Please write in c++ showing comments of what is being done. Thank you. Existing code is pasted below question.
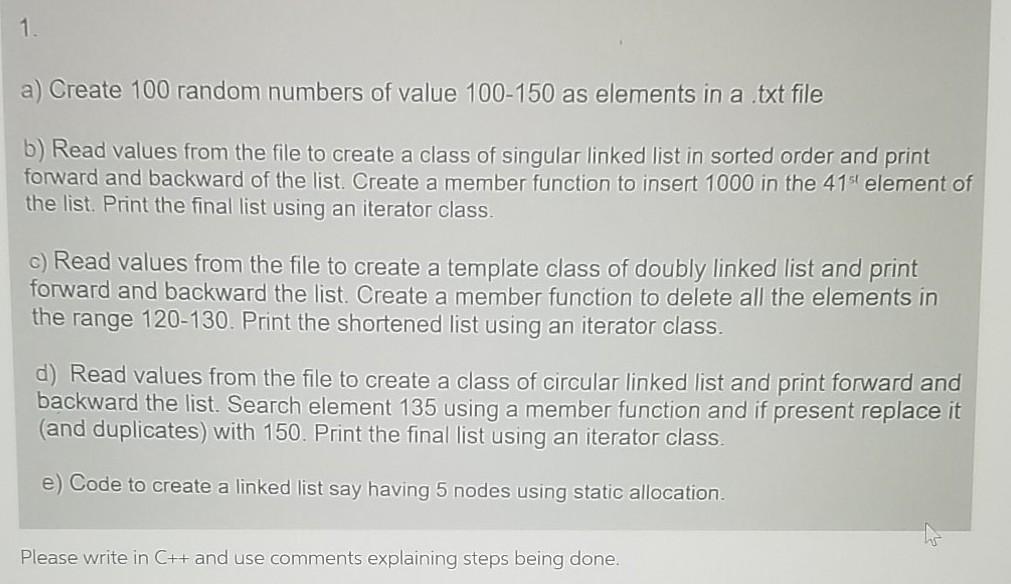
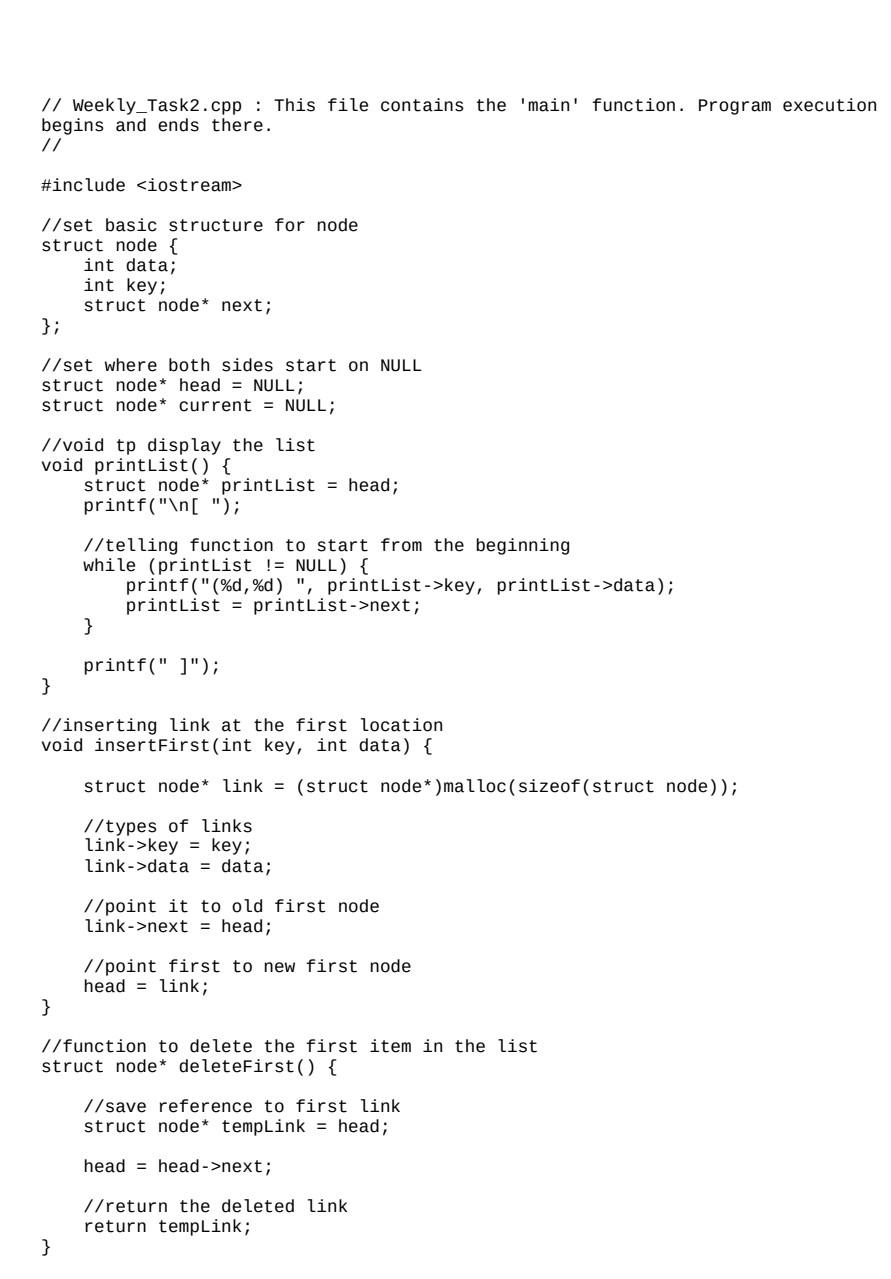
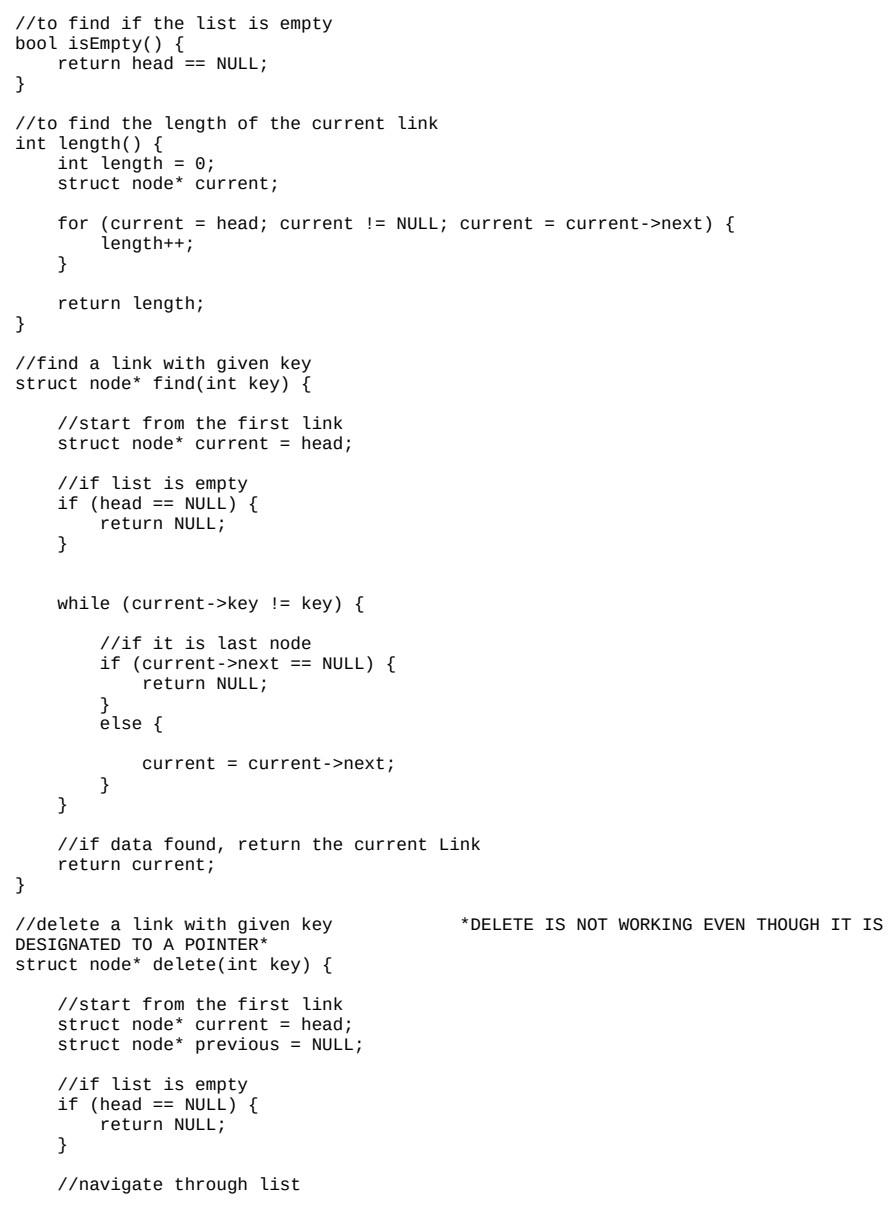
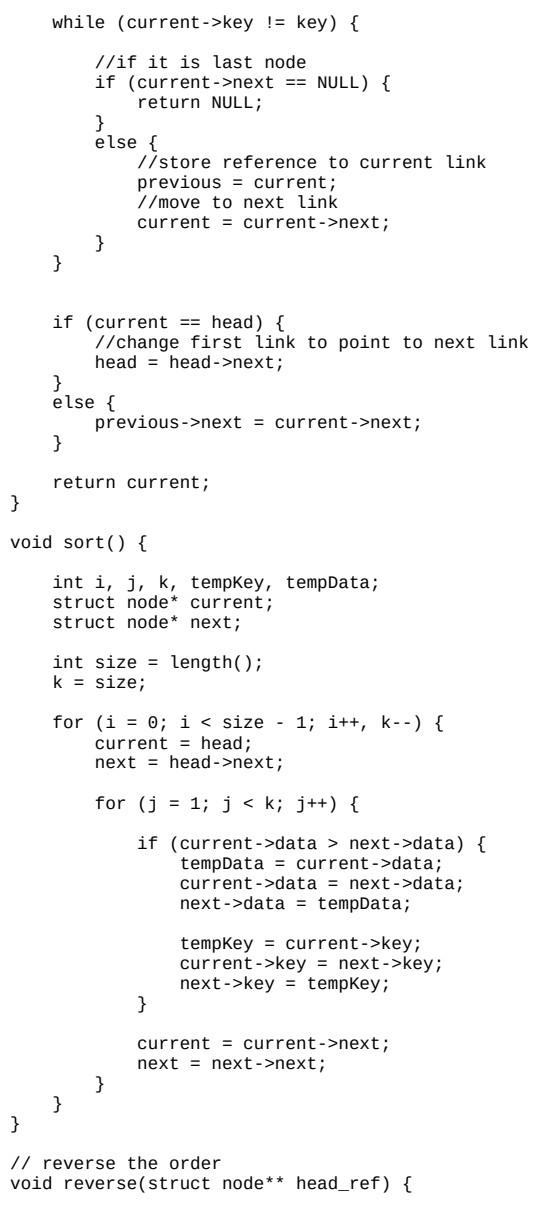
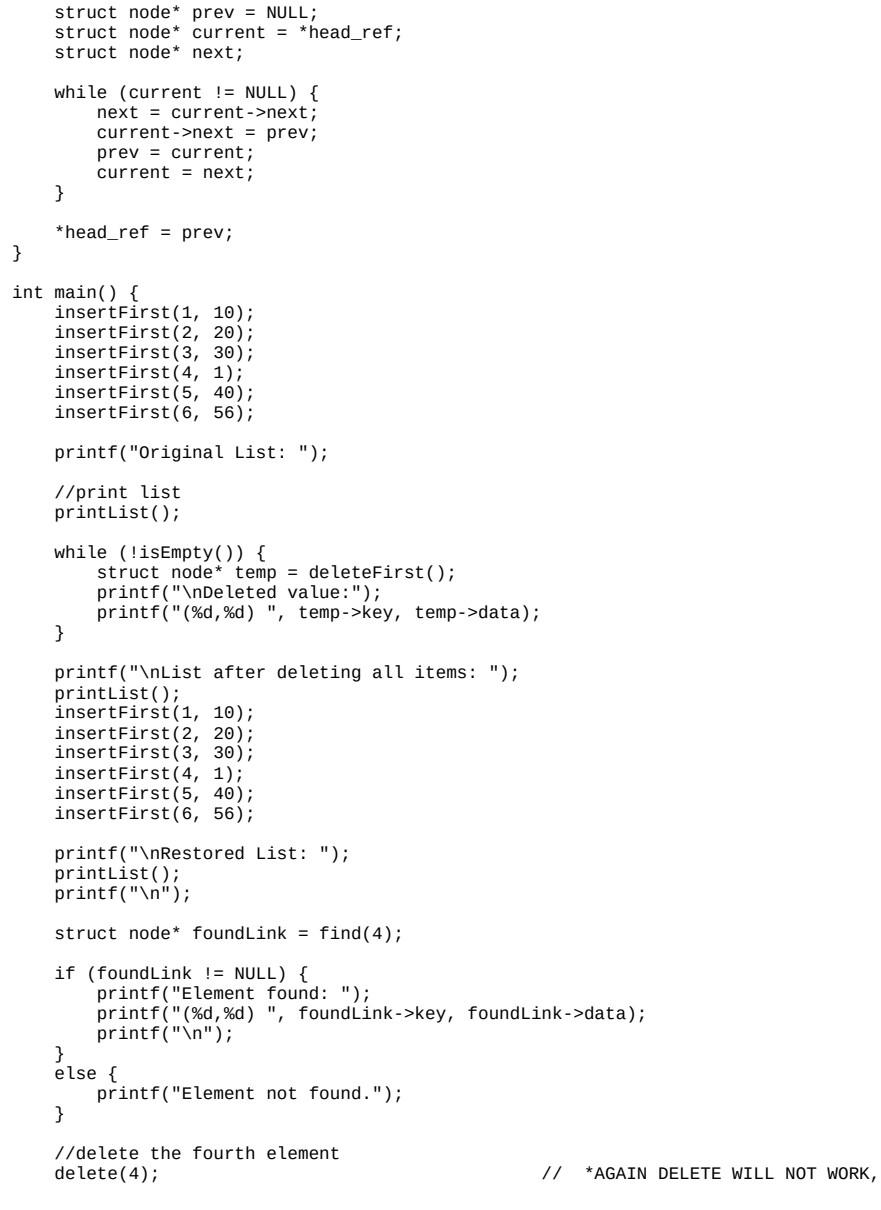
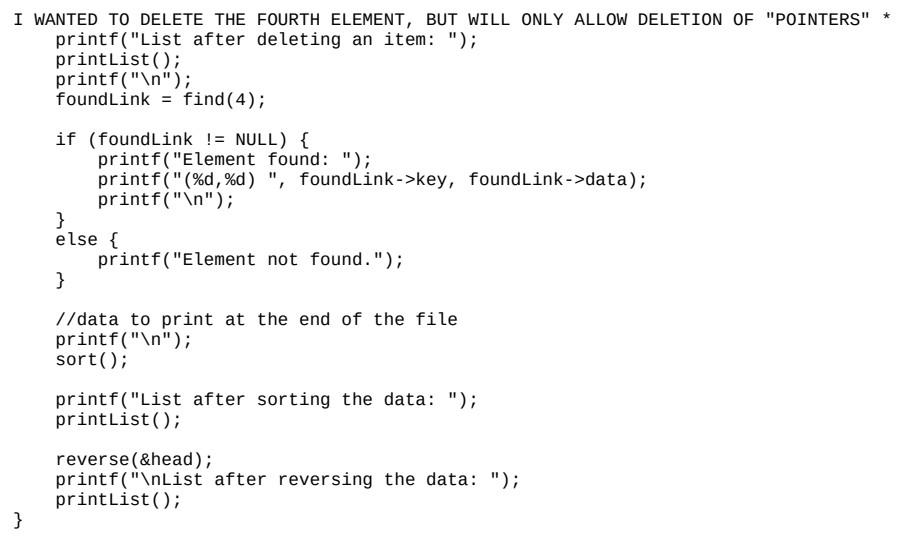
Existing code to change:
#include
//set basic structure for node
struct node {
int data;
int key;
struct node* next;
};
//set where both sides start on NULL
struct node* head = NULL;
struct node* current = NULL;
//void tp display the list
void printList() {
struct node* printList = head;
printf(" [ ");
//telling function to start from the beginning
while (printList != NULL) {
printf("(%d,%d) ", printList->key, printList->data);
printList = printList->next;
}
printf(" ]");
}
//inserting link at the first location
void insertFirst(int key, int data) {
struct node* link = (struct node*)malloc(sizeof(struct node));
//types of links
link->key = key;
link->data = data;
//point it to old first node
link->next = head;
//point first to new first node
