Question
Please use the code below and edit/change it to make it follow these criterias: 1) In Lab 2, we assume the key provided by user
Please use the code below and edit/change it to make it follow these criterias:
1) In Lab 2, we assume the key provided by user in a command-line argument only consists of upper-case letters. However, in this Project 1, a correct key can include both upper-case and lower-case letters, such as foRk. In that case, the numbers of shifts are still 5, 14, 17, and 10 for the key foRk, because for a lower-case letter in the key, the number of shifts for encryption is its relative distance to the lower-case letter a. In other words, when the program meets a lower-case letter in the key, we use the lower-case letter a to calculate the number of shifts. (Of course, we use A to calculate that for an upper-case letter in the key.) Hence, the encrypted texts for the key foRk are exactly the same as the encrypted texts for the key FORK.
2) In Lab 2, we assume the key always contains four letters. On the contrary, in this Project 1, the key length can be flexible.
To simplify the problem, we can also have the following assumptions and requirements in this project.
1) A correct key only contains letters to encrypt and decrypt a file. However, it is possible that the user provides a wrong key that contains characters other than letters (e.g., abcd123efg). If that happens, your program should print a message Wrong key! The key can only contain letters! and exit without further encryption/decryption.
2) The original input text file can have both upper-case and lower-case letters, along with numbers and other special characters.
3) The key can contain duplicate letters.
4) Encrypting and decrypting are only applied to letters. Numbers and other special symbols in the text will be kept the same in encryption and decryption.
5) Upper-case letters will be encrypted/decrypted as upper-case letters, and lower-case letters will be encrypted/decrypted as lower-case letters.
My Code From Lab 2:
#include#include #include #include char encrypt_decrypt(char ch, int k); void setKeys(char* word, int* keys, int choice); int main(int argc, char* argv[]) { int choice = 0, index, key[4]; char ch; FILE *fin, *fout; if (argc != 5) { printf ("Usage: cipher inputFile key outputFile option(e or d) "); printf ("Option e for encryption and d for decryption"); exit(1); } if (strcmp(argv[4],"d")==0) choice = 1; setKeys(argv[2], key, choice); fin = fopen(argv[1], "r"); fout = fopen(argv[3], "w"); if (fin == NULL || fout == NULL) { printf("File could not be opened! "); exit(1); } index = 0;//initialize the index to 0 while ( fscanf(fin, "%c", &ch) != EOF ) { fprintf(fout, "%c", encrypt_decrypt(ch, key[index])); index = (index + 1) % 4; } fclose(fin); fclose(fout); return 0; } char encrypt_decrypt(char ch, int k) { if ( k The code should be almost similar but with modifications following the criteria.
In this project, you will need to implement a C program for encryption and decryption based on what you have learned and achieved in Lab 1 and Lab 2. Please revisit the requirements of Lab 1 and Lab 2 first. You can start from the C source file of your Lab 2 solution. For your convenience, the idea of how Lab 2 works is copied and pasted below. Start of copy and paste from Lab 2 Caesar ciphers are a basic encryption technique in which each letter in the original text is replaced by a letter some fixed number of positions down the alphabet. Here, the fixed number of positions is referred to as the key for the cipher. For example, if the key is 4 , then the mapping between the original and encrypted alphabets will be as follows: Caesar ciphers can be automatically broken by simply trying out all the 25 keys. A simple yet effective way of improvement is to use several numbers, instead of one, as the key; that is, using different shifts at different positions in the original text. For example, we could shift the first character by 5 , the second by 14 , the third by 17 , and the fourth by 10 . Then we repeat the pattern, shifting the fifth character by 5 , the sixth by 14 , and so on, until we run out of characters in the text. Obviously, the more numbers the key has, the more difficult to break the encryption. In the above example, the four numbers are 5,14,17, and 10 , which are the numerical equivalents of the