Question
Please use this template below: #include using namespace std; // Rational Class declaration class Rational { private : int numerator; int denominator; public : Rational();
Please use this template below:
#include
using namespace std;
// Rational Class declaration
class Rational {
private:
int numerator;
int denominator;
public:
Rational();
explicit Rational(int);
Rational(int, int);
const Rational add(const Rational &) const;
const Rational subtract(const Rational &) const;
const Rational multiply(const Rational &) const;
const Rational divide(const Rational &) const;
void simplify();
void display() const;
private:
int gcd(int, int) const;
};
// Implement Rational class member functions here
// Do not change any of the code below this line!!
Rational getRational();
void displayResult(const string &, const Rational &, const Rational&, const Rational&);
int main() {
Rational A, B, result;
char choice;
cout
A = getRational();
cout
cout
B = getRational();
cout
cout
cin >> choice;
cout
if (choice == 'a') {
result = A.add(B);
displayResult("+", A, B, result);
} else if (choice == 's') {
result = A.subtract(B);
displayResult("-", A, B, result);
} else if (choice == 'm') {
result = A.multiply(B);
displayResult("*", A, B, result);
} else if (choice == 'd') {
result = A.divide(B);
displayResult("/", A, B, result);
} else if (choice == 'y') {
A.simplify();
A.display();
} else {
cout
}
cout
return 0;
}
Rational getRational() {
int choice;
int numer, denom;
cout
cin >> choice;
cout
if (choice == 2) {
cout
cin >> numer;
cout
cout
cin >> denom;
cout
return Rational(numer, denom);
} else if (choice == 1) {
cout
cin >> numer;
cout
return Rational(numer);
} else {
return Rational();
}
}
void displayResult(const string &op, const Rational &lhs, const Rational&rhs, const Rational &result) {
cout
lhs.display();
cout
rhs.display();
cout
result.display();
cout
}
Rational Numbers It may come as a bit of a surprise when the C++ floating-point types (float, double), fail to capture a particular value accurately. Certainly double, which is usually stored as a 64-bit value, is far better than the old float, which is only 32 bits, but problems do arise. For example: float n = 2.0; float d = 3.0; cout
Step by Step Solution
There are 3 Steps involved in it
Step: 1
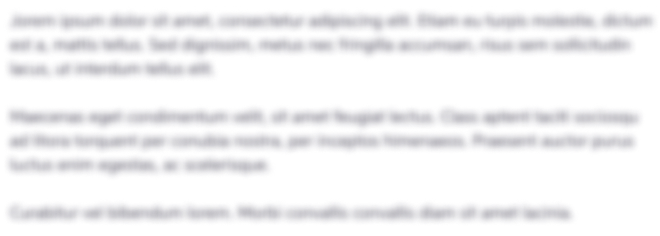
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started