Question
Please write this program in Java with constructor, set, get, implements exception handling, Override 2.33 ( Body Mass Index Calculator ) We introduced the body
Please write this program in Java with constructor, set, get, implements exception handling, Override
2.33 (Body Mass Index Calculator) We introduced the body mass index (BMI) calculator in Exercise 1.10. The formulas for calculating BMI are:
BMI = weightInPounds x 703 heightInInches x heightInInches
Create a BMI calculator that reads the users weight in pounds and height in inches (or, if you prefer, the users weight in kilograms and height in meters), then calculates and displays the users body mass index. Also, display the following information from the Department of Health and Human Services/National Institutes of Health so the user can evaluate his/her BMI.
BMI Categories: Underweight =
Note: Should implements exception handling.
Output:
How many pounds you weight? 200
What is your height in inches? 68
Your BMI is 30.4 (Obese).
These are examples of how BMI should look like:
public class Complex { private int realNumber; /** * Guarda un numero imaginario. */ private int imgNumber; public Complex() { this(0, 0); } public Complex(int realNumber, int imgNumber) { this.realNumber = realNumber; this.imgNumber = imgNumber; } public int getRealNumber() { return realNumber; } public void setRealNumber(int realNumber) { this.realNumber = realNumber; } public int getImgNumber() { return imgNumber; } public void setImgNumber(int imgNumber) { this.imgNumber = imgNumber; } /** * Method that add two complex numbers. * * @param aComplex complex number to be added * @return the addition of two complex numbers */ public Complex add(Complex aComplex) { return new Complex(realNumber + aComplex.realNumber, imgNumber + aComplex.imgNumber); } public Complex subtract(Complex aComplex) { return new Complex(realNumber - aComplex.realNumber, imgNumber - aComplex.imgNumber); } public Complex multiply(Complex aComplex) { int real = realNumber * aComplex.realNumber - imgNumber * aComplex.imgNumber; int img = imgNumber * aComplex.realNumber + realNumber * aComplex.imgNumber; return new Complex(real, img); } public void askComplex() throws BusinessException { Scanner input = new Scanner(System.in); System.out.print("Enter real part: "); realNumber = input.nextInt(); System.out.print("Enter imaginary part: "); imgNumber = input.nextInt(); if(realNumber == 5 && imgNumber == 5){ throw new BusinessException("Complex (5 + 5i) is not valid!"); } } @Override public String toString() { return realNumber + " + " + imgNumber + "i"; } }
---------------------------------------------------------------------------------------------------------------------------------------
public class ComplexDriver { /** * @param args the command line arguments */ public static void main(String[] args) { Complex a = new Complex(6, 9); Complex b = new Complex(5, 1); Complex c; c = a.add(b); System.out.println("A = " + a); System.out.println("B = " + b); System.out.println("A + B = " + c); System.out.println(""); c = a.subtract(b); System.out.println("A = " + a); System.out.println("B = " + b); System.out.println("A - B = " + c); System.out.println(""); c = a.multiply(b); System.out.println("A = " + a); System.out.println("B = " + b); System.out.println("A x B = " + c); System.out.println(""); boolean flag = true; do{ try { b.askComplex(); flag = false; } catch (InputMismatchException inputMismatchException) { System.err.println("You must enter an integer!"); } catch (BusinessException businessException) { System.err.printf("Exception: %s ", businessException); } }while(flag); c = a.add(b); System.out.println("A = " + a); System.out.println("B = " + b); System.out.println("A + B = " + c); } }
-----------------------------------------------------------------------------------------------------------------------------
public class BusinessException extends Exception { public BusinessException() { } public BusinessException(String message) { super(message); } public BusinessException(String message, Throwable cause) { super(message, cause); } public BusinessException(Throwable cause) { super(cause); } public BusinessException(String message, Throwable cause, boolean enableSuppression, boolean writableStackTrace) { super(message, cause, enableSuppression, writableStackTrace); } @Override public String toString() { return "Business Exception: " + " " + getMessage() + "Cause: " + getCause(); } }
----------------------------------------------------------------------------------------------------------------------------------
public class FormulaCuadratica { private int coefA; private int coefB; private int coefC; private double x1; private double x2; public FormulaCuadratica() { this(-1, 0, 0); } public FormulaCuadratica(int coefA, int coefB, int coefC) { setCoefA(coefA); setCoefB(coefB); setCoefC(coefC); } public int getCoefA() { return coefA; } public void setCoefA(int coefA) { if(coefA != 0) { this.coefA = coefA; } else { throw new ArithmeticException("\"a\" can not be zero!"); } } public int getCoefB() { return coefB; } public void setCoefB(int coefB) { this.coefB = coefB; } public int getCoefC() { return coefC; } public void setCoefC(int coefC) { this.coefC = coefC; } public double getX1() { return x1; } public double getX2() { return x2; } private int discriminante() { return (coefB * coefB - 4 * coefA * coefC); } public void calc() { if(discriminante() >= 0) { x1 = (-coefB + Math.sqrt(discriminante())) / (2 * coefA); x2 = (-coefB - Math.sqrt(discriminante())) / (2 * coefA); } else { throw new ArithmeticException("Discriminent is negative!"); } } public void ask() { Scanner input = new Scanner(System.in); System.out.println("Enter the coefficients to calculate the roots"); System.out.println("in the form of aX^2 + bX + c:"); System.out.print("a = "); setCoefA(input.nextInt()); System.out.print("b = "); setCoefB(input.nextInt()); System.out.print("c = "); setCoefC(input.nextInt()); calc(); } @Override public String toString() { return "(" + x1 + ", " + x2 + ")"; } public String equation() { return String.format("%dX^2 + %dX + %d", getCoefA(), getCoefB(), getCoefC()); } public static void main(String[] args) { boolean flag = true; do { try { FormulaCuadratica formulaCuadratica = new FormulaCuadratica(); formulaCuadratica.ask(); System.out.printf("Roots for %s = %s%n", formulaCuadratica.equation(), formulaCuadratica); flag = false; } catch (InputMismatchException inputMismatchException) { System.err.println("Exception: Not a valid number!"); } catch (ArithmeticException arithmeticException) { System.err.println(arithmeticException.getMessage()); } } while (flag); } }2.33 (Body Mass Index Calculator) We introduced the body mass index (BMI) calculator itn Exercise 1.10. The formulas for calculating BMI are BM1 = weigh heightInInches x heightInInches Create a BMI calculator that reads the user's weight in pounds and height in inches (or, if you prefer, the user's weight in kilograms and height in meters), then calculates and displays the user's body mass index. Also, display the following information from the Department of Health and Human Services National Institutes of Health so the user can evaluate his/her BMI BMI Categories: Underweight
Step by Step Solution
There are 3 Steps involved in it
Step: 1
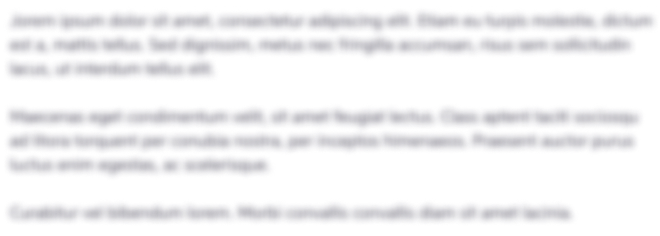
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started