5, ListNode(6)))))) linked_list_3 = None linked_list_4 = None linked_list_34 = None linked_list_5 = ListNode(5) linked_list_6 = ListNode(2, ListNode(4, ListNode(6))) linked_list_56 = ListNode(2, ListNode(4, ListNode(5, ListNode(6)))) print('Test case 1') linked_list_your_res = merge_two_lists(linked_list_1, linked_list_2) print('First linked list: ', linked_list_1) print('Second linked list: ', linked_list_2) print('Expected result: ', linked_list_12) print('Your result: ', linked_list_your_res) type_match = type(linked_list_12) == type(linked_list_your_res) type_match_str = '==' if type_match else '!=' print('Return Type: ', 'type(Expected result)', type_match_str, 'type(Your result)') print()
print('Test case 2') linked_list_your_res = merge_two_lists(linked_list_3, linked_list_4) print('First linked list: ', linked_list_3) print('Second linked list: ', linked_list_4) print('Expected result: ', linked_list_34) print('Your result: ', linked_list_your_res) type_match = type(linked_list_34) == type(linked_list_your_res) type_match_str = '==' if type_match else '!=' print('Return Type: ', 'type(Expected result)', type_match_str, 'type(Your result)') print() print('Test case 3') linked_list_your_res = merge_two_lists(linked_list_5, linked_list_6) print('First linked list: ', linked_list_5) print('Second linked list: ', linked_list_6) print('Expected result: ', linked_list_56) print('Your result: ', linked_list_your_res) type_match = type(linked_list_56) == type(linked_list_your_res) type_match_str = '==' if type_match else '!=' print('Return Type: ', 'type(Expected result)', type_match_str, 'type(Your result)') print() if __name__ == '__main__': main() #===== Testing scripts main function section ends
for this problem they are referring to this template file."
# -*- coding: utf-8 -*- """ # Programming Assignment 4, Problem B, CSE30-02, Winter 2023 ## README * Please **carefully** follow the instructions given in this template code * Only edit within the allowed sections as instructed by the comments; you are risking your own assignment grade if you make edits outside those sections or deviate from the instructions * The testing script provided at the end only performs some basic tests; it DOES NOT imply anything about your assignment grade * You may add your own tesing code in the main function while working on your assignment * **However**, you will need to remove your own testing code, and re-run the original testing script right before you submit the code, to ensure your code is free from syntax errors """ #===== Import section begins # In this assignment, external libraries are NOT allowed # DO NOT ADD any import statements # keep this section as is #===== Import section ends #===== Helper classes/functions section begins # You may add your own classes or functions within this section # **class** and **function** only! # any statement that is not encapsulated inside a class or function # may result in 0 grade #===== Helper classes/functions section ends #===== Problem B function begins # follow the instruction below # DO NOT change the function signature! def nested_dict_sum(in_dict: 'Union[int, str, dict]') -> int: ''' Sum of all the positive integers in a dictionary - Parameters: - in_dict: the input, which can either be a int, a str, or a dict - Returns: - int: Sum of all the positive integers in a dictionary ''' #===== Your implementation begins here #===== Your implementation ends here pass #===== Problem B function ends #===== Testing scripts main function section begins # follow the instruction below # DO NOT add any statement outside of main() function
def main() -> None: # you may add your own testing code within this function while you're # working on your assignment; # however, please remember to remove them, and re-run this testing script # right before you submit your work, in order to ensure your code is # free from syntax error dict_1 = 2 res_1 = 2 dict_2 = 'foo' res_2 = 0 dict_3 = { 'a': 2, 'b': {'x': 2}, 'c': {'p': {'h': 2, 'r': 5}}, } res_3 = 11 dict_4 = { 'a': 2, 'b': {'x': 2, 'y': {'foo': 3, 'z': {'bar': 2}}}, 'c': {'p': {'h': 2, 'r': 5}, 'q': 'ball', 'r': 5}, 'd': 1, 'e': {'nn': {'lil': 2}, 'mm': 'car'} } res_4 = 24 print('Test case 1') out_res = nested_dict_sum(dict_1) print('Dictionary: ', dict_1) print('Expected result: ', res_1) print('Your result: ', out_res) type_match = type(res_1) == type(out_res) type_match_str = '==' if type_match else '!=' print('Return Type: ', 'type(Expected result)', type_match_str, 'type(Your result)') print() print('Test case 2') out_res = nested_dict_sum(dict_2) print('Dictionary: ', dict_2) print('Expected result: ', res_2) print('Your result: ', out_res) type_match = type(res_2) == type(out_res) type_match_str = '==' if type_match else '!=' print('Return Type: ', 'type(Expected result)', type_match_str, 'type(Your result)') print() print('Test case 3') out_res = nested_dict_sum(dict_3) print('Dictionary: ', dict_3) print('Expected result: ', res_3) print('Your result: ', out_res) type_match = type(res_3) == type(out_res) type_match_str = '==' if type_match else '!=' print('Return Type: ', 'type(Expected result)', type_match_str, 'type(Your
