Pls write the full code C++
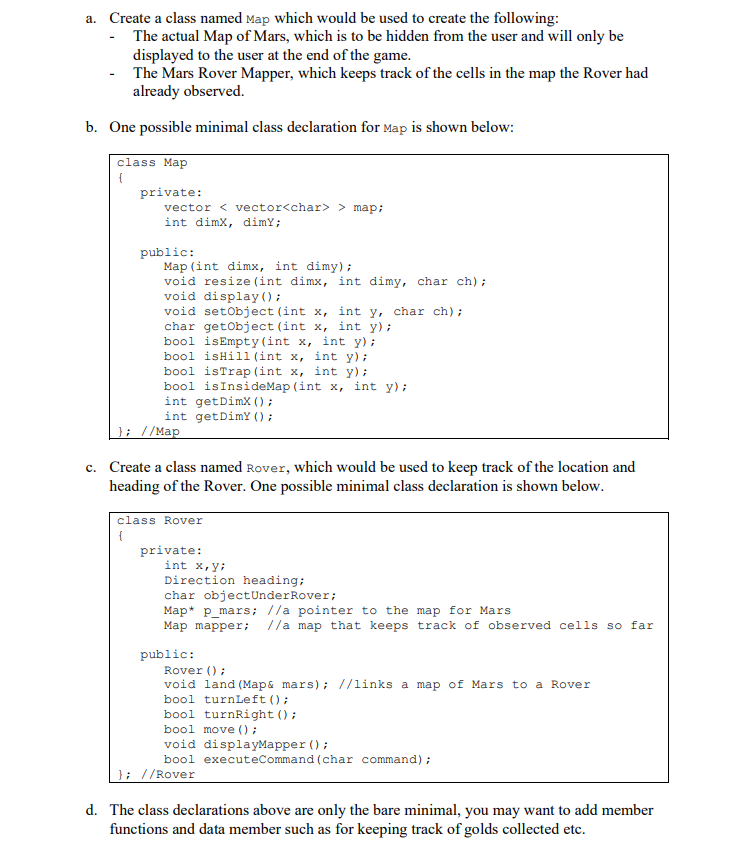
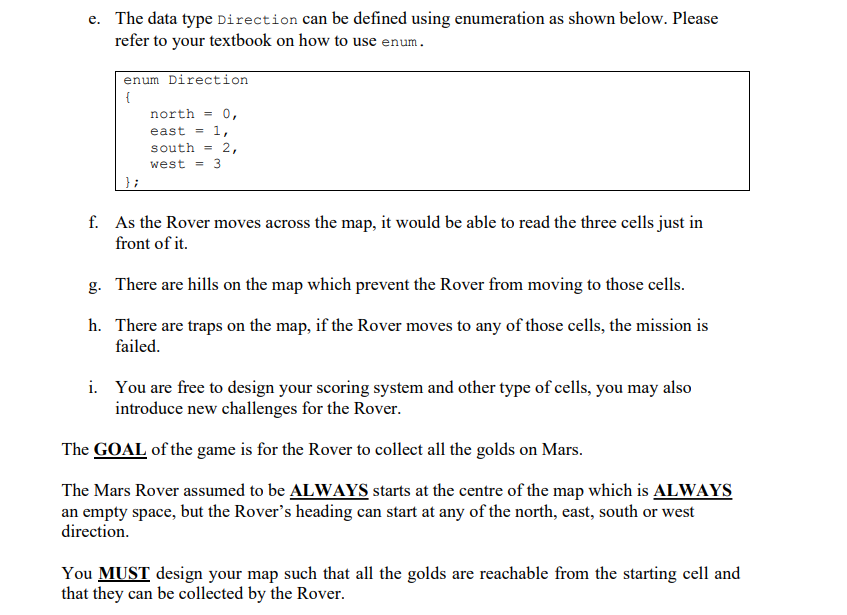
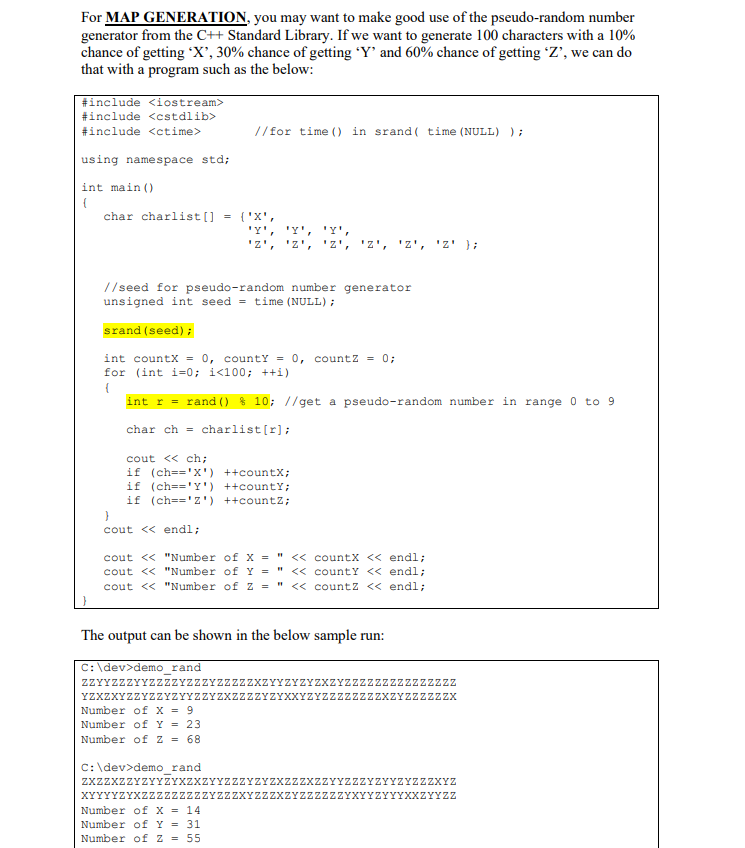
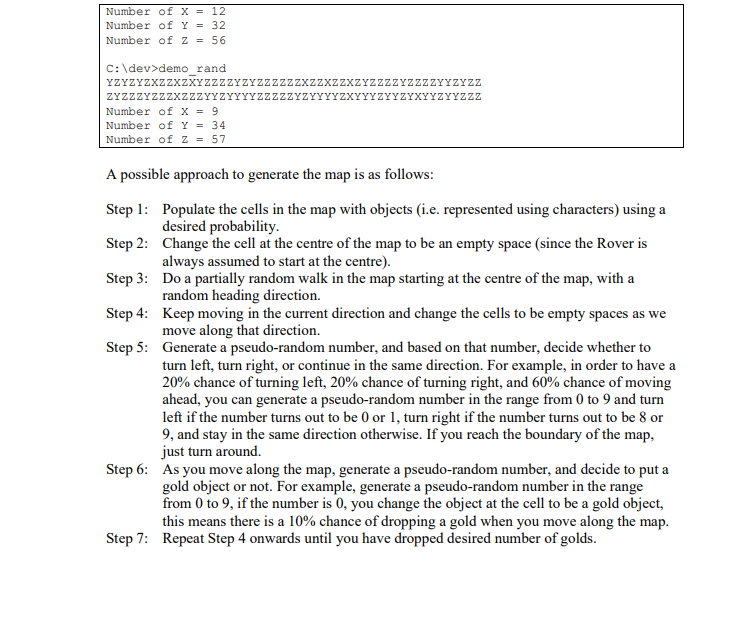
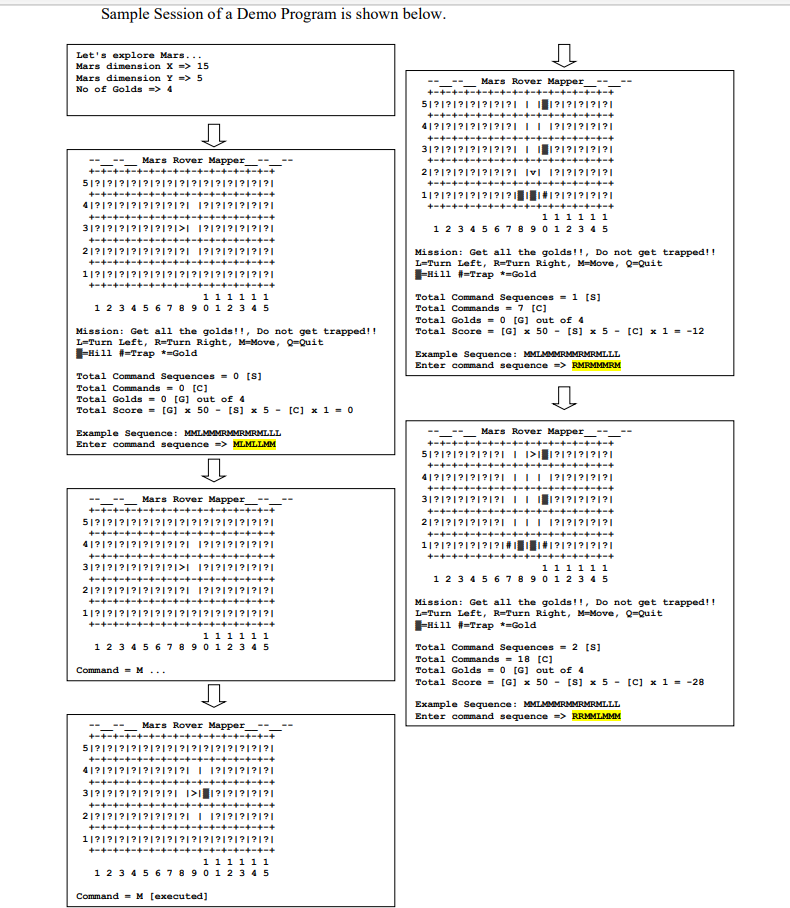
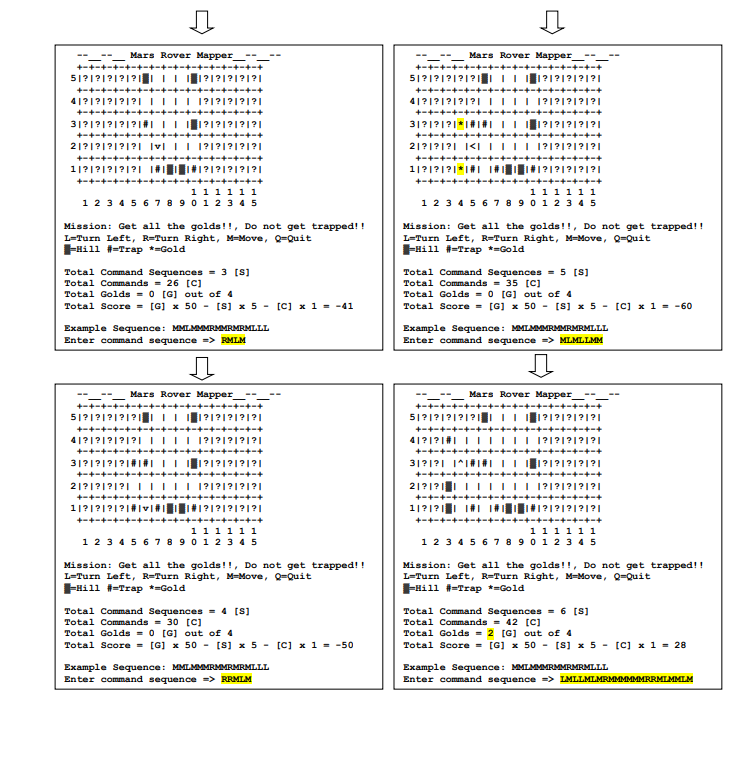
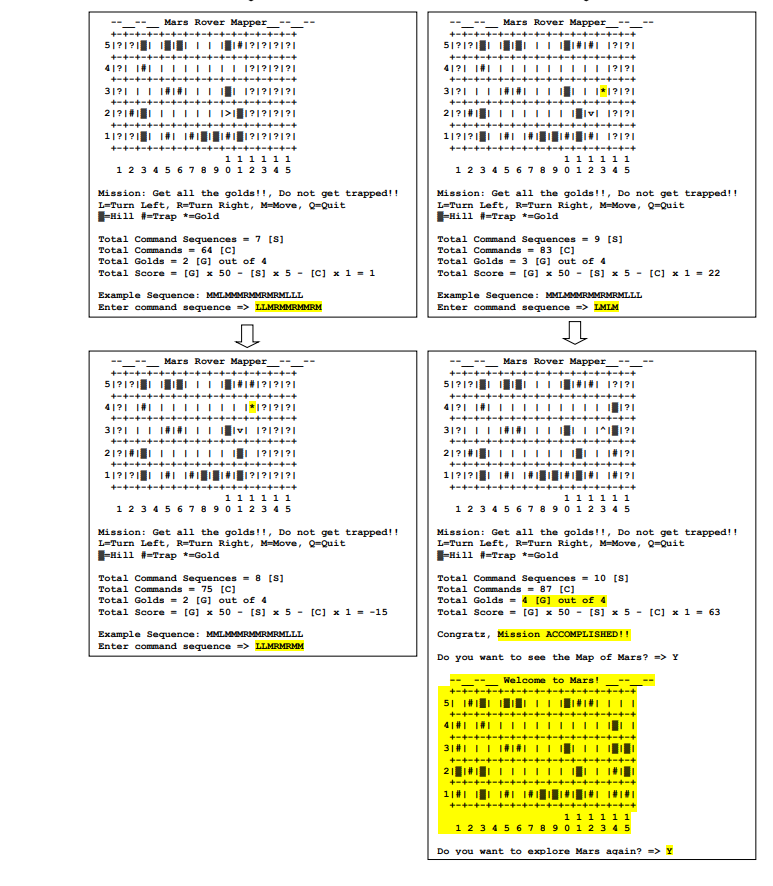
a. Create a class named Map which would be used to create the following: The actual Map of Mars, which is to be hidden from the user and will only be displayed to the user at the end of the game. The Mars Rover Mapper, which keeps track of the cells in the map the Rover had already observed. b. One possible minimal class declaration for Map is shown below: class Map private: vector > map; int dimx, dimy; public: Map (int dimx, int dimy); void resize (int dimx, int dimy, char ch); void display(); void setObject (int x, int y, char ch); char getObject (int x, int y); bool isEmpty(int x, int y); bool isHill(int x, int y); bool isTrap (int x, int y); bool isInsideMap (int x, int y); int get Dimx(); int get Dimy(); }; //Map c. Create a class named Rover, which would be used to keep track of the location and heading of the Rover. One possible minimal class declaration is shown below. class Rover private: int x,y: Direction heading; char object UnderRover; Map* p_mars; //a pointer to the map for Mars Map mapper; //a map that keeps track of observed cells so far public: Rover(); void land (Map& mars); //links a map of Mars to a Rover bool turnleft(); bool turnRight(); bool move(); void displayMapper(); bool executeCommand (char command); }; //Rover d. The class declarations above are only the bare minimal, you may want to add member functions and data member such as for keeping track of golds collected etc. e. The data type Direction can be defined using enumeration as shown below. Please refer to your textbook on how to use enum. enum Direction north = 0, east = 1, south = 2, west = 3 f. As the Rover moves across the map, it would be able to read the three cells just in front of it. g. There are hills on the map which prevent the Rover from moving to those cells. h. There are traps on the map, if the Rover moves to any of those cells, the mission is failed. i. You are free to design your scoring system and other type of cells, you may also introduce new challenges for the Rover. The GOAL of the game is for the Rover to collect all the golds on Mars. The Mars Rover assumed to be ALWAYS starts at the centre of the map which is ALWAYS an empty