Question
PLEASE START AT PHASE 2 JUST ADDED THE FIRST HALF SO YOU CAN SEE WHAT I'M WORKING WITH The design specifications of a class include:
PLEASE START AT PHASE 2 JUST ADDED THE FIRST HALF SO YOU CAN SEE WHAT I'M WORKING WITH
The design specifications of a class include:
- declarations for a list of instance fields (think of these as nouns) and
- method declarations (think of these as verbs), including parameters, which determine how objects are used.
Implement a class, called Car, with the following instance variables/fields and methods. Exact spelling is required for the name of the Car class and the headers of all the methods within the Car class. Do not make any changes to the following requirements. If you do so, the automated tests that we provide you (see below) will likely fail.
Class Fields/Instance Variables
Declare meaningful names with appropriate data types for each of these private instance variables:
- String for the make of the car (e.g. Ford)
- String for the model of the car (e.g. Explorer)
- an integer for the year of the car
- a double for the odometer
- a double for the mileage of the next oil change
- a double for the gallons of gas in the tank
- a double for miles per gallon
- a constant for the gas tank capacity
private static final double TANK_CAPACITY = 12.5;
- a constant for the number of miles in between oil change.
private static final int MILES_BETWEEN_OIL_CHANGE = 5000;
- an object of the DecimalFormat class to format the numbers (miles and gas)
private static DecimalFormat df = new DecimalFormat ("###,##0.00");
Phase 1 (20 pts)
Constructors
- public Car () this is the default constructor; it assigns the following initial values to the instance variables:
- make: Ford
- model: Explorer
- year of the car: 2020
- miles per gallon: 29.0
- fills the tank
- sets the odometer to zero
- sets the next oil change reminder.
- public Car (String make, String model, int y, double mpg) - this constructor sets the make, model, year and miles per gallon to the provided input parameters and sets the rest of the instance variables to the same values as the default constructor does.
Accessor Methods
- public String getMake ( ) - return the make of the car.
- public String getModel ( ) - return the model of the car.
- public double getMpg ( ) - return the miles per gallon.
- public int getYear ( ) - return the year of the car
- public double getMileageNextOilChange ( ) - return mileage of next oil change
- public double checkOdometer ( ) - return the current mileage.
- public double checkGasGauge ( ) - return the amount of gas in the tank.
Mutator Methods
- public void setMake (String make) set the make of the car to the value of the input parameter
- public void setModel (String model) set the model of the car to the value of the input parameter
- public void setMpg (double value) set the miles per gallon of the car to the value of the input parameter
- public void setYear (int y) set the year of the car to the value of the input parameter
- public void honkHorn ( ) - print a message more creative than "beep beep!
Phase 2 (30 pts)
More Methods - continuation
Note:
The following methods should output simple text messages on the screen for feedback, when appropriate. ALWAYS include the make, model and year of the car when printing messages about the car. Use the DecimalFormat class to format the odometer and gas in tank to include the commas and the two decimals as needed.
- public void addGas (double gallons) add the provided input parameter gallons of gas to the tank. Check for three possibilities, 1) provide a warning message if the value of the input parameter is negative and do not modify the gas tank; 2) provide a warning message if the number of gallons entered as input parameter results in the tank overflowing but still fill the tank; or 3) add the provided number of gallons to the tank. For all conditions, print a message showing the amount of gas in the tank after the fill up. Examples:
- Ford Explorer 2020 gallons cannot be a negative number - Gas in Tank after the fill up: 12.36
- Ford Explorer 2020 tank overflowed - Gas in Tank after the fill up: 12.50
- Ford Explorer 2020 added gas: 2.00 - Gas in Tank after the fill up: 11.05
- public void drive (double miles) this method is used to drive a given number of miles. It checks three possibilities: 1) print a warning message if the parameter is negative and do not update the instance of the Car; 2) print a warning message if the car runs out of gas during the trip and the miles driven. Only add the actual miles driven before running out of gas to the odometer and update the gas in the tank. Or 3) modify the odometer and amount of gas in the tank to reflect the miles driven and print an appropriate message. Think carefully about the expressions to update the gas in the tank and the odometer.
Examples:
- Ford Explorer 2020 cannot drive negative miles.
- Ford Explorer 2020 ran out of gas after driving 320.50 miles.
- Ford Explorer 2020 drove 100.00 miles.
- public void changeOil ( ) - set the oil change reminder to 5,000 miles from the current odometer reading. Print an appropriate message that the oil was changed and when it should be next changed.
Example:
Ford Explorer 2020 oil changed, next mileage to change oil is: 15,424.50
- public void checkOil ( ) Print one of two options depending on how the odometer compares to the next oil change reminder: 1) It is time to change the oil, or 2) Oil is OK, no need to change.
Examples:
- Ford Explorer 2020 - It is time to change the oil.
- Ford Explorer 2020 - Oil is OK, no need to change.
- public String toString ( ) return (dont print) a formatted String of the car. Use the DecimalFormat class to format the odometer and gas in tank to include the commas and the two decimals as needed.
Example:
Ford Explorer 2020 Odometer: 424.50 Gas in Tank: 12.36
- public boolean equals (Car other) return true if the make, model and year of a car are the same as the make, model and year of the other car passed as input parameter. Return false otherwise.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
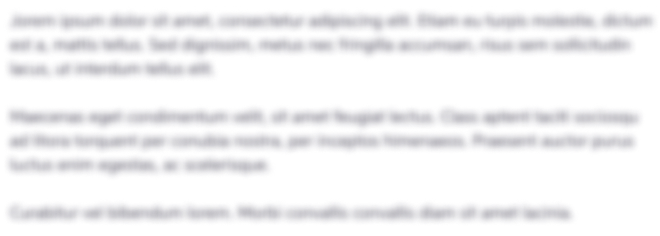
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started