Question
Please use c++ to solve this. -------------------The Problem We are going to work on making our own classes with private data members and accessors. We
Please use c++ to solve this.
-------------------The Problem
We are going to work on making our own classes with private data members and accessors. We are going to build a Table class, a 2D vector class.
-------------------Table class
The header for the Table class has the following private elements:
private: vector> t_; // 2D vector of long long width_; // how wide is t_ (how many columns) long height_; // how high is t_ (how many rows)
-------------------------------The methods are as follows:
1.Table(long width, long height, long val=0) constructor. Makes a Table that is rectangle shaped, width x height. Each element is set to val, which defaults to 0. Remember that t_ is a vector>and that what you can push_back onto t_ is a vector which constitutes a row of t_
2.void fill_random(long lo, long hi, unsigned int seed=0). Method to set every t_ element to a random number of long between lo and hi inclusive. seed sets the random number seed, defaults to 0.
3.bool set_value(unsigned int row_num, unsigned int col_num, long val). A method to set a particular element, indicated by row_num and col_num, to the provided val.
If row_num and col_num are indicies that exist in t_, sets that element to val and returns true.
Otherwise it does not set the element and then it returns false.
4.long get_value (unsigned int row_num, unsigned int col_num) const. Method to provide the value at (row_num, col_num) of t_ if those two indicies exist.
If the two indicies are valid, return the t_ element.
If not, well we have a decision to make. Any long we return might actually be a legit long in the table, even though our intention was to indicate "not there" somehow. So we choose something weird. There is a special function that prints out the smallest long possible on the machine. Let's do that. It would look something like:
#include
// ... do your thing ...
return std::numeric_limits::lowest();
5. ostream& operator<<(ostream& out, Table t) a friend function to print the contents of t_ in a "nice way" (as a square with rows and columns) to the provided stream out . Must be declared a friend in the class, then defined as a function.]
-----------------------------Here is the Assignment
You are provided with table.h and main-table.cpp(i have posted down below). Create "table.cpp". Results should look like the below.
0,0,0,0,0, 0,0,0,0,0, 0,0,0,0,0, 0,0,0,0,0, 0,0,0,0,0, 2,10,1,6,6, 1,7,5,9,10, 5,7,10,4,3, 5,8,7,6,3, 2,6,6,2,2, Result:false 6 -9223372036854775808 100,10,1,6,6, 1,100,5,9,10, 5,7,100,4,3, 5,8,7,100,3, 2,6,6,2,100,
-------------------------------------Here is the table.h
#ifndef TABLE_CLASS #define TABLE_CLASS #include using std::vector; #include using std::cout; using std::endl; using std::ostream; class Table{ private: vector> t_; // 2D vector of long long width_; // how wide is t_ (how many columns) long height_; // how high is t_ (how many rows) public: // table will be width x height, default val is 0 Table(long width, long height, long val=0); // range from lo to hi, seed has default void fill_random(long lo, long hi, unsigned int seed=0); bool set_value(unsigned int row_num, unsigned int col_num, long val); long get_value (unsigned int row_num, unsigned int col_num) const; void print_table(ostream&); friend ostream& operator<<(ostream&, Table); }; ostream& operator<<(ostream&, Table); #endif
---------------------------Here is the main-table.cpp
#include using std::cout; using std::endl; using std::boolalpha; #include "table.h" int main (){ bool result_bool; long result_long; cout << boolalpha; Table my_table(5,5); cout << my_table << endl;; my_table.fill_random(1,10); cout << my_table << endl; result_bool = my_table.set_value(100,100,100); cout << "Result:"<
Step by Step Solution
There are 3 Steps involved in it
Step: 1
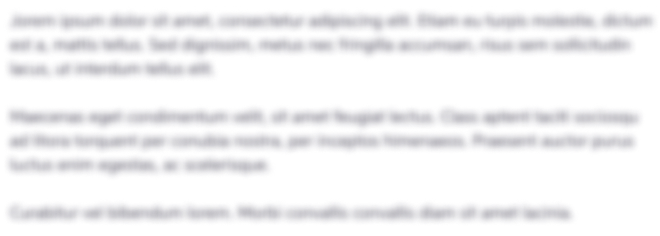
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started