space, but the Rover's heading can start at any of the north, east, south or west direction. You MUST design your map such that all the golds are reachable from the starting cell and that they can be collected by the Rover. For MAP GENERATION, you may want to make good use of the pseudo-random number generator from the CH Standard Library. If we want to generate 100 characters with a 10% chance of getting "X', 30% chance of getting 'Y' and 60% chance of getting Z', we can do that with a program such as the below: #include
#include #include //for time() in srand( time (NULL)); using namespace std; int main() char charlist[] {'X', 'Y', 'Y', 'Y', 'z', 'z', 'z', 'z', 'z', 'z' ); //seed for pseudo-random number generator unsigned int seed = time (NULL); srand (seed); int countx = 0, county = 0, countz = 0; for (int i=0; idemo_rand ZZYYZZZYYZZZZYZZzyzzzzzxzYYZYZYZXZYzzzzzzzzzzzzzzz YZXZXYZZYZZYZYYZZYZXZZZZYZYXXYZYZZZZZZZZXZYZZZZZZX Number of x = 9 Number of Y = 23 Number of Z = 68 C:\dev>demo_rand ZXZZXZZYZYYZYXZXZYYZZZYZYZXZZZXZZYYZZZYZYYZYZZZXYZ XYYYYZYXZzzzzzzzzzZZZXYZZZXZYZZZZZZYXYYZYYYXXZYYZZ Number of x = 14 Number of Y = 31 Number of z = 55 Number of x = 12 Number of Y = 32 Number of Z = 56 C:\dev>demo_rand YZYZYZXZzxzxzZZZZYZYZZZzzzxzzxzzxzyzZZZYZZZZYYZYZZ ZZYZZZXZZZYYZYYYYZZZZZYZYYYYZXYYYZYYZYXYYZYYZZZ Number of x = 9 Number of Y = 34 Number of 2 - 57 A possible approach to generate the map is as follows: Step 1: Populate the cells in the map with objects (i.e. represented using characters) using a desired probability Step 2: Change the cell at the centre of the map to be an empty space (since the Rover is always assumed to start at the centre). Step 3: Do a partially random walk in the map starting at the centre of the map, with a random heading direction. Step 4: Keep moving in the current direction and change the cells to be empty spaces as we move along that direction. Step 5: Generate a pseudo-random number, and based on that number, decide whether to turn left, turn right, or continue in the same direction. For example, in order to have a 20% chance of turning left, 20% chance of turning right, and 60% chance of moving ahead, you can generate a pseudo-random number in the range from 0 to 9 and turn left if the number turns out to be 0 or 1, turn right if the number turns out to be 8 or 9, and stay in the same direction otherwise. If you reach the boundary of the map, just turn around. Step 6: As you move along the map, generate a