Question
please update this code to carry out the correct implementation and work correctly with these files The program should do the following: read a NDFSM
please update this code to carry out the correct implementation and work correctly with these files
The program should do the following:
- read a NDFSM from the user specified file
- Convert to a DFSM
- repeat
ask the user to input a string which can be empty
output true if the string is accepted by the machine, false otherwise.
Until the user wants to stop
Format of the NDFSM file:
First row is alphabet separated by comma
Second row is the number of states of the NDFSM, implicitly the states are 0,1, 2.. where 0 is the initial state
Third row is the accepting states: one or more separated by comma.
The remaining rows are the transition relation, one row for each state. A transition is a triple (p, c, q), where p is the state, c is the alphabet, and q is the new state; for epsilon transition use (p, , q).
File example 1

a, b 3 1 (0, a, 1) (1, a, 1), (1, , 2) (2, b, 0)
File example 2

a, b 7 2, 6 (0, , 3), (0, , 4) (3, a, 3), (3, b, 3), (3, b, 1) (1, b, 2) (2, a, 2), (2, b, 2) (4, a, 4), (4, b, 4), (4, a, 5) (5, a, 6) (6, a, 6,), (6, b, 6)
This is the output that I'm getting for both files
File example 1 output
Converted DFSM: 0 : {a=1, } 1 : {a=1, } Enter a string (type 'exit' to stop): aab false Enter a string (type 'exit' to stop): abab false Enter a string (type 'exit' to stop):
File example 2 output
Converted DFSM: 0 : {=1, } 1 : {=-1, } Enter a string (type 'exit' to stop): aab false Enter a string (type 'exit' to stop): abab false Enter a string (type 'exit' to stop): ba false Enter a string (type 'exit' to stop):
import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.*;
public class NDFSMToDFSMConverter {
private static Set
public static void main(String[] args) { Scanner scanner = new Scanner(System.in);
// Read NDFSM from file readNDFSMFromFile("ex1.txt");
// Convert NDFSM to DFSM Map
// Query user for strings while (true) { System.out.print("Enter a string (type 'exit' to stop): "); String input = scanner.nextLine().trim();
if (input.equalsIgnoreCase("exit")) { break; }
boolean isAccepted = checkString(dfsm, input); System.out.println(isAccepted); }
scanner.close(); }
private static void readNDFSMFromFile(String filename) { ndfsm = new HashMap(); acceptingStates = new HashSet();
try (BufferedReader reader = new BufferedReader(new FileReader(filename))) { String alphabetLine = reader.readLine(); String[] alphabet = alphabetLine.split(", ");
int numStates = Integer.parseInt(reader.readLine());
String acceptingStatesLine = reader.readLine(); if (!acceptingStatesLine.isEmpty()) { String[] acceptingStatesArray = acceptingStatesLine.split(", "); for (String state : acceptingStatesArray) { acceptingStates.add(Integer.parseInt(state)); } }
for (int i = 0; i
ndfsm.computeIfAbsent(currentState, k -> new HashMap()); ndfsm.get(currentState).computeIfAbsent(symbol, k -> new HashSet()); ndfsm.get(currentState).get(symbol).add(nextState); } } catch (IOException e) { e.printStackTrace(); } }
private static Map
Set
Set
while (!queue.isEmpty()) { Set
powersetStates.computeIfAbsent(currentStateSet, k -> new HashMap());
for (String symbol : alphabet) { Set
powersetStates.get(currentStateSet).put(symbol, nextStateSet);
if (!powersetStates.containsKey(nextStateSet) && !nextStateSet.isEmpty()) { queue.offer(new HashSet(nextStateSet)); } } }
// Map the powerset states to unique integer IDs Map
// Build the final DFSM Map
// Print the converted DFSM for debugging purposes System.out.println("Converted DFSM:"); for (Map.Entry
return dfsm; }
private static boolean checkString(Map
Step by Step Solution
There are 3 Steps involved in it
Step: 1
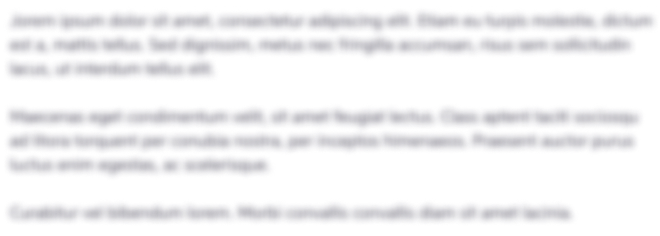
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started