Question
please write the code in java language try to write all methods public void advance( ) { assert wellFormed() : invariant failed at start of
please write the code in java language
try to write all methods
public void advance( ) { assert wellFormed() : "invariant failed at start of advance"; // TODO: Implement this code. assert wellFormed() : "invariant failed at end of advance"; }
/** * Remove the current element from this sequence. * @param - none * @precondition * isCurrent() returns true. * @postcondition * The current element has been removed from this sequence. * There is no longer any current element. * If there was no following element, then we are at the end. * @exception IllegalStateException * Indicates that there is no current element, so * removeCurrent may not be called. **/ public void removeCurrent( ) { assert wellFormed() : "invariant failed at start of removeCurrent"; // TODO: Implement this code. // You will need to shift elements in the array. assert wellFormed() : "invariant failed at end of removeCurrent"; }
/** * Add a new element to this sequence, before the current element (if any). * If the new element would take this sequence beyond its current capacity, * then the capacity is increased before adding the new element. * @param element * the new element that is being added, it is allowed to be null * @postcondition * The element has been added to this sequence. If there was * a current element, then the new element is placed before the current * element. If there was no current element, then the new element is placed * where the removed element was, or at the end. In all cases, the new element becomes the * new current element of this sequence. * @exception OutOfMemoryError * Indicates insufficient memory for increasing the sequence's capacity. * @note * An attempt to increase the capacity beyond * Integer.MAX_VALUE will cause the sequence to fail with an * arithmetic overflow. **/ public void addBefore(Transaction element) { assert wellFormed() : "invariant failed at start of addBefore"; // TODO: Implement this code. assert wellFormed() : "invariant failed at end of addBefore"; }
/** * Add a new element to this sequence, after the current element if any. * If the new element would take this sequence beyond its current capacity, * then the capacity is increased before adding the new element. * @param element * the new element that is being added, may be null * @postcondition * The element has been added to this sequence. If there was * a current element, then the new element is placed after the current * element. If there was no current element, then the new element is placed * where the element was, or at the end of the sequence. * In all cases, the new element becomes the * new current element of this sequence. * @exception OutOfMemoryError * Indicates insufficient memory for increasing the sequence's capacity. * @note * An attempt to increase the capacity beyond * Integer.MAX_VALUE will cause the sequence to fail with an * arithmetic overflow. **/ public void addAfter(Transaction element) { assert wellFormed() : "invariant failed at start of addAfter"; // TODO: Implement this code. assert wellFormed() : "invariant failed at end of addAfter"; }
/** * Place the contents of another sequence at the end of this sequence. * @param addend * a sequence whose contents will be placed at the end of this sequence * @precondition * The parameter, addend, is not null. * @postcondition * The elements from addend have been placed at the end of * this sequence. The current element of this sequence if any, * remains unchanged. The addend is unchanged. * This sequence is 'atEnd' only if it was 'atEnd' before the call, and the * addend was empty. * @exception NullPointerException * Indicates that addend is null. * @exception OutOfMemoryError * Indicates insufficient memory to increase the size of this sequence. * @note * An attempt to increase the capacity beyond * Integer.MAX_VALUE will cause an arithmetic overflow * that will cause the sequence to fail. **/ public void addAll(TransactionSeq addend) { assert wellFormed() : "invariant failed at start of addAll"; // TODO: Implement this code. // Recall that you can freely access private fields of the addend. assert wellFormed() : "invariant failed at end of addAll"; }
/** * Change the current capacity of this sequence. * @param minimumCapacity * the callers desired capacity. The end result must have this * or a greater capacity. * @postcondition * This sequence's capacity has been changed to at least minimumCapacity. * If the capacity was already at or greater than minimumCapacity, * then the capacity is left unchanged. * If the size is changed, it must be at least twice as big as before. * @exception OutOfMemoryError * Indicates insufficient memory for: new array of minimumCapacity elements. **/ private void ensureCapacity(int minimumCapacity) { // TODO: Implement this code. // This is a private method: don't check invariants }
/** * Generate a copy of this sequence. * @param - none * @return * The return value is a copy of this sequence. Subsequent changes to the * copy will not affect the original, nor vice versa. * @exception OutOfMemoryError * Indicates insufficient memory for creating the clone. **/ public TransactionSeq clone( ) { // Clone a TransactionSeq object. assert wellFormed() : "invariant failed at start of clone"; TransactionSeq answer;
try { answer = (TransactionSeq) super.clone( ); } catch (CloneNotSupportedException e) { // This exception should not occur. But if it does, it would probably // indicate a programming error that made super.clone unavailable. // The most common error would be forgetting the "Implements Cloneable" // clause at the start of this class. throw new RuntimeException ("This class does not implement Cloneable"); }
answer.data = data.clone( ); // all that's needed for Homework #2
assert wellFormed() : "invariant failed at end of clone"; assert answer.wellFormed() : "invariant failed for clone"; return answer; }
/** * Class for internal testing. * Do not use in client/application code */ public static class Spy { /** * Return the sink for invariant error messages * @return current reporter */ public Consumer
/** * Change the sink for invariant error messages. * @param r where to send invariant error messages. */ public void setReporter(Consumer
/** * Create an instance of the ADT with give data structure. * This should only be used for testing. * @param d data array * @param m manyItems * @param i isCurrent * @param c currentIndex * @return instance of TransactionSeq with the given field values. */ public TransactionSeq create(Transaction[] d, int m, boolean i, int c) { TransactionSeq result = new TransactionSeq(false); result.data = d; result.manyItems = m; result.isCurrent = i; result.currentIndex = c; return result; } /** * Return whether the wellFormed routine returns true for the argument * @param s transaction seq to check. * @return */ public boolean wellFormed(TransactionSeq s) { return s.wellFormed(); }
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
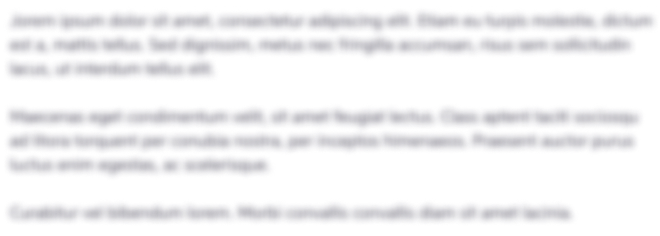
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started