pseudo-random number, and decide to put a gold object or not. For example, generate a pseudo-random number in the range from 0 to 9, if the number is 0, you change the object at the cell to be a gold object, this means there is a 10% chance of dropping a gold when you move along the map. Step 7: Repeat Step 4 onwards until you have dropped desired number of golds. Sample Session of a Demo Program is shown below. Let's explore Mars... Mars dimension X => 15 Mars dimension Y => 5 No of Golds => 4 Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|?|?|?|?|?|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 41?|?|?|?|?|?|?|?| ?|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+ 31?|?|?|?|?|?|?|I |?|?|?|?|?|?|| Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|?|?| 11?|?|?|?|?|| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 41?|?|?|?|?|?|? |||?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+ 31?|?|?|?|?|?|?|II|?|?|?|?|?| +-+-+-+-+-+-+ 21?|?|?|?|?|?|?|IV|?|?|?|?|?| +-+-+-+-+-+-+ +-+-+-+-+ 1|?|?|?|?|?|?|?|111#1?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, O=Quit FHill #-Trap *=Gold 21?|?|?|?|?|?|?|?| ?|?|?|?|?|?| +-+-+-+-+ 1|?|?|?|?|?|?|?|?|?|?|?|?|?|?|?|| +-+-+-+-+-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M=Move, Q-Quit FHill #-Trap *=Gold Total Command Sequences = 1 [S] Total Commands = 7 [C] Total Golds = 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -12 Example Sequence: MMLMMMMMRMRMLLL Enter command sequence => RMRMMMRM Total Command Sequences = 0 [S] Total Commands - 0 [C] Total Golds - 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = 0 Example Sequence: MMLMMMMMRMRMLLL Enter command sequence => MLMLLMM Mars Rover Mapper_ +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|?|?|?|?|?|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+ 4|?|?|?|?|?|?|?|?| ?|?|?|?|?|?|| Mars Rover Mapper +-+- 5|?|?|?|?|?|?|I>1?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+- 41?|?|?|?|?|?|II||?|?|?|?|?| +-+-+-+-+ 31?|?|?|?|?|?|II|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+ 2|?|?|?|?|?|?|II?|?|?| +-+-+-+-+-+-+-+-+-+-+- 1|?|?|?|?|?|?I#11011?|?|? +-+-+-+-+-+-+-+-+-+-+ iiiiii 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 +-+- 31?|?|?|?|?|?|?I>I |?|?|?|?|?|?| +-+-+-+-+- 2|?|?|?|?|?|?|?|?| ?|?|?|?|?|?| +-+-+-+-+ 1|?|?|?|?|?|?13 ?|?|?|?|?|?|?| +-+-+-+ 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, O=Quit "Hill #Trap *=Gold Total Command Sequences - 2 (S) Total Commands = 18 [C] Total Golds = 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -28 Command = M... Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => RRMMLMMM Mars Rover Mapper -+-+-+-+-+-+ 51?|?|?|?|?|?|?|?|?|?|?|?|?|?|?| +-+-+-+-+-+-+ -+-+-+-+-+-+ 4|?|?|?|?|?|?|?|?|I |?|?|?|?|?|| +-+-+-+-+-+-+ 31?|?|?|?|?|?|?|I>1?|?|?|?|?| +-+-+-+-+-+- -+-+-+-+-+-+-+-+-+ 2|?|?|?|?|?|?|?|?|I|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+- 1|?|?|?|?|?|?|?|?|?|?|?|?|?|?|?| +-+-+-+-+-+- 1 1 1 1 1 1 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Command = M (executed] Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|II|||?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 4|?|?|?|?|?|IIII|?|?|?|?|?| +-+-+-+- 31?|?|?|?|?|# 11 1?|?|?|?|?1 +-+-+-+-+-+- 21?|?|?|?|?|IV|I?|?|?|?|?| +-+-+-+-+- 1|?|?|?|?|?| #111#1?|?|?|?|?| +-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|II||?|?|? +-+-+-+-+-+-+-+-+-+- 4|?|?|?|?|?|II|||?|?|?|?|?| +-+-+-+-+-+-+ 31?|?|?I*1## |?|?|?|?|?| +-+-+-+-+-+-+ 21??|?|IIII|||?|?|?|?|?| 1|?|?|?I*1#1 #01#1?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, O=Quit Hill #Trap *=Gold Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, Q=Quit Hill #Trap *=Gold Total Command Sequences - 3 (S) Total Commands = 26 [C] Total Golds = 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -41 Total Command Sequences = 5 [S] Total Commands 35 [C] Total Golds = 0 [G] out of 4 Total score = [G] x 50 - [S] x 5 - [C] x 1 = -60 Example Sequence : MMLMMMRMMRMRMLLL Enter command sequence => MLMLLMM Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => RMLM Mars Rover Mapper Mars Rover Mapper +-+-+-+-+-+-+-+-+ 51?|????|| |?|?|?|?|?! +-+-+-+-+- 41?|?|?|?|? !!! |?|?|?|?|?1 +-+-+-+ 31?|?|?|?|#I#1 |?|?|?|?|?1 +-+-+-+-+-+ 21???? 1 ?|?|?|?|? +-+-+-+- 11?|??|? ?|?|?|?1 +-+-+-+- 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M=Move, O=Quit Hill #-Trap *=Gold 51?|?|?|?|?|II|||?|?|?|?|?| +-+-+ +-+-+-+-+-+-+-+-+-+-+-+ 4|?|?|IIIII | ?|?|?|?|?1 +-+-+-+ 31?|?|I*T#I#1 |?|?|?|?|?1 +-+-+-+-+-+ 21?l? |?|?|?|?|?1 11?1?1 |?|?|?|?|?1 +-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, =Quit Hill #Trap *=Gold Total Command Sequences - 4 [S] Total Commands 30 [C] Total Golds = 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -50 Total Command Sequences - 6 [S] Total Commands 42 (C) Total Golds = 2 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = 28 Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => RRMLM Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => LMLLMLMRMMMMMMRRMLMMLM Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51? ? | LIVIL 17171 Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 517121 #171717171 4|?