Question
Please use functions to abstract the code below. The goal of this is to make the code more readable and organized. The new code should
Please use functions to abstract the code below. The goal of this is to make the code more readable and organized. The new code should run the exact same way as the code below.
Execution, readability and cleanliness are important.
Once you have completed this, please write approx 1-2 pages explaning
your technique of abstracting the code. Please discuss your decisions, your process, your
struggles, etc.
Please do not submit a handwritten response as this is not legible in most cases.
Thank you.
from random import randint
def deck_of_cards():
face_val = ['Ace','2','3','4','5','6','7','8','9','10','J','Q','K']
cards_val = ['Hearts','Spades','Clubs','Diamonds']
decks = []
for x in cards_val:
for y in face_val:
decks.append(y + ' of ' + x)
return decks
def face_val(card):
if card[:1] in ('J','Q','K','1'):
return int(10)
elif card[:1] in ('2','3','4','5','6','7','8','9'):
return int(card[:1])
elif card[:1] == 'A':
print (" "+ str(card))
num = input("Do you want this to be 1 or 11? >")
while num !='1' or num !='11':
if num == '1':
return int(1)
elif num == '11':
return int(11)
else:
num = input("Do you want this to be 1 or 11? >")
def new_cards(decks):
return decks[randint(0,len(decks)-1)]
def removing_cards(decks,card):
return decks.remove(card)
restart = ''
while restart != 'EXIT':
new_deck = deck_of_cards()
crd = new_cards(new_deck)
removing_cards(new_deck,crd)
crd1 = new_cards(new_deck)
removing_cards(new_deck,crd1)
print (" " + crd + " and " + crd1) #doing this statement first allows for selection between 1 and 11
val1 = face_val(crd)
val2 = face_val(crd1)
tot = val1 + val2
print("with a tot of " + str(tot) )
deal_cards1 = new_cards(new_deck)
removing_cards(new_deck,deal_cards1)
deal_cards2 = new_cards(new_deck)
removing_cards(new_deck,deal_cards2)
deal_val1 = face_val(deal_cards1)
deal_val2 = face_val(deal_cards1)
deal_tot = deal_val1 + deal_val2
print (' The Dealer looks at you and deals one card up and one card face down')
print ("First a " + deal_cards1 + " and face down card.")
if tot == 21:
print("Blackjack!")
else:
while tot < 21: #not win or loss yet
choice = input("do you want to hit or stand? > ")
if choice.lower() == 'hit':
cards_extra = new_cards(new_deck)
removing_cards(new_deck,cards_extra)
more_value = face_val(cards_extra)
tot += int(more_value)
print (cards_extra + " for a new tot of " + str(tot))
if tot > 21: #lose condition
print("You LOSE!")
restart = input("Continue? EXIT to leave ")
elif tot == 21: #winning condition
print("You WIN WIN!")
restart = input("Continue? EXIT to leave ")
else:
continue
elif choice.lower() == 'stand':
print("The dealer reveals his other card to be ")
print("a " + deal_cards2 + " for a tot of " + str(deal_tot))
if deal_tot < 17:
print("The Dealer hits again.")
dealer_more = new_cards(new_deck)
more_dealer_value = face_val(dealer_more)
print("The card is a " + str(dealer_more))
deal_tot += int(more_dealer_value)
if deal_tot > 21 and tot <=21:
print("Dealer Bust! You win!")
elif deal_tot < 21 and deal_tot > tot:
print("Dealer has " + str(deal_tot) + " You lose!")
else:
continue
elif deal_tot == tot:
print("Equal Deals, no winner")
elif deal_tot < tot:
print("You win!")
else:
print("You Lose!")
restart = input(" Would you like to continue? EXIT to leave ")
break
print("Thank you for Playing")
#Please write answer in computer text. Not hand-written.
#Thank you.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
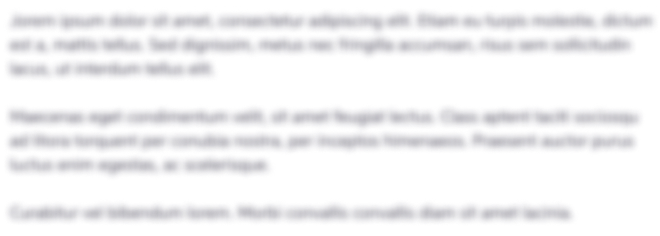
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started