head = link;
}
//function to delete the first item in the list
struct node* deleteFirst() {
//save reference to first link
struct node* tempLink = head;
head = head->next;
//return the deleted link
return tempLink;
}
//to find if the list is empty
bool isEmpty() {
return head == NULL;
}
//to find the length of the current link
int length() {
int length = 0;
struct node* current;
for (current = head; current != NULL; current = current->next) {
length++;
}
return length;
}
//find a link with given key
struct node* find(int key) {
//start from the first link
struct node* current = head;
//if list is empty
if (head == NULL) {
return NULL;
}
while (current->key != key) {
//if it is last node
if (current->next == NULL) {
return NULL;
}
else {
current = current->next;
}
}
//if data found, return the current Link
return current;
}
//delete a link with given key *DELETE IS NOT WORKING EVEN THOUGH IT IS DESIGNATED TO A POINTER*
struct node* delete(int key) {
//start from the first link
struct node* current = head;
struct node* previous = NULL;
//if list is empty
if (head == NULL) {
return NULL;
}
/avigate through list
while (current->key != key) {
//if it is last node
if (current->next == NULL) {
return NULL;
}
else {
//store reference to current link
previous = current;
//move to next link
current = current->next;
}
}
if (current == head) {
//change first link to point to next link
head = head->next;
}
else {
previous->next = current->next;
}
return current;
}
void sort() {
int i, j, k, tempKey, tempData;
struct node* current;
struct node* next;
int size = length();
k = size;
for (i = 0; i
current = head;
next = head->next;
for (j = 1; j
if (current->data > next->data) {
tempData = current->data;
current->data = next->data;
next->data = tempData;
tempKey = current->key;
current->key = next->key;
next->key = tempKey;
}
current = current->next;
next = next->next;
}
}
}
// reverse the order
void reverse(struct node** head_ref) {
struct node* prev = NULL;
struct node* current = *head_ref;
struct node* next;
while (current != NULL) {
next = current->next;
current->next = prev;
prev = current;
current = next;
}
*head_ref = prev;
}
int main() {
insertFirst(1, 10);
insertFirst(2, 20);
insertFirst(3, 30);