string "FORK" in a 26-letter alphabet consisting of the letters A-Z. (We can see that ' F ', ' O ', ' R ' and ' K ' are 5th, 14th,17th, and 10th letters respectively in the alphabet, if the index starts at 0. .) In this lab, you will modify a Caesar cipher program "cipher.c" (on the BlackBoard) which only uses one number as its key, to encrypt and decrypt a text file. Your modified program should accept the key consisting of four characters, like "FORK", as a command-line argument. End of copy and paste from Lab 2 - The idea and result of this project are very similar to Lab 2. In other words, in this project, we still use a key consisting of letters to indicate the numerical shifts of texts in input files for encryption and decryption, as in Lab 2. However, this project must support additional requirements as follows. 1) In Lab 2, we assume the key provided by user in a command-line argument only consists of upper-case letters. However, in this Project 1, a correct key can include both upper-case and lower-case letters, such as "foRk". In that case, the numbers of shifts are still 5, 14, 17, and 10 for the key "foRk", because for a lower-case letter in the key, the number of shifts for encryption is its relative distance to the lower-case letter ' a '. In other words, when the program meets a lower-case letter in the key, we use the lower-case letter ' a ' to calculate the number of shifts. (Of course, we use 'A' to calculate that for an upper-case letter in the key.) Hence, the encrypted texts for the key "foRk" are exactly the same as the encrypted texts for the key "FORK". 2) In Lab 2, we assume the key always contains four letters. On the contrary, in this Project 1, the key length can be flexible. To simplify the problem, we can also have the following assumptions and requirements in this project. 1) A correct key only contains letters to encrypt and decrypt a file. However, it is possible that the user provides a wrong key that contains characters other than letters (e.g., "abcd123efg"). If that happens, your program should print a message "Wrong key! The key can only contain letters!" and exit without further encryption/decryption. 2) The original input text file can have both upper-case and lower-case letters, along with numbers and other special characters. 3) The key can contain duplicate letters. 4) Encrypting and decrypting are only applied to letters. Numbers and other special symbols in the text will be kept the same in encryption and decryption. 5) Upper-case letters will be encrypted/decrypted as upper-case letters, and lower-case letters will be encrypted/decrypted as lower-case letters. Same as Lab 2, your program should provide the user with an option that is encryption or decryption. It should also allow the user to specify the key as well as the input and output filenames. Use command line arguments for the user to pass them into your program. When you test your program, you need to run it to generate an encrypted file from an original text file, run your program again with the encrypted file as the input to produce a decrypted file, and then compare the decrypted file to the original text file and see if they are the same. Usage: (Please follow this usage and make sure your program supports the same format for command line input) When you finish programming, you can use the following command in Linux environment to compile. gcc YourSourceFile.c -o project1 Then you should use keyboard input to provide the original text file name, the key, the output (encrypted) text file name, and the choice of encryption (e) or decryption (d). (Please follow this example to provide command line input.) For example, ./project1 original.txt ComPuTer encrypted.txt e After this command, your program should encrypt the original text in "original.txt" using the key "ComPuTer", and write the encrypted text to the file "encrypted.txt". Then you can use ./project1 encrypted.txt ComPuTer decrypted.txt d to decrypt the encrypted text file "encrypted.txt" using the key "ComPuTer", and write the decrypted text to the file "decrypted.txt". If your program works correctly, the texts in files "original.txt" and "decrypted.txt" should be identical
Step by Step Solution
There are 3 Steps involved in it
Step: 1
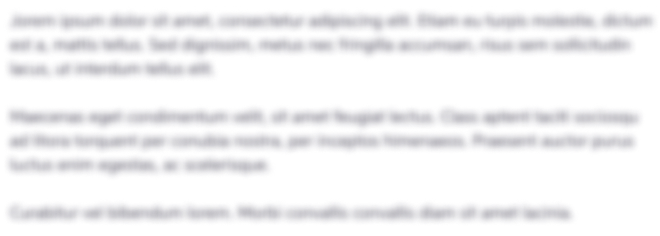
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started