Question
Please write JUnit test for EndlessList Class. Thank you //////////////////////////////////////////////////////////// node class (already written) public class Node { //fields private Node prev; private Node next;
Please write JUnit test for EndlessList Class. Thank you
//////////////////////////////////////////////////////////// node class (already written)
public class Node
//fields private Node prev; private Node next; private E value; /** * Constructor that sets prev and next to null. * * @param value the value to enter in this node */ public Node(E value) { this.value = value; } /** * Full constructor. * * @param value the value to enter in this node * @param prev the node just before this one * @param next the node just after this one */ public Node(E value, Node prev, Node next) { this(value); this.prev = prev; this.next = next; }
/** * Provides access to the previous node. * * @return the previous node */ public Node getPrev() { return prev; }
/** * Allows the previous node to be set. * * @param prev the previous node */ public void setPrev(Node prev) { this.prev = prev; }
/** * Provides access to the next node. * * @return the next node */ public Node getNext() { return next; }
/** * Allows the next node to be set. * * @param next the next node */ public void setNext(Node next) { this.next = next; }
/** * Provides access to the value stored in this node * * @return the value */ public E getValue() { return value; }
/** * Allows the value stored in this node to be set. * * @param value the value */ public void setValue(E value) { this.value = value; } }
/////////////////////////////////////////////////////////////////////////////////////Endlesslist class////////////////////
import java.util.Iterator;
/** * This data class represents an ordered collection in an endless circular list. * * @param
//fields private Node
/** * Adds a value before the current one and moves the cursor to the new * value. If the list is empty the value is simply added and becomes the * current one. * * @param value the value to add to the list */ public void addPrev(E value) { // TODO write method body // }
/** * Adds a value after the current one and moves the cursor to the new value. * If the list is empty the value is simply added and becomes the current * one. * * @param value the value to add to the list */ public void addNext(E value) { // TODO write method body // }
/** * Removes the current value from the list and moves the cursor to the next * value, returning the removed value. Returns a null if the list is empty. * If this is the last value in the list the cursor becomes null. * * @return the value removed */ public E remove() { // TODO write method body // return null; }
/** * Returns the value at the current cursor position. Returns a null if the * list is empty. * * @return the value */ public E getValue() { // TODO write method body // return null; }
/** * Changes the current value at the current cursor position. Returns false * if the list is empty and true if the change is made. * * @param value the new value * @return true if successful, false if not */ public boolean setValue(E value) { // TODO write method body // return false; }
/** * Moves the cursor to the previous value in the list and returns that * value. Returns a null if the list is empty. * * @return the value */ public E getPrev() { // TODO write method body // return null; }
/** * Moves the cursor to the next value in the list and returns that value. * Returns null if the list is empty. * * @return the value */ public E getNext() { // TODO write method body // return null; }
/** * Moves the cursor to the next occurrence of the given value, moving * forward in the list. If the value is not found the cursor remains at the * same position in the list. * * @param value the value to search for * @return true if the value is found, false if not */ public boolean moveToNext(E value) { // TODO write method body // return false; }
/** * Moves the cursor to the next occurrence of the given value, moving * backwards in the list. If the value is not found the cursor remains at * the same position in the list. * * @param value the value to search for * @return true if the value is found, false if not */ public boolean moveToPrev(E value) { // TODO write method body // return false; }
/** * Provides and EndlessList iterator. * * @return the iterator */ @Override public Iterator
/** * Private class used to create an EndlessList iterator. */ private class EndlessListIterator implements Iterator
//fields private Node
/** * Reports if the current node has not been reported yet by calling * next(). Returns false if the list is empty. * * @return true if the current node has not been reported, false if it * has */ @Override public boolean hasNext() { // TODO write method body // return false; }
/** * Returns the current value in the list and moves to the next. * * @return the current value, or null if the list is empty */ @Override public E next() { // TODO write method body // return null; }
/** * Removes the last value returned from next(). This assumes that next * is called before each remove. If this is the only value in the list * the cursor becomes null. */ @Override public void remove() { // TODO write method body // }
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
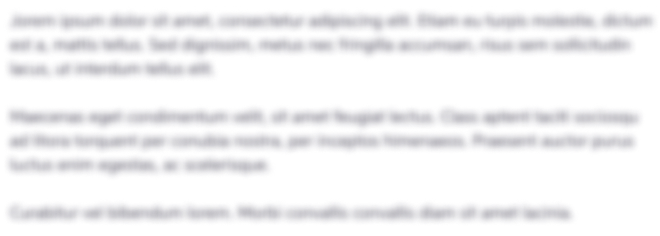
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started