insertFirst(4, 1);
insertFirst(5, 40);
insertFirst(6, 56);
printf("Original List: ");
//print list
printList();
while (!isEmpty()) {
struct node* temp = deleteFirst();
printf(" Deleted value:");
printf("(%d,%d) ", temp->key, temp->data);
}
printf(" List after deleting all items: ");
printList();
insertFirst(1, 10);
insertFirst(2, 20);
insertFirst(3, 30);
insertFirst(4, 1);
insertFirst(5, 40);
insertFirst(6, 56);
printf(" Restored List: ");
printList();
printf(" ");
struct node* foundLink = find(4);
if (foundLink != NULL) {
printf("Element found: ");
printf("(%d,%d) ", foundLink->key, foundLink->data);
printf(" ");
}
else {
printf("Element not found.");
}
//delete the fourth element
delete(4); // *AGAIN DELETE WILL NOT WORK, I WANTED TO DELETE THE FOURTH ELEMENT, BUT WILL ONLY ALLOW DELETION OF "POINTERS" *
printf("List after deleting an item: ");
printList();
printf(" ");
foundLink = find(4);
if (foundLink != NULL) {
printf("Element found: ");
printf("(%d,%d) ", foundLink->key, foundLink->data);
printf(" ");
}
else {
printf("Element not found.");
}
//data to print at the end of the file
printf(" ");
sort();
printf("List after sorting the data: ");
printList();
reverse(&head);
printf(" List after reversing the data: ");
printList();
}
This is what I was asked to do, and what the existing code was doing. These are the steps that were given for the existing code listed above:
- Create 10 random numbers of value 100-150 as elements of a doubly linked list and circular linked list. Perform the following tasks (write functions and calling them in main())
- Create both lists by insert begin and print each of them.
- Insert 1000 at the beginning of the circular list and print. Draw a picture as how will perform the task. Print the list.
- Insert 3000 at the 5th position of the doubly linked list. Draw a picture to show how insertion happened. Print the list.
- Delete 4th element from the circular list. Draw a picture to show how deletion happened. Print the list.
- Convert the doubly linked list to a doubly linked circular list. How will you confirm this list is circular?
- Create a sorted doubly linked list by inserting the generated random numbers one by one in an ascending order.
