Question
Please use Chain of Responsibility pattern for the corresponding code using this reference https://sourcemaking.com/design_patterns/chain_of_responsibility class LoanApprover { protected LoanApprover nextApprover ; public void SetNextApprover (
Please use Chain of Responsibility pattern for the corresponding code using this reference https://sourcemaking.com/design_patterns/chain_of_responsibility
class LoanApprover
{
protected LoanApprover nextApprover;
public void SetNextApprover(LoanApprover nextApprover)
{
this.nextApprover = nextApprover;
}
public virtual void ApproveLoan(Loan i);
}
class Manager : public LoanApprover
{
public void ApproveLoan(Loan i)
{
if (i.Amount <= 100000)
cout << "Loan amount of " << i.Amount << " approved by the Manager") << endl;
else
nextApprover.ApproveLoan(i);
}
}
class Director : public LoanApprover
{
public void ApproveLoan(Loan i)
{
if (i.Amount <= 250000)
cout << "Loan amount of " << i.Amount << " approved by the Director") << endl;
else
nextApprover.ApproveLoan(i);
}
}
class VicePresident : LoanApprover
{
public void ApproveLoan(Loan i)
{
cout << "Loan amount of " << i.Amount << " approved by the Vice President") << endl;
}
}
public class Loan
{
private int amount;
public int Amount
{
return amount;
}
public Loan(int amount)
{
this.amount = amount;
}
}
void main(int argc, char* argv[])
{
LoanApprover a = new Manager();
LoanApprover b = new Director();
LoanApprover c = new VicePresident();
a.SetNextApprover(b);
b.SetNextApprover(c);
a.ApproveLoan(new Loan(50000)); //this will be approved by the manager
a.ApproveLoan(new Loan(200000)); //this will be approved by the director
a.ApproveLoan(new Loan(500000)); //this will be approved by the vice president
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
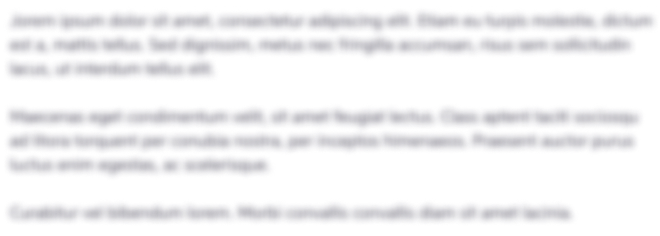
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started