Question
Please use the code provided in Python and use the following set of instructions. This will not work unless it is done in Python. Matrix
Please use the code provided in Python and use the following set of instructions. This will not work unless it is done in Python.
Matrix Code:
# Write your code below:
def main():
m1 = Matrix(3, 3, 2)
m2 = Matrix(3, 3, 4)
print(m1 + m2)
m1 = Matrix(4, 4, 2)
m2 = Matrix(4, 4, 4)
print(m2 - m1)
m1 = Matrix(2, 3, 2)
m2 = Matrix(3, 2, 4)
print(m1 * m2)
if __name__ == "__main__": main()
Arrays Code:
class Array(object):
"""Represents an array."""
def __init__(self, capacity, fillValue = None):
"""Capacity is the static size of the array.
fillValue is placed at each position."""
self.items = list()
for count in range(capacity):
self.items.append(fillValue)
def __len__(self):
"""-> The capacity of the array."""
return len(self.items)
def __str__(self):
"""-> The string representation of the array."""
return str(self.items)
def __iter__(self):
"""Supports traversal with a for loop."""
return iter(self.items)
def __getitem__(self, index):
"""Subscript operator for access at index."""
return self.items[index]
def __setitem__(self, index, newItem):
"""Subscript operator for replacement at index."""
self.items[index] = newItem
Grid Code:
from arrays import Array
class Grid(object):
"""Represents a two-dimensional array."""
def __init__(self, rows, columns, fillValue = None):
self.data = Array(rows)
for row in range(rows):
self.data[row] = Array(columns, fillValue)
def getHeight(self):
"""Returns the number of rows."""
return len(self.data)
def getWidth(self):
"Returns the number of columns."""
return len(self.data[0])
def __getitem__(self, index):
"""Supports two-dimensional indexing with [][]."""
return self.data[index]
def __str__(self):
"""Returns a string representation of the grid."""
result = ""
for row in range(self.getHeight()):
for col in range(self.getWidth()):
result += str(self.data[row][col]) + " "
result += " "
return result
def main():
g = Grid(10, 10, 1)
print(g)
if __name__ == "__main__":
main()
A Matrix class can be used to perform some of the operations found in linear algebra, such as matrix arithmetic. Develop a Matrix class that uses the built-in operators for arithmetic. The Matrix class should extend the Grid class. If two matrices cannot be multiplied, raise an exception . A main() has been provided to test the implementation of Matrix
Step by Step Solution
There are 3 Steps involved in it
Step: 1
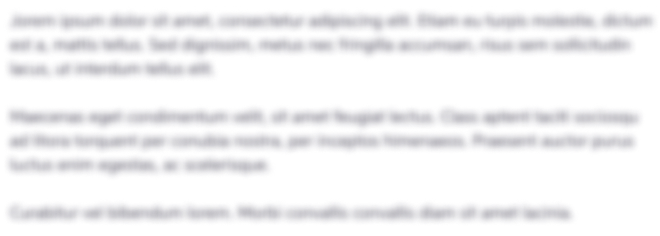
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started