| #1 II????1 +-+-+-+ -+-+-+ 31?11 I???? 1 ?|? +-+-+-+-+-+ 41?1111 +-+- 31?#1 +-+- 21?18 +-+- 11?1?11 I*?|? +-+-+ IV I?? 1?|?|?|? 21?1# +-+ 11?1?1 1 1 1 1 1 1 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M=Move, Q=Quit Hill #Trap *=Gold Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, O=Quit Hill #Trap *=Gold Total Command Sequences = 7 [S] Total Commands - 64 [C] Total Golds - 2 [G] out of 4 Total score = [G] x 50 - [S] x 5 - [C] x 1 = 1 Total Command Sequences = 9 [S] Total Commands = 83 [C] Total Golds = 3 (G) out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = 22 Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => LLMRMMRMMRM Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => LMLM Mars Rover Mapper Mars Rover Mapper +-+-+-+ 51?? +-+-+ 4|?| #1 1#1#1?1 +-+-+-+ 51?|?| +-+-+- I#1 #1#1 1?I?! 1*1??13 31? v |?|?|?| 31?1 21?1 |?|??| 21?1 11?1?11 +-+-+-+ 1iiiii 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 11?1? +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M=Move, Q=Quit Hill #Trap *=Gold Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, Q=Quit Fhill #Trap Gold Total Command Sequences - 8 [S] Total Commands - 75 [C] Total Golds = 2 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -15 Total Command Sequences = 10 [S] Total Commands = 87 [C] Total Golds = 4 [G] out of 4 Total score = [G] x 50 - [S] x 5 - [C] x 1 = 63 Congratz, Mission ACCOMPLISHED!! Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => LLMRMRMM Do you want to see the Map of Mars? => Y Welcome to Mars! +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51 #111 +-+-+-+ 4#1 #1 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Do you want to explore Mars again? => Y a. Create a class named Map which would be used to create the following: The actual Map of Mars, which is to be hidden from the user and will only be displayed to the user at the end of the game. The Mars Rover Mapper, which keeps track of the cells in the map the Rover had already observed. b. One possible minimal class declaration for Map is shown below: class Map private: vector > map; int dimx, dimy; public: Map (int dimx, int dimy); void resize (int dimx, int dimy, char ch); void display(); void setObject (int x, int y, char ch); char getObject (int x, int y); bool isEmpty(int