Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Please submit the following files: - all java code files you code - the snapshots of running results using the Test Java code - the
Please submit the following files:
all java code files you code
the snapshots of running results using the Test Java code
the snapshots of running results of the following method
public void displayNumberOfOrdersProduct product
public void displayTotalQuantityOfProducts
The deadline is : pm Saturday, May
Assignment :
exercise : Implementing the Collections in the Gourmet Coffee System
you should:
implement classes in blue
add remove method for each collection class, such as Catalg, implement Iterable interface and iterator method
modify TestXX classes, use foreach loop instead of using iterator, use template for collection object, add test methods for remove
implement mehods displayNumberOfOrdersProduct product and displayTotalQuantityOfProducts
Implementing the Collections in the Gourmet Coffee System
Prerequisites, Goals, and Outcomes
Prerequisites: Before you begin this exercise, you need mastery of the following:
Collections
Use of vectors
Use of iterators
Goals: Reinforce your ability to implement classes that use collections
Outcomes: You will demonstrate mastery of the following:
Implementing a Java class that uses collections
Background
In this assignment, you will implement the classes in the Gourmet Coffee System that use collections.
Description
The following class diagram of the Gourmet Coffee System highlights the classes that use collections:
Figure Gourmet Coffee System class diagram
Complete implementations of the following classes are provided in the student archive:
Coffee
CoffeeBrewer
Product
OrderItem
GourmetCoffee
In this assignment, you will implement the following classes:
Catalog
Order
Sales
GourmetCoffee
The class specifications are as follows:
Class Catalog
The class Catalog models a product catalog.
Instance variables:
products A vector that contains references to instances of class Product.
Constructor and public methods:
public Catalog Creates the vector products, which is initially empty.
public void addProductProduct product Adds the specified product to the vector products.
public Iterator getProductsIterator Returns an iterator over the instances in the vector products.
public Product getProductString code Returns a reference to the Product instance with the specified code. Returns null if there are no products in the catalog with the specified code.
public int getNumberOfProducts Returns the number of instances in the vector products.
Class Order
The class Order maintains a list of order items.
Instance variables:
items A vector that contains references to instances of class OrderItem.
Constructor and public methods:
public Order Creates the vector items, which is initially empty.
public void addItemOrderItem orderItem Adds the specified order item to the vector items.
public void removeItemOrderItem orderItem Removes the specified order item from the vector items.
public Iterator getItemsIterator Returns an iterator over the instances in the vector items.
public OrderItem getItemProduct product Returns a reference to the OrderItem instance with the specified product. Returns null if there are no items in the order with the specified product.
public int getNumberOfItems Returns the number of instances in the vector items.
public double getTotalCost Returns the total cost of the order.
Class Sales
The class Sales maintains a list of the orders that have been completed.
Instance variables:
orders A vector that contains references to instances of class Order.
Constructor and public methods:
public Sales Creates the vector orders, which is initially empty.
public void addOrderOrder order Adds the specified order to the vector orders.
public Iterator getOrdersIterator Returns an iterator over the instances in the vector orders.
public int getNumberOfOrders Returns the number of instances in the vector orders.
Class GourmetCoffee
The class GourmetCoffee creates a console interface to process store orders. Currently, it includes the complete implementation of some of the methods. The methods displayNumberOfOrders and displayTotalQuantityOfProducts are incomplete and should be implemented. The following is a screen shot of the interface:
Figure Execution of GourmetCoffee
Instance variables:
catalog A Catalog object with the products that can be sold.
currentOrder An Order object with the information about the current order.
sales A Sales object with information about all the orders sold by the store.
