Question
please solve by python 3 Have you ever wondered how social networks, such as Facebook, recommend friends to you? Most of the social networks use
please solve by python 3
Have you ever wondered how social networks, such as Facebook, recommend friends to you? Most of the social networks use highly sophisticated algorithms for this, but for this assignment you will implement a fairly naive algorithm to recommend the most likely new friend to users of a social network. In particular, you will recommend the most probable user to befriend based upon the intersection of your common friends. In other words, the user that you will suggest to Person A is the person who has the most friends in common with Person A, but who currently is not friends with Person A. Five text les have been provided for you to run your program with. Each represents a social network. Three are small test les containing a made-up set of users and their friendships (these les are net1.txt, net2.txt and net3.txt). The two are a subset of a real Facebook dataset, which was obtained from: fhttps://snap.stanford.edu/data/egonets-Facebook.html The format of all ve les is the same: The rst line of the le is an integer representing the number of users in the given network. The following lines are of the form: user_u user_v where user_u and user_v are the (non-negative integer) IDs of two users who are friends. In addition user_u is always less than user_v For example, here is a very small le that has 5 users in the social network: 5
0 1
1 2
1 8
2 3
The above is a representation of a social network that contains 5 users.
User ID=0 is friends with User IDs = 1
User ID=1 is friends with User IDs = 0, 2, 8
User ID=2 is friends with User IDs = 1, 3
User ID=3 is friends with User IDs = 2
User ID=8 is friends with User IDs = 1 Spend time studying the above small example to understand the model. For example, notice that since friendship is a symmetric relationship the social media networks in this assignment, if user_u is friends with user_v, that means that user_v is also friends with user_u. Such duplicate friendships are not present in the le. In particular each friendship is listed once in such way that user_u < user_v
Also note that, while you can assume that user IDs are sorted, you cannot assume that they are consecutive integers diering by one. For example the user IDs above are: 0,1,2,3,8.
You can also assume that in each le the users are sorted from smallest to largest (in the above example you see that users appear as: 0 1 1 2). Specically, friendships of user_u appear before friendships of user_v if and only if user_u < user_v. And also for each user its friends appear sorted, for example for user 1 friendship with friend 2 appears before friendship with friend 4.
To complete the assignment you will have to code the following 9 functions. I strongly recommend you code the in the order given below and do not move onto coding a function until you complete all before. The function descriptions, including what they need to do, are given in a4_xxxxxx.py. 1.
create_network(file_name) (35 points) This is the most important (and possibly the most dicult) function to solve. The function needs to read a le and return a list of tuples representing the social network from the le. In particular the function returns a list of tuples where each tuple has 2 elements: the rst is an integer representing an ID of a user and the second is the list of integers representing his/her friends. In the a4_xxxxxx.py I refer the list that create_network function returns as a 2D-list for friendship network (although one can argue that is is a 3D list). In addition the 2D-list for friendship network that must create_network function returns must be sorted by the ID and a list of friends in each tuple also must be sorted.
So for the example above, this function should return the following 2D-list for 2D-list for friendship network:
[(0, [1]), (1, [0,2,8]), (2,[1,3]), (3,[2]), (8,[1])]
More examples: >>> net1=create_network("net1.txt")
>>> net1 [(0, [1, 2, 3]), (1, [0, 4, 6, 7, 9]), (2, [0, 3, 6, 8, 9]), (3, [0, 2, 8, 9]), (4, [1, 6, 7, 8]), (5, [9]), (6, [1, 2, 4, 8]), (7, [1, 4, 8]), (8, [2, 3, 4, 6, 7]), (9, [1, 2, 3, 5])]
net.txt file contains: 100 10 20 31 41 61 71 92 32 62 82 93 83 94 64 74 58 96 87 8
Step by Step Solution
There are 3 Steps involved in it
Step: 1
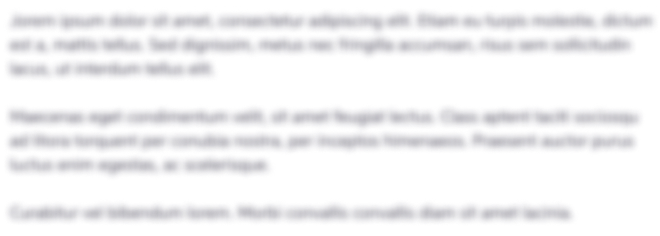
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started