1. a) Create 100 random numbers of value 100-150 as elements in a txt file b) Read values from the file to create a class of singular linked list in sorted order and print forward and backward of the list. Create a member function to insert 1000 in the 41 element of the list. Print the final list using an iterator class. c) Read values from the file to create a template class of doubly linked list and print forward and backward the list. Create a member function to delete all the elements in the range 120-130. Print the shortened list using an iterator class. d) Read values from the file to create a class of circular linked list and print forward and backward the list. Search element 135 using a member function and if present replace it (and duplicates) with 150. Print the final list using an iterator class e) Code to create a linked list say having 5 nodes using static allocation. Please write in C++ and use comments explaining steps being done. // Weekly_Task2.cpp: This file contains the 'main' function. Program execution begins and ends there. #include
//set basic structure for node struct node { int data; int key; struct node* next; }; //set where both sides start on NULL struct node* head = NULL; struct node* current = NULL; //void tp display the list void printList() { struct node* printList = head; printf(" [ "); //telling function to start from the beginning while (printList != NULL) { printf("%d, %d) ", printList->key, printList->data); printList = printList->next; } printf("]"); } //inserting link at the first location void insertFirst(int key, int data) { struct node* link = (struct node*)malloc(sizeof(struct node)); //types of links link->key = key; link->data = data; //point it to old first node link->next = head; //point first to new first node head = link; } //function to delete the first item in the list struct node* deleteFirst() { //save reference to first link struct node* tempLink = head; head = head->next; //return the deleted link return tempLink; } //to find if the list is empty bool isEmpty() { return head == NULL; } //to find the length of the current link int length() { int length = 0; struct node* current; for (current = head; current != NULL; current = current->next) { length++; } return length; } //find a link with given key struct node* find(int key) { //start from the first link struct node* current = head; //if list is empty if (head == NULL) { return NULL; } while (current->key != key) { //if it is last node if (current->next == NULL) { return NULL; else { current = current->next; } } //if data found, return the current Link return current; } *DELETE IS NOT WORKING EVEN THOUGH IT IS 1/delete a link with given key DESIGNATED TO A POINTER* struct node* delete(int key) { //start from the first link struct node* current = head; struct node* previous = NULL; //if list is empty if (head == NULL) { return NULL; } 1avigate through list while (current->key != key) { //if it is last node if (current->next == NULL) { return NULL; } else { //store reference to current link previous = current; //move to next link current = current->next; } } == if (current head) { //change first link to point to next link head = head->next; } else { previous->next = current->next; } return current; } void sort() { int i, j, k, tempkey, tempData; struct node* current; struct node* next; int size = length(); k = size; for (i = 0; i next; for (j = 1; j data > next->data) { tempData = current->data; current->data = next->data; next->data = tempData; tempkey = current->key; current ->key = next ->key; next->key = tempkey; } current = current->next; next = next->next; } } } // reverse the order void reverse(struct node** head_ref) { struct node* prev = NULL; struct node* current = *head_ref; struct node* next; while (current != NULL) { next = current->next; current->next = prev; prev = current; current = next; } *head_ref = prev; } int main() { insertFirst(1, 10); insert First(2, 20); insertFirst(3, 30); insert First(4, 1); insertFirst(5, 40); insertFirst(6, 56); printf("Original List: "); //print list printList(); while (!isEmpty()) { struct node* temp = deleteFirst(); printf(" Deleted value:"); printf("%d,%d) ", temp->key, temp->data); } printf(" List after deleting all items: "); printList(); insert First(1, 10); insert First(2, 20); insert First(3, 30); insert First(4, 1); insert First(5, 40); insertFirst(6, 56); printf(" Restored List: "); printList(); printf(" "); struct node* foundLink = find(4); if (foundLink != NULL) { printf("Element found: "); printf("%d,%d) ", foundLink ->key, foundLink->data); printf(" "); } else { printf("Element not found."); } //delete the fourth element delete(4); // *AGAIN DELETE WILL NOT WORK, I WANTED TO DELETE THE FOURTH ELEMENT, BUT WILL ONLY ALLOW DELETION OF "POINTERS" * printf("List after deleting an item: "); printList(); printf(" "); foundLink = find(4); if (foundLink != NULL) { printf("Element found: "); printf("%d, %d ", foundLink->key, foundLink->data); printf(" "); } else { printf("Element not found."); } //data to print at the end of the file printf(" "); sort(); printf("List after sorting the data: "); printList(); reverse(&head); printf(" List after reversing the data: "); printList(); } 