Question
Please write in assembly language. Need procedure for option 2, option 3, and option 6 Description: This program presents a menu allowing the user to
Please write in assembly language. Need procedure for option 2, option 3, and option 6
Description: This program presents a menu allowing the user to pick a menu option
; which then performs a given task.
; If the user has not entered a string, options 2 through 5 can not be
; accomplished and the user should be prompted to enter a string.
; The palidrome check should not be case-sensitive or care about punctuation,
; to include spaces.The user will be allowed to enter a string containing any
; symbol or number on the standard QWERTY keyboard.
; When option 5 is selected, print the existing string(modified by other options or not)
; Option 4 prints the string and informs the user if the string is a palindrome or not.
; With options 2 - 3, print the string received by the option, and
; then the resulting string.
; The max string length must be a symbolic constant and used where necessary.
; Option Definitions
; 1. The user enters a string of no more than 100 characters.
; 2. The entered string is converted to lower case.
; 3. The entered string has all non - letter elements removed except numbers.
; 4. Is the entered string a palindrome?
; 5. Print the string.
; 6. Exit
; ====================================================================================
;// Your assignment is to implement all remaining functionality and error checking as required.
;// Remember this code allows the user to keep entering strings until
Include Irvine32.inc
;//Macros
ClearEAX textequ
ClearEBX textequ
ClearECX textequ
ClearEDX textequ
ClearESI textequ
ClearEDI textequ
Newline textequ <0ah, 0dh>
maxLength = 101d
.data
UserOption byte 0h
theString byte maxLength dup(0) ; // declares the array to be used throughout the program.
theStringLen byte 0
errormessage byte 'You have entered an invalid option. Please try again.', Newline, 0h
.code
main PROC
call ClearRegisters ;// clears registers
startHere:
mov ebx, OFFSET UserOption
call displayMenu
mov edx, offset theString
mov ecx, lengthof theString
opt1:
cmp useroption, 1
jne opt2
call clrscr
mov ebx, offset thestringlen ;// will hold the length of the entered string
call option1
jmp starthere
opt2:
cmp useroption, 2
jne opt3
call clrscr
movzx ecx, thestringlen
call option2
jmp starthere
opt3:
;// awaiting development
opt4:
;// awaiting development
opt5:
cmp useroption, 5
jne opt6
call clrscr
call option5
call waitmsg
jmp starthere
opt6:
cmp useroption, 6
jne oops ;// invalid entry
jmp quitit
;// error check
oops:
push edx
mov edx, offset errormessage
call writestring
call waitmsg
pop edx
jmp starthere
quitit:
exit
main ENDP
;// Procedures
;// ===============================================================
DisplayMenu Proc
;// Description: Displays the Main Menu to the screen and gets user input
;// Receives: Offset of UserOption variable in ebx
;// Returns: User input will be saved to UserOption variable
.data
MainMenu byte 'MAIN MENU', 0Ah, 0Dh,
'==========', 0Ah, 0Dh,
'1. Enter a String:', 0Ah, 0Dh,
'2. Convert all elements to lower case: ',0Ah, 0Dh,
'3. Remove all non-letter elements: ',0Ah, 0Dh,
'4. Determine if the string is a palindrome: ',0Ah, 0Dh,
'5. Display the string: ',0Ah, 0Dh,
'6. Exit: ',0Ah, 0Dh, 0Ah, 0Dh,
'Please enter a number between 1 and 6 -->', 0h
.code
push edx ;// preserves current value of edx
call clrscr
mov edx, offset MainMenu ;// required by WriteString
call WriteString
call readhex ;// get user input
mov byte ptr [ebx], al ;// save user input to UserOption
pop edx ;// restores current value of edx
ret
DisplayMenu ENDP
ClearRegisters Proc
;// Description: Clears the registers EAX, EBX, ECX, EDX, ESI, EDI
;// Requires: Nothing
;// Returns: Nothing, but all registers will be cleared.
cleareax
clearebx
clearecx
clearedx
clearesi
clearedi
ret
ClearRegisters ENDP
;// ---------------------------------------------------------------
option1 proc uses edx ecx
;// Description: Gets string from user.
;// Receives: Address of string
;// Returns: String is modified and length of entered string is in saved in theStringLen
.data
option1prompt byte 'Please enter a string of characters (', maxLength, ' or less): ', newline, '---> ', 0h
.code
push edx ;//saving the address of the string pass in.
mov edx, offset option1prompt
call writestring
pop edx
;//add procedure to clear string (loop through and place zeros)
call readstring
mov byte ptr [ebx], al ;//length of user entered string, now in thestringlen
ret
option1 endp
option2 proc uses edx
;// Description: Converts all elements to lower case
;// Receives: address of string in edx
;// Returns: noting, but string is now lower case.
.data
opt2prompt1 byte "Original :", newline , 0
opt2prompt2 byte "Modified :", newline,0
.code
push edx
mov edx, offset opt2prompt1
call writestring
pop edx
call option5
call crlf
push edx
L2:
mov al, byte ptr [edx+esi]
cmp al, 41h
jb keepgoing ;// not a letter
cmp al, 5ah
ja keepgoing ;// not a letter
or al, 20h ;//could use add al, to convert to lower case.
mov byte ptr [edx+esi], al ;// letter now lower case
keepgoing:
inc esi
loop L2
mov edx, offset opt2prompt2
call writestring
pop edx
call option5
call waitmsg
ret
option2 endp
option5 proc
;// Description: Displays the string.
;// Receives: address of string in edx
;// Returns: nothing
.data
option5prompt byte 'The String is: ', 0h
.code
push edx ;// save the address of the string to write prompt
mov edx, offset option5prompt
call writestring
pop edx
call writestring ;// write the string
call crlf
ret
option5 endp
END main
Step by Step Solution
There are 3 Steps involved in it
Step: 1
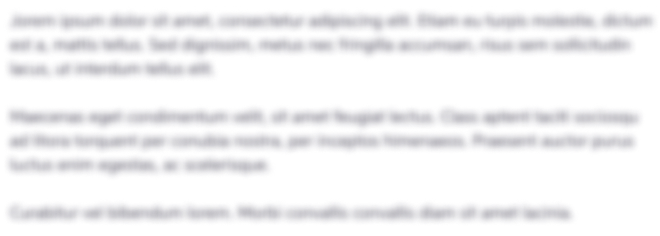
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started