Question
Please write generic code for binary heap sort. Using only the provided public methods (you may add private/protected methods) import java.util.Iterator; public interface PriorityQueue> extends
Please write generic code for binary heap sort. Using only the provided public methods (you may add private/protected methods)
import java.util.Iterator;
public interface PriorityQueue> extends Iterable { public static final int DEFAULT_MAX_CAPACITY = 1000;
// Inserts a new object into the priority queue. Returns true if the insertion is successful. If the PQ is full, the insertion is aborted, and the method returns false. public boolean insert(E object);
// Removes the object of highest priority that has been in the // PQ the longest, and returns it. Returns null if the PQ is empty.
public E remove();
// Deletes all instances of the parameter obj from the PQ if found, and // returns true. Returns false if no match to the parameter obj is found.
public boolean delete(E obj);
// Returns the object of highest priority that has been in the PQ the longest, but does NOT remove it. // Returns null if the PQ is empty. public E peek();
// Returns true if the priority queue contains the specified element false otherwise. public boolean contains(E obj);
// Returns the number of objects currently in the PQ. public int size();
// Returns the PQ to an empty state. public void clear();
// Returns true if the PQ is empty, otherwise false
public boolean isEmpty();
// Returns true if the PQ is full, otherwise false. List based implementations should always return false. public boolean isFull();
// Returns an iterator of the objects in the PQ, in no particular order. public Iterator iterator();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
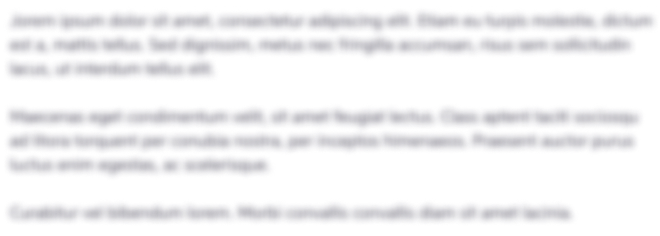
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started