1. a) Create 100 random numbers of value 100-150 as elements in a txt file b) Read values from the file to create a class of singular linked list in sorted order and print forward and backward of the list. Create a member function to insert 1000 in the 41 element of the list. Print the final list using an iterator class. c) Read values from the file to create a template class of doubly linked list and print forward and backward the list. Create a member function to delete all the elements in the range 120-130. Print the shortened list using an iterator class. d) Read values from the file to create a class of circular linked list and print forward and backward the list. Search element 135 using a member function and if present replace it (and duplicates) with 150. Print the final list using an iterator class e) Code to create a linked list say having 5 nodes using static allocation. Please write in C++ and use comments explaining steps being done. // Weekly_Task2.cpp: This file contains the 'main' function. Program execution begins and ends there. #include //set basic structure for node struct node { int data; int key; struct node* next; }; //set where both sides start on NULL struct node* head = NULL; struct node* current = NULL; //void tp display the list void printList() { struct node* printList = head; printf(" [ "); //telling function to start from the beginning while (printList != NULL) { printf("%d, %d) ", printList->key, printList->data); printList = printList->next; } printf("]"); } //inserting link at the first location void insertFirst(int key, int data) { struct node* link = (struct node*)malloc(sizeof(struct node)); //types of links link->key = key; link->data = data; //point it to old first node link->next = head; //point first to new first node head = link; } //function to delete the first item in the list struct node* deleteFirst() { //save reference to first link struct node* tempLink = head; head = head->next; //return the deleted link return tempLink; } //to find if the list is empty bool isEmpty() { return head == NULL; } //to find the length of the current link int length() { int length = 0; struct node* current; for (current = head; current != NULL; current = current->next) { length++; } return length; } //find a link with given key struct node* find(int key) { //start from the first link struct node* current = head; //if list is empty if (head == NULL) { return NULL; } while (current->key != key) { //if it is last node if (current->next == NULL) { return NULL; else { current = current->next; } } //if data found, return the current Link return current; } *DELETE IS NOT WORKING EVEN THOUGH IT IS 1/delete a link with given key DESIGNATED TO A POINTER* struct node* delete(int key) { //start from the first link struct node* current = head; struct node* previous = NULL; //if list is empty if (head == NULL) { return NULL; } 1avigate through list while (current->key != key) { //if it is last node if (current->next == NULL) { return NULL; } else { //store reference to current link previous = current; //move to next link current = current->next; } } == if (current head) { //change first link to point to next link head = head->next; } else { previous->next = current->next; } return current; } void sort() { int i, j, k, tempkey, tempData; struct node* current; struct node* next; int size = length(); k = size; for (i = 0; i next; for (j = 1; j data > next->data) { tempData = current->data; current->data = next->data; next->data = tempData; tempkey = current->key; current ->key = next ->key; next->key = tempkey; } current = current->next; next = next->next; } } } // reverse the order void reverse(struct node** head_ref) { struct node* prev = NULL; struct node* current = *head_ref; struct node* next; while (current != NULL) { next = current->next; current->next = prev; prev = current; current = next; } *head_ref = prev; } int main() { insertFirst(1, 10); insert First(2, 20); insertFirst(3, 30); insert First(4, 1); insertFirst(5, 40); insertFirst(6, 56); printf("Original List: "); //print list printList(); while (!isEmpty()) { struct node* temp = deleteFirst(); printf(" Deleted value:"); printf("%d,%d) ", temp->key, temp->data); } printf(" List after deleting all items: "); printList(); insert First(1, 10); insert First(2, 20); insert First(3, 30); insert First(4, 1); insert First(5, 40); insertFirst(6, 56); printf(" Restored List: "); printList(); printf(" "); struct node* foundLink = find(4); if (foundLink != NULL) { printf("Element found: "); printf("%d,%d) ", foundLink ->key, foundLink->data); printf(" "); } else { printf("Element not found."); } //delete the fourth element delete(4); // *AGAIN DELETE WILL NOT WORK, I WANTED TO DELETE THE FOURTH ELEMENT, BUT WILL ONLY ALLOW DELETION OF "POINTERS" * printf("List after deleting an item: "); printList(); printf(" "); foundLink = find(4); if (foundLink != NULL) { printf("Element found: "); printf("%d, %d ", foundLink->key, foundLink->data); printf(" "); } else { printf("Element not found."); } //data to print at the end of the file printf(" "); sort(); printf("List after sorting the data: "); printList(); reverse(&head); printf(" List after reversing the data: "); printList(); }