x, int y); bool isHill(int x, int y); bool isTrap (int x, int y); bool isInsideMap (int x, int y); int get Dimx(); int get Dimy(); }; //Map c. Create a class named Rover, which would be used to keep track of the location and heading of the Rover. One possible minimal class declaration is shown below. class Rover private: int x,y: Direction heading; char object UnderRover; Map* p_mars; //a pointer to the map for Mars Map mapper; //a map that keeps track of observed cells so far public: Rover(); void land (Map& mars); //links a map of Mars to a Rover bool turnleft(); bool turnRight(); bool move(); void displayMapper(); bool executeCommand (char command); }; //Rover d. The class declarations above are only the bare minimal, you may want to add member functions and data member such as for keeping track of golds collected etc. e. The data type Direction can be defined using enumeration as shown below. Please refer to your textbook on how to use enum. enum Direction north = 0, east = 1, south = 2, west = 3 f. As the Rover moves across the map, it would be able to read the three cells just in front of it. g. There are hills on the map which prevent the Rover from moving to those cells. h. There are traps on the map, if the Rover moves to any of those cells, the mission is failed. i. You are free to design your scoring system and other type of cells, you may also introduce new challenges for the Rover. The GOAL of the game is for the Rover to collect all the golds on Mars. The Mars Rover assumed to be ALWAYS starts at the centre of the map which is ALWAYS an empty space, but the Rover's heading can start at any of the north, east, south or west direction. You MUST design your map such that all the golds are reachable from the starting cell and that they can be collected by the Rover. For MAP GENERATION, you may want to make good use of the pseudo-random number generator from the CH Standard Library. If we want to generate 100 characters with a 10% chance of getting "X', 30% chance of getting 'Y' and 60% chance of getting Z', we can do that with a program such as the below: #include #include #include //for time() in srand( time (NULL)); using namespace std; int main() char charlist[] {'X', 'Y', 'Y', 'Y', 'z', 'z', 'z', 'z', 'z', 'z' ); //seed for pseudo-random number generator unsigned int seed = time (NULL); srand (seed); int countx = 0, county = 0, countz = 0; for (int i=0; idemo_rand ZZYYZZZYYZZZZYZZzyzzzzzxzYYZYZYZXZYzzzzzzzzzzzzzzz