Question
Please write the functions in P ython and also include explanation for each line of code and a snapshot of the code to allow for
Please write the functions in Python and also include explanation for each line of code and a snapshot of the code to allow for viewing of correct indentation, thank you.
Design a class named Rectangle to represent a rectangle.
Question 1 a):
Define a class called Rectangle containing three methods: - __init__ method, or the constructor/initializer method for the class which accepts the width and height of the rectangle as parameters in attribute variables called __width and __height respectively. Note that you are required to define the object variables as private data fields of the class. - The other two methods are the accessor methods for the private variables, named as get_width() and get_height().
More specifically, the Rectangle class contains the following:
- A private int data field named __width that defines the width of the rectangle.
- A private int data field named __height that defines the height of the rectangle.
- A constructor that creates a rectangle with the specified width and height or creates a rectangle with the default values (width=10, height=10). This method should be the special __init__(self, width, height) method, or the initialiser method for the class.
- The get_width(self) method which returns the width of a rectangle.
- The get_height(self) method which returns the height of a rectangle.
Test | Result |
r1 = Rectangle(3, 4) print(r1.get_width(), r1.get_height()) r2 = Rectangle() print(r2.get_width(), r2.get_height()) | 3 4 10 10 |
Question 1 b):
Add a few methods to your Rectangle class to extend its functionality.
- The __str__(self) method which returns a nicely formatted string representation of the object as in the examples below.
- The __repr__(self) method which returns a string that unambiguously describes the object. In this case, the __repr__ method should return a string that looks like the statement that would be used to actually create the object.
Test | Result |
r1 = Rectangle(3, 4) print(r1) print(repr(r1)) r2 = Rectangle() print(r2) print(repr(r2)) | 3 x 4 Rectangle(3, 4) 10 x 10 Rectangle(10, 10) |
Question 1 c):
Add the following two methods to your Rectangle class to extend its functionality.
- The get_area(self) method which returns the area of a rectangle.
- The get_perimeter(self) method which returns the perimeter of a rectangle.
Test | Result |
r1 = Rectangle(3, 4) print(r1.get_area()) print(r1.get_perimeter()) r3 = Rectangle() print(r3.get_area()) print(r3.get_perimeter()) | 12 14 100 40 |
Question 1 d):
Extend the Rectangle class by implementing the __eq__ method. The __eq__(self, other) method corresponds to the "equal to" operator (==). In this case, two rectangles are considered to be equal to each other if and only if they have exactly the same area. The method returns True if the two rectangles are the same, and False otherwise.
Use the isinstance() function to check for cases where a non-Rectangle object is passed to the __eq__ method.
Test | Result |
r = Rectangle(2, 1) s = Rectangle(1, 2) t = Rectangle(2, 2) print(r == s) print(s == r) print(s == t) print(t == 4) | True True False False |
r = Rectangle(2, 1) s = Rectangle() print(r == s) print(s == r) | False False |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
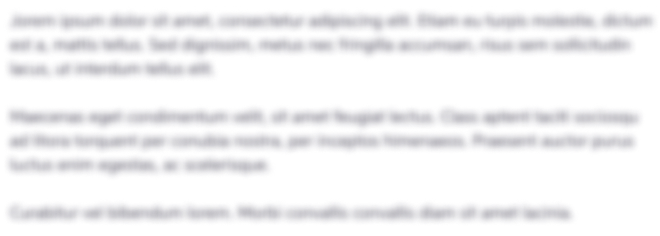
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started