Question
Please write the psuedo code for the following Java code. import java.util.Scanner; // First class OrderBurger public class OrderBurger { public static void main(String[] args)
Please write the psuedo code for the following Java code.
import java.util.Scanner;
// First class OrderBurger
public class OrderBurger {
public static void main(String[] args) { Scanner input = new Scanner(System.in); System.out.println("What type of burger would you like (Vegan or Beef)?: "); String name = input.next(); if(name.equalsIgnoreCase("Vegan")) { Vegan burger = new Vegan(); System.out.println("You have ordered: "); System.out.println(burger); } else if(name.equalsIgnoreCase("Beef")) { Burger burger = new Burger(); System.out.println("What type of bread would you like (White or Wheat)?: "); String bread = input.next(); burger.setBread(bread); System.out.println("What type of drink would you like (Water or Soda)?: "); String drink = input.next(); burger.setDrink(drink); System.out.println("You have ordered: "); System.out.println(burger); } } }
//==================================================================================================================================
Second class Burger
class Burger { private String type; private double cost; private String burger; private String bread; private String drink;
public Burger() { this.type = "Beef"; this.cost = 6.99; } public Burger(String type, double cost) { this.type = type; this.cost = cost; }
public double getCost() { return cost; }
public void setCost() { this.cost += 2; }
public String getType() { return type; }
public void setType(String type) { this.type = type; }
public String getBread() { return bread; }
public String getDrink() { return drink; }
public void setBread(String bread) { this.bread = bread; }
public void setDrink(String drink) { this.drink = drink; setCost(); }
@Override public String toString() { return getType() + " burger " + this.getBread() + " bread" + " " + this.getDrink() + "(+ $0.99) "
+ "Please pay total cost of $"+ this.getCost(); } }
// Third class Vegan
class Vegan extends Burger { public Vegan() { this.setType("Vegan"); setBread(); } public void setBread() { this.setBread("Wheat Bread"); } @Override public String toString() { return getType() + " burger "+ this.getBread() + " "+ "Please pay total cost of $"+ this.getCost(); } }
//==================================================================================================================================
import java.util.Scanner;
public class TotalCost {
private static final int VEGAN_COST = 7; private static final int BEEF_COST = 9; private static final double TIP_COST = 0.1;
private static int getBurger(String bought, Scanner in) { int num = -1; System.out.print(bought); num = in.nextInt();
// Clear keyboard buffer in.nextLine();
// Check if num is negative if (num < 0) { System.out.println("ERROR! Number of burgers can not be negative"); getBurger(bought, in); }
return num; }
private static int getTotal(int noOfVegan, int noOfBeef) { return (noOfVegan * VEGAN_COST) + (noOfBeef * BEEF_COST); }
private static double getTipCost(int total) { return total * TIP_COST; }
public static void main(String[] args) { // Scanner to get user input Scanner in = new Scanner(System.in);
double purchaseCost = 0; // Enter the number of burgers ordered. // A customer can order either vegan or beef, or both burgers. // If only vegan burger is ordered, "please enter the number of beef burgers ordered = 0," and vice versa. int noOfVegan = getBurger("Please enter the number of vegan burgers ordered: ", in); int noOfBeef = getBurger("Please enter the number of beef burgers ordered: ", in);
// Calculate total sale int total = getTotal(noOfVegan, noOfBeef);
// Add tip double tipCost = getTipCost(total);
// Update daily sale purchaseCost += total + tipCost; System.out.println("Your total cost is: $" + purchaseCost); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
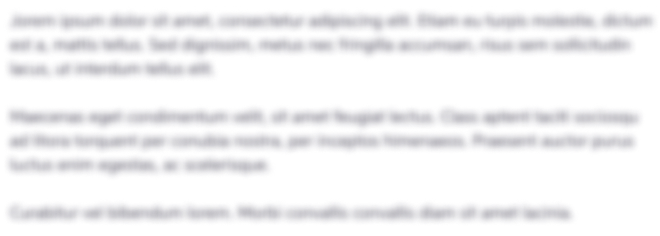
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started