YZXZXYZZYZZYZYYZZYZXZZZZYZYXXYZYZZZZZZZZXZYZZZZZZX Number of x = 9 Number of Y = 23 Number of Z = 68 C:\dev>demo_rand ZXZZXZZYZYYZYXZXZYYZZZYZYZXZZZXZZYYZZZYZYYZYZZZXYZ XYYYYZYXZzzzzzzzzzZZZXYZZZXZYZZZZZZYXYYZYYYXXZYYZZ Number of x = 14 Number of Y = 31 Number of z = 55 Number of x = 12 Number of Y = 32 Number of Z = 56 C:\dev>demo_rand YZYZYZXZzxzxzZZZZYZYZZZzzzxzzxzzxzyzZZZYZZZZYYZYZZ ZZYZZZXZZZYYZYYYYZZZZZYZYYYYZXYYYZYYZYXYYZYYZZZ Number of x = 9 Number of Y = 34 Number of 2 - 57 A possible approach to generate the map is as follows: Step 1: Populate the cells in the map with objects (i.e. represented using characters) using a desired probability Step 2: Change the cell at the centre of the map to be an empty space (since the Rover is always assumed to start at the centre). Step 3: Do a partially random walk in the map starting at the centre of the map, with a random heading direction. Step 4: Keep moving in the current direction and change the cells to be empty spaces as we move along that direction. Step 5: Generate a pseudo-random number, and based on that number, decide whether to turn left, turn right, or continue in the same direction. For example, in order to have a 20% chance of turning left, 20% chance of turning right, and 60% chance of moving ahead, you can generate a pseudo-random number in the range from 0 to 9 and turn left if the number turns out to be 0 or 1, turn right if the number turns out to be 8 or 9, and stay in the same direction otherwise. If you reach the boundary of the map, just turn around. Step 6: As you move along the map, generate a pseudo-random number, and decide to put a gold object or not. For example, generate a pseudo-random number in the range from 0 to 9, if the number is 0, you change the object at the cell to be a gold object, this means there is a 10% chance of dropping a gold when you move along the map. Step 7: Repeat Step 4 onwards until you have dropped desired number of golds. Sample Session of a Demo Program is shown below. Let's explore Mars... Mars dimension X => 15 Mars dimension Y => 5 No of Golds => 4 Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|?|?|?|?|?|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 41?|?|?|?|?|?|?|?| ?|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+ 31?|?|?|?|?|?|?|I |?|?|?|?|?|?|| Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|?|?| 11?|?|?|?|?|| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 41?|?|?|?|?|?|? |||?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+ 31?