Question
Please use the code below and make it operate as one program. Notating what you did to make it work would also be greatly appreciated.
Please use the code below and make it operate as one program. Notating what you did to make it work would also be greatly appreciated.
#include
using namespace std;
void getValidUserinputPosNumGTO(int& userInput) { do { cout << "Please enter a positive integer greater than zero: "; cin >> userInput; } while (userInput <= 0); }
int main() { int userInput; getValidUserinputPosNumGTO(userInput); cout << "The user entered: " << userInput << endl; return 0; }
#include
int CountOfDivisors(int num) { int count = 0; for (int i = 1; i <= num; i++) { if (num % i == 0) { count++; } } return count; }
int main() { int num = 24; int count = CountOfDivisors(num); std::cout << "The number " << num << " has " << count << " divisors." << std::endl; return 0; }
#include
bool IsSquare(int num) { int count = 0; for (int i = 1; i <= num; i++) { if (num % i == 0) { count++; } } return (count % 2 != 0); }
int main() { int num = 25; bool isSquare = IsSquare(num); if (isSquare) { std::cout << num << " is a square number." << std::endl; } else { std::cout << num << " is not a square number." << std::endl; } return 0; }
#include
bool IsPrime(int num); // Declaration of the IsPrime function
void DisplayPrime(int num) { if (IsPrime(num)) { std::cout << num << " is a prime number." << std::endl; } else { std::cout << num << " is not a prime number." << std::endl; } }
int main() { int num = 17; DisplayPrime(num); return 0; }
bool IsPrime(int num) // Definition of the IsPrime function { if (num <= 1) { return false; } for (int i = 2; i <= num / 2; i++) { if (num % i == 0) { return false; } } return true; }
#include
bool IsPrime(int num);
int main() { int num = 17; if (IsPrime(num)) { std::cout << num << " is a prime number." << std::endl; } else { std::cout << num << " is not a prime number." << std::endl; } return 0; }
bool IsPrime(int num) { if (num <= 1) { return false; } for (int i = 2; i <= num / 2; i++) { if (num % i == 0) { return false; } } return true; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
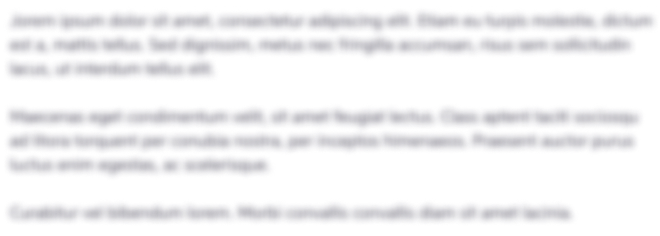
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started