Constructor and public methods:
public GourmetCoffeeSolution Initializes the attributes catalog, currentOrder and sales. This constructor is complete and should not be modified.
public void displayCatalog Displays the catalog. This method is complete and should not be modified.
public void displayProductInfo Prompts the user for a product code and displays information about the specified product. This method is complete and should not be modified.
public void displayOrder Displays the products in the current order. This method is complete and should not be modified.
public void addModifyProduct Prompts the user for a product code and quantity. If the specified product is not already part of the order, it is added; otherwise, the quantity of the product is updated. This method is complete and should not be modified.
public void removeProduct Prompts the user for a product code and removes the specified product from the current order. This method is complete and should not be modified.
public void saleOrder Registers the sale of the current order. This method is complete and should not be modified.
public void displayOrdersSold Displays the orders that have been sold. This method is complete and should not be modified.
public void displayNumberOfOrdersProduct product Displays the number of orders that contain the specified product. This method is incomplete and should be implemented.
public void displayTotalQuantityOfProducts Displays the total quantity sold for each product in the catalog. This method is incomplete and should be implemented.
Test driver classes
Complete implementations of the following test drivers are provided in the student archive:
Class TestCatalog
Class TestOrder
Class TestSales
Files
The following files are needed to complete this assignment:
studentfiles.zip Download this file. This archive contains the following:
Class files
Coffee.class
CoffeeBrewer.class
Product.class
OrderItem.class
Documentation
Coffee.html
CoffeeBrewer.html
Product.html
OrderItem.html
Java files
GourmetCoffee.java An incomplete implementation.
TestCatalog.java A complete implementation.
TestOrder.java A complete implementation.
TestSales.java A complete implementation.
Tasks
Implement classes Catalog, Order, and Sales. Document your code using Javadoc and follow Sun's code conventions. The following steps will guide you through this assignment. Work incrementally and test each increment. Save often.
Extract the files by issuing the following command at the command prompt.
C:unzip studentfiles.zip
Then, implement class Catalog from scratch. Use TestCatalog to test your implementation.
Next, implement class Order from scratch. Use TestOrder to test your implementation.
Then, implement class Sales from scratch. Use TestSales to test your implementation.
Finally, complete class GourmetCoffee. It uses a Catalog object created by method GourmetCoffee.loadCatalog and a Sales object generated by method GourmetCoffee.loadSales. To complete class GourmetCoffee, implement the following methods:
public void displayNumberOfOrdersProduct product This method displays the number of orders in the sales object that contain the specified product. Compile and execute the class GourmetCoffee. Verify that the method displayNumberOfOrders works correctly. The following is the output that should be displayed for the product with product code A and the orders preloaded by the method loadSales:
Quit
Display catalog
Display product
Display current order
Addmodify product toin current order
Remove product from current order
Register sale of current order
Display sales
Display number of orders with a specific product
Display the total quantity sold for each product
choice
Product code A
Number of orders that contains the product A:
public void displayTotalQuantityOfProducts This method displays the total quantity sold for each product in the catalog. The information of each product must be displayed on a single line in the following format:
ProductCode Quantity
The following is a description of the information included in the format above:
ProductCode the code of the product
Quantity the total quantity of product that has been sold in the store
Compile and execute the class GourmetCoffee. Verify that the method displayTotalQuantityOfProducts works correctly. The following is the output that should be displayed for the orders preloaded by the method loadSales:
Quit
Display catalog
Display product
Display current order
Addmodify product toin current order
Remove product from current order
Register sale of current order
Display sales
Display number of orders with a specific product
Display the total quantity sold for each product
choice
C
C
C
C
C
B
B
B
A
A
A
A
A
Submission
Upon completion, submit only the following.
Catalog.java
Order.java
Sales.java
GourmetCoffee.java

Step by Step Solution
There are 3 Steps involved in it
Step: 1
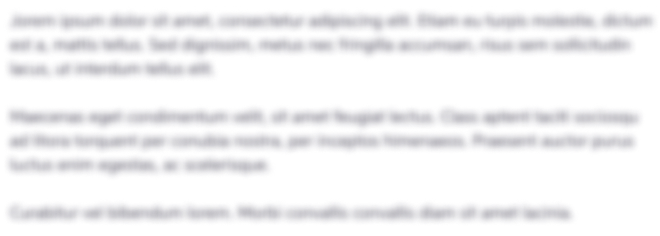
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started