Question
PLEASE TEST YOUR ANSWER/CODE WITH GIVEN TESTER FILE AND THEN POST YOUR ANSWER HERE. -- Instruction -- Given: You are given a Python Class template.
PLEASE TEST YOUR ANSWER/CODE WITH GIVEN TESTER FILE AND THEN POST YOUR ANSWER HERE.
-- Instruction --
Given: You are given a Python Class template. In this template, there 3 classes: MyQueue, MyStack. The goal of this lab is to implement sequential stacks and queues. Task:
- First, create a method called getName() that returns your name. If this does not work, you will receive a mark of zero.
- You are to create Queue and Stack ADTs using a sequential implementation.
- A class called MyQueue is given. You are to implement the methods enqueue(), dequeue(), and the over loaded __len__() method. Enqueue should add an item to the queue. Dequeue should remove an item from the queue. The function __len__() should return the number of items in the queue. All methods MUST be O(1).
- A class called MyStack is given. You are to implement the methods push(), pop(), and the over loaded __len__() method. Push should add an item to the stack. Pop should remove an item from the stack. The function ___len__() should return the number of items in the stack. All methods MUST be O(1). Rule: You MUST use Python's list in your implementation. However you cannot use any built-in list methods such as append, pop, clear, etc. You are also not allow to use any splicing. The only permissible way to manipulate a list is to access it through it's index. Example: array[i] = x
-- Start Up File --
def getName(): return "Last name, first name" class MyQueue: def __init__(self, data=None, maxSize = 100000): # Initialize this queue, and store data if it exists pass
def enqueue(self, data): # Add data to the end of the queue pass
def dequeue(self): # Return the data in the element at the beginning of the queue, or None if the queue is empty pass
def __len__(self): # Return the number of elements in the stack pass
class MyStack: def __init__(self, data=None, maxSize = 100000): # Initialize this stack, and store data if it exists pass def push(self, data): # Add data to the beginning of the stack pass
def pop(self): # Return the data in the element at the beginning of the stack, or None if the stack is empty pass
def __len__(self): # Return the number of elements in the stack pass
-- Tester File --
#Module Imports
import string
import sys
from importlib import import_module
#Testing Imports
import random
def Test(lib, seed=0, size=10, rounds=10, verbose=False):
if not lib:
print("You need to include a testable library")
return False
random.seed(a=seed)
flag = True
try:
queue = lib.MyQueue(data=3, maxSize=3)
queue
except:
if verbose:
print("Error: Queue initialization incomplete")
flag = False
try:
assert(len(queue) == 1 and queue.dequeue() == 3)
except:
if verbose:
print("Error: Initial enqueue missing")
flag = False
try:
queue.enqueue(1)
except:
if verbose:
print("Error: Queue push incomplete.")
flag = False
try:
queue.enqueue(2)
queue.enqueue(3)
queue.enqueue(4)
assert(len(queue) == 3 and queue.dequeue() == 1)
except:
if verbose:
print("Error: Queue accepts elements beyond maxSize")
flag = False
try:
queue = lib.MyQueue()
queue
except:
if verbose:
print("Error: Queue reinitialization incomplete")
flag = False
r=[]
#Stress test
for j in range(size):
n=random.randint(0,size)
r.append(n)
try:
queue.enqueue(n)
except:
if verbose:
print("Error: Queue push incomplete.")
flag = False
try:
int(len(queue))
except:
if verbose:
print("Error: Queue __len__ method not returning integer")
flag = False
if(len(queue) != size):
if verbose:
print("Error: Queue size should be " + str(size) + " but is " + str(len(queue)))
flag = False
if verbose:
if flag:
print("Queue size test complete")
else:
print("Queue size test failed")
yield flag
q=''
if flag:
for j in range(size):
try:
q=queue.dequeue()
except:
if verbose:
print("Error: Queue retrieval incomplete")
flag = False
if r[j] != q:
if verbose:
print("Error: Queue value should be " + str(r[j]) + " but is " + str(q) )
flag = False
if verbose:
if flag:
print("Queue value test complete")
else:
print("Queue value test failed")
yield flag
flag = True
try:
stack = lib.MyStack(3)
stack
except:
if verbose:
print("Error: Stack initialization incomplete")
flag = False
r = [3]
for j in range(size-1):
n=random.randint(0,size)
r.append(n)
try:
stack.push(n)
except:
if verbose:
print("Error: Stack push incomplete.")
flag = False
try:
int(len(stack))
except:
if verbose:
print("Error: Stack __len__ method not returning integer")
flag = False
if(len(stack) != size):
if verbose:
print("Error: Stack size should be " + str(size) + " but is " + str(len(stack)))
flag = False
if verbose:
if flag:
print("Stack size test complete")
else:
print("Stack size test failed")
yield flag
s=""
if flag:
for j in range(size):
try:
s=stack.pop()
except:
if verbose:
print("Error: Sack retrieval incomplete")
flag = False
if r[size-j-1] != s:
if verbose:
print("Error: Stack value should be " + str(r[size-j-1]) + " but is " + str(s) )
flag = False
#End stress test
if verbose:
if flag:
print("Stack value test complete")
else:
print("Stack value test failed")
yield flag
flag = True
# Check that queues are different objects, ensure non-loss of head node
m = random.randint(0,size)
n = random.randint(0,size)
o = random.randint(0,size)
queue2=None
try:
queue.enqueue(m)
queue2 = lib.MyQueue(data=n)
queue2.enqueue(o)
except:
if verbose:
print("Error: Queue operations incorrect")
flag = False
stack2=None
try:
stack.push(m)
stack2 = lib.MyStack(data=n)
stack2.push(o)
except:
if verbose:
print("Error: Stack operations incorrect")
flag = False
nQ = None
try:
nQ = queue2.dequeue()
nQ
except:
flag = False
if(nQ != n):
if verbose:
print(f"Error: Queue retrieval should be {n} but is {nQ}")
flag = False
oS = None
try:
oS = stack2.pop()
oS
oS = stack2.pop()
oS
except:
flag = False
if(oS != n):
if verbose:
print(f"Error: Stack retrieval should be {n} but is {oS}")
flag = False
if(queue.dequeue() != m):
if verbose:
print("Error: Queue instancing incomplete.")
flag = False
if(stack.pop() != m):
if verbose:
print("Error: Stack instancing incomplete.")
flag = False
if verbose:
if flag:
print("Object instantiation test complete")
else:
print("Object instantiation test failed")
yield flag
if __name__ == "__main__":
if len(sys.argv) < 2:
print("Include at least a library name as an argument.")
exit()
name = sys.argv[1]
if name.startswith(".\\"):
name = name[2:]
if name.endswith(".py"):
name = name[:-3]
module=import_module(name,package=__name__)
print(f"Testing module {name} by {module.getName()}")
score = 0
for i in Test(module,seed=77777, size=30000, rounds=10, verbose=True):
if i:
score+=1
print(f"Tests passed: {score}/5")
Step by Step Solution
There are 3 Steps involved in it
Step: 1
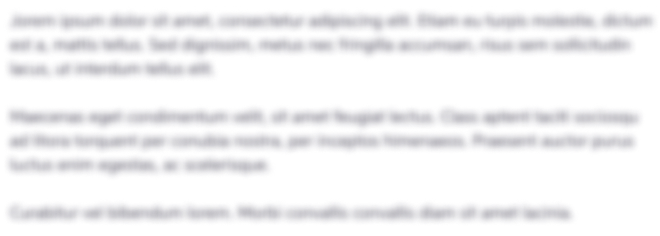
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started