Question
Please who can help with this question in Java thank you 1. Reading the following codes carefully. Make any corrections if needed and write out
Please who can help with this question in Java thank you
1. Reading the following codes carefully. Make any corrections if needed and
write out the output.
/*
The Java programming language allows you to define a class within another class.
Such a class is called a nested class and is illustrated here:
class OuterClass {
...
(private) class NestedClass {
...
}
}
--------------------------------------------------------------------------------
Terminology: Nested classes are divided into two categories: static and non-static.
Nested classes that are declared static are simply called static nested classes.
Non-static nested classes are called inner classes.
--------------------------------------------------------------------------------
class OuterClass {
...
static class StaticNestedClass {
...
}
(private) class InnerClass {
...
}
}
A nested class is a member of its enclosing class. Non-static nested classes (inner classes)
have access to other members of the enclosing class, even if they are declared private.
Static nested classes do not have access to other members of the enclosing class.
As a member of the OuterClass, a nested class can be declared private, public,
protected, or package private. (Recall that outer classes can only be declared public
or package private.)
Why Use Nested Classes?
There are several compelling reasons for using nested classes, among them:
It is a way of logically grouping classes that are only used in one place.
It increases encapsulation.
Nested classes can lead to more readable and maintainable code.
Logical grouping of classes - If a class is useful to only one other class,
then it is logical to embed it in that class and keep the two together.
Nesting such "helper classes" makes their package more streamlined.
Increased encapsulation - Consider two top-level classes, A and B, where B
needs access to members of A that would otherwise be declared private.
By hiding class B within class A, A's members can be declared private
and B can access them. In addition, B itself can be hidden from the outside world.
More readable, maintainable code - Nesting small classes within
top-level classes places the code closer to where it is used.
Static Nested Classes
As with class methods and variables, a static nested class is
associated with its outer class. And like static class methods,
a static nested class cannot refer directly to instance variables or
methods defined in its enclosing class - it can use them only through an object reference.
To see an inner class in use, let's first consider an array.
In the following example, we will create an array, fill it with
integer values and then output only values of even indices of
the array in ascending order.
The DataStructure class below consists of:
The DataStructure outer class, which includes methods to add
an integer onto the array and print out values of even indices
of the array.
The InnerEvenIterator inner class, which is similar to a standard
Java iterator. Iterators are used to step through a data structure
and typically have methods to test for the last element, retrieve the
current element, and move to the next element.
A main method that instantiates a DataStructure object (ds) and uses
it to fill the arrayOfInts array with integer values (0, 1, 2, 3, etc.),
then calls a printEven method to print out values of even indices of arrayOfInts. */
public class DataStructure {
//create an array
private final static int SIZE = 15;
private int[] arrayOfInts = new int[SIZE];
public DataStructure() {
//fill the array with ascending integer values
for (int i = 0; i < SIZE; i++) {
arrayOfInts[i] = i;
}
}
public void printEven() {
//print out values of even indices of the array
InnerEvenIterator iterator = this.new InnerEvenIterator();
while (iterator.hasNext()) {
System.out.println(iterator.getNext() + " ");
}
}
//inner class implements the Iterator pattern
private class InnerEvenIterator {
//start stepping through the array from the beginning
private int next = 0;
public boolean hasNext() {
//check if a current element is the last in the array
return (next <= SIZE - 1);
}
public int getNext() {
//record a value of an even index of the array
int retValue = arrayOfInts[next];
//get the next even element
next += 2;
return retValue;
}
}
public static void main(String s[]) {
//fill the array with integer values and print out only values of even indices
DataStructure ds = new DataStructure();
ds.printEven();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
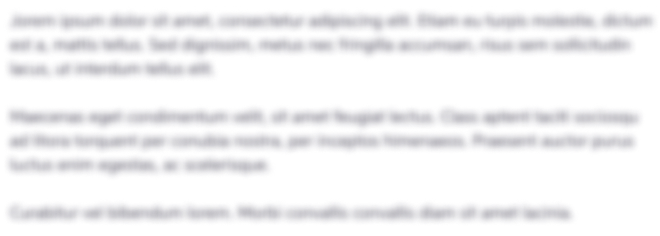
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started