result)') print() print('Test case 4') out_res = nested_dict_sum(dict_4) print('Dictionary: ', dict_4) print('Expected result: ', res_4) print('Your result: ', out_res) type_match = type(res_4) == type(out_res) type_match_str = '==' if type_match else '!=' print('Return Type: ', 'type(Expected result)', type_match_str, 'type(Your result)') print() if __name__ == '__main__': main() #===== Testing scripts main function section ends"
This problem is about single-linked lists. You are given the heads of two sorted linked lists: linked_list_1 and linked_list_2, containing only integer values and ending with None. Merge the two lists into one sorted list. The list should be made by splicing together the nodes of the first two lists. Return the head of the merged linked list. You must solve this problem using recursion. - Examples Example 1 - linked_list_1: 135 None - linked_list_2: 2 -> 4 -> 6 None - output: 1 > 2 > 3 > 4 > > > None Example 2 - linked_list_1: None - linked_list_2: None - output: None Example 3 - linked_list_1: 5 None - linked_list_2: 246 None - output: 2 > 4 -> 5 > > >one - Please patiently read through and follow the instructions below and the instructions in the template file; otherwise, any violation of these instructions may result in 0 points in this problem - You should use the provided template python file to begin your work the template file can be found in File > ProgrammingAssignment-4 -> merge_two_lists.py - alternatively, you may use the colab notebook version, under the File -> ProgrammingAssignment-4 -> Colab Notebook Version directory. But, after you finish your work, please download the notebook as a python file (NOT a notebook file) - on the menu bar (Colab's menu bar, NOT your browser's menu bar), go to File Download Download .py you are expected to download the template file from Canvas, follow the instructions in that file, work directly in that file, and turn the entire python file in - Notes: - You may assume: - all numbers in both linked lists are integers and unique - Use the ListNode found in the template code to construct linked lists - Do not use any other class to construct/store linked lists You must use recursion to solve the problem - The function 'merge_two_lists' itself must be a recursive function - Recursive function (programming), a function which references itself - source: wikipedia - If you didn't use recursion, or 'merge_two_lists' is not a recursive function, no points will be given EXTERNAL LIBRARIES SHOULD NOT BE USED - Point Allocation (6 points): - 6 points for passing all the test cases - Documentation is necessary ( you should always document your code, even if it's not required!) - Submission Instructions: - A single python (.py) file named "merge_two_lists.py" - Please read and carefully before submitting your assignment - Do a double-check right before submitting the code, to ensure your code is free from syntax errors and aligns with ALL given instructions Problem-B - Recursion on Nested Dictionary Problem Statement: Write a recursive function that will return the sum of all the integer numbers in a dictionary which may contain more dictionaries nested in it. You must solve this problem using recursion. - Examples - Example 1 - in_dict: 2 - output: 2 - Example 2 - in_dict: 'foo' - output: 0 - Example 3 - in_dict: - 1 a:2,b:{xx:2},}c:{p:{ph:2,r:5}}, - output: 11 - Example 4 - in_dict: - - output: 24 - Please patiently read through and follow the instructions below and the instructions in the template file; otherwise, any violation of these instructions may result in 0 points in this problem - You should use the provided template python file to begin your work - the template file can be found in File -> ProgrammingAssignment-4 -> nested_dict_sum.py - alternatively, you may use the colab notebook version, under the File -> ProgrammingAssignment-4 -> Colab Notebook Version directory. But, after you finish your work, please download the notebook as a python file (NOT a notebook file) - on the menu bar (Colab's menu bar, NOT your browser's menu bar), go to File -> Download -> Download .py - you are expected to download the template file from Canvas, follow the instructions in that file, work directly in that file, and turn the entire python fil in - Notes: - You may assume: - All the integers in the dictionary are positive integers - All keys in the dictionary are in string type - The value in the dictionary could either be an integer, a string, or a nested dictionary - for values in string type, you may ignore them, or treat them as 0 - You must use recursion to solve the problem - The function 'nested_dict_sum' itself must be a recursive function - Recursive function (programming), a function which references itself - source: wikipedia B - If you didn't use recursion, or 'nested_dict_sum' is not a recursive function, no points will be given - EXTERNAL LIBRARIES SHOULD NOT BE USED - Point Allocation (6 points): - 6 points for passing all the test cases - Documentation is necessary ( you should always document your code, even if it's not required!) - Submission Instructions: - A single python (.py) file named "nested_dict_sum.py" - Please read this announcement and this announcement carefully before submitting your assignment - Do a double-check right before submitting the code, to ensure your code is free from syntax errors and aligns with ALL given instructionsStep by Step Solution
There are 3 Steps involved in it
Step: 1
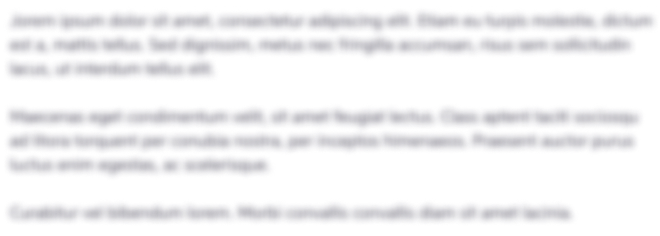
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started