Question
PLEASE USE JAVA WHILE CODING THIS PROGRAM Overview For this project, you will be looking at a competition of your choosing (e.g., the NHL sea-
PLEASE USE JAVA WHILE CODING THIS PROGRAM
Overview
For this project, you will be looking at a competition of your choosing (e.g., the NHL sea- son/playoffs), and analyzing the statistics of the competitors to see what attributes are shared
by successful competitors. Consider the 2015-2016 NHL season. You could write a program that would look at (for each NHL team), the average number of points scored in a game, the average number of points scored by opponents in a game, the average number of shots taken per game, the average number of shots taken by opponents per game, and the percentage of penalties killed throughout the season. You program would then compute the average, min, and max of each of these stats across all teams in the NHL, the average, min, and max of teams that made the playoffs, and the average, min, and max of teams that did not make the playoffs. Are all of these statistics important to a winning team? Is there one that stands out as prevalant amongst winning teams? Is there one that seems to indicate that a team will not make the playoffs?
1
1 Gathering data The first part of this project will be to pick a competition to analyze and gather data. You must pick a competition where at least 8 competitors/teams will be considered winners (e.g., make the playoffs), and at least 8 will not. Then, you must pick 5 statistics for each competitor/team (e.g., goals scored per game, goals scored against per game, shots taken per game, shots taken against per game, % of penalties killed) and gather the necessary data into a single text file. All of your statistics should be of type double. Once you have selected a competition and statistics to track, you should construct a text file named data.txt that will store all of your data. This file should be formatted such that there is one value on each line. E.g., Name1 (String) Stat1a (double) Stat1b (double) Stat1c (double) Stat1d (double) Stat1e (double) Winner? (boolean) Name2 (String) Stat2a (double) Stat2b (double) Stat2c (double) Stat2d (double) Stat2e (double) Winner? (boolean) ... For example, a file for the 2015-2016 NHL season tracking the stats listed above could result in a data.txt that looks like this: Pittsburgh Penguins 2.94 2.43 33.2 29.7 84.4 true New Jersey Devils 2.22 2.46 24.4 28.6 83.0 false ...
2
2 Basic analysis For the second part of the project, you should write a program that will read your data.txt and print out the min, max, and average for each of your chosen statistics. Note that you will need to be very careful about reading through your file to ensure that you read all of your data correctly. An example run for the 2015-2016 NHL season would look like this: PPG PAPG SHOTPG SHOTAPG PK MIN 2.22 2.29 24.40 27.30 75.50 MAX 3.23 3.13 33.20 32.80 87.20 AVG 2.67 2.67 29.74 29.73 81.32
3
3 Utilizing arrays and methods For the third part of this project, you will have to redo your previous solution to split it up into multiple parts. Specifically, you will be writing 3 methods to aid in your analysis: double[][] readFile(String filename) This function should be used to read data.txt and produce a 2D array containing all of the stats for each team or competitor. Each row of this 2D array should contain the stats for a single team, hence it will be an n 5 array where n is the number of teams/competitors. One row for each team/competitor, and one column for each stat. For the NHL example, the 2D array would look like this:
2.94 2.43 33.2 29.7 84.4 2.22 2.46 24.4 28.6 83.0
...
double[][] readFile(String filename, boolean winner) This function presents an example of overloading. It should operate in a very similar way to the first readFile function, however, if winner is set to true, it should return a 2D array containing only the stats of teams/competitors that are considered winners (e.g., NHL teams that made the playoffs). If set to false, it should return a 2D array of only teams/competitors who are not considered winners (e.g., NHL teams that did not make the playoffs). void analyze(double[][] data) This function should take in a 2D array of statistics and print out the min, max, and average of each column of the array (i.e., of each statistic). Bringing the methods together Once you have finished writing each of these three methods, your program should operate as follows: Call double[][] readFile(String filename) to get the statistics on all teams/competitors. Call void analyze(double[][] data) on the resulting 2D array to display the min, max, and average across all teams/competitors. Call double[][] readFile(String filename, boolean winner) with winner set to true to get the statistics on all winners. Call void analyze(double[][] data) on the resulting 2D array to display the min, max, and average across all winners. Call double[][] readFile(String filename, boolean winner) with winner set to false to get the statistics on all non-winners. Call void analyze(double[][] data) on the resulting 2D array to display the min, max, and average across all non-winners. 4
4 Notes and hints Note that this assignment will require you to carefully keep track of the ordering of your statistics (e.g., points scored per game is the first stat after the team name in data.txt and will appear in column 0 of any 2D array). Since double[][] readFile(String filename, boolean winner), is more detailed than double[][] readFile(String filename), it is recommended to get double[][] readFile(String filename) working first before working on double[][] readFile(String filename, booean winner).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
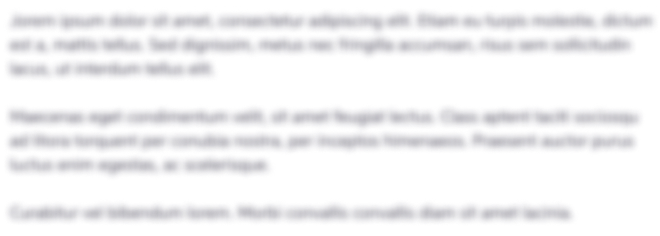
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started