|?|?|?|?|?|?|II|?|?|?|?|?| +-+-+-+-+-+-+ 21?|?|?|?|?|?|?|IV|?|?|?|?|?| +-+-+-+-+-+-+ +-+-+-+-+ 1|?|?|?|?|?|?|?|111#1?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, O=Quit FHill #-Trap *=Gold 21?|?|?|?|?|?|?|?| ?|?|?|?|?|?| +-+-+-+-+ 1|?|?|?|?|?|?|?|?|?|?|?|?|?|?|?|| +-+-+-+-+-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M=Move, Q-Quit FHill #-Trap *=Gold Total Command Sequences = 1 [S] Total Commands = 7 [C] Total Golds = 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -12 Example Sequence: MMLMMMMMRMRMLLL Enter command sequence => RMRMMMRM Total Command Sequences = 0 [S] Total Commands - 0 [C] Total Golds - 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = 0 Example Sequence: MMLMMMMMRMRMLLL Enter command sequence => MLMLLMM Mars Rover Mapper_ +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|?|?|?|?|?|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+ 4|?|?|?|?|?|?|?|?| ?|?|?|?|?|?|| Mars Rover Mapper +-+- 5|?|?|?|?|?|?|I>1?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+- 41?|?|?|?|?|?|II||?|?|?|?|?| +-+-+-+-+ 31?|?|?|?|?|?|II|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+ 2|?|?|?|?|?|?|II?|?|?| +-+-+-+-+-+-+-+-+-+-+- 1|?|?|?|?|?|?I#11011?|?|? +-+-+-+-+-+-+-+-+-+-+ iiiiii 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 +-+- 31?|?|?|?|?|?|?I>I |?|?|?|?|?|?| +-+-+-+-+- 2|?|?|?|?|?|?|?|?| ?|?|?|?|?|?| +-+-+-+-+ 1|?|?|?|?|?|?13 ?|?|?|?|?|?|?| +-+-+-+ 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, O=Quit "Hill #Trap *=Gold Total Command Sequences - 2 (S) Total Commands = 18 [C] Total Golds = 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -28 Command = M... Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => RRMMLMMM Mars Rover Mapper -+-+-+-+-+-+ 51?|?|?|?|?|?|?|?|?|?|?|?|?|?|?| +-+-+-+-+-+-+ -+-+-+-+-+-+ 4|?|?|?|?|?|?|?|?|I |?|?|?|?|?|| +-+-+-+-+-+-+ 31?|?|?|?|?|?|?|I>1?|?|?|?|?| +-+-+-+-+-+- -+-+-+-+-+-+-+-+-+ 2|?|?|?|?|?|?|?|?|I|?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+- 1|?|?|?|?|?|?|?|?|?|?|?|?|?|?|?| +-+-+-+-+-+- 1 1 1 1 1 1 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Command = M (executed] Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|II|||?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 4|?|?|?|?|?|IIII|?|?|?|?|?| +-+-+-+- 31?|?|?|?|?|# 11 1?|?|?|?|?1 +-+-+-+-+-+- 21?|?|?|?|?|IV|I?|?|?|?|?| +-+-+-+-+- 1|?|?|?|?|?| #111#1?|?|?|?|?| +-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+ 51?|?|?|?|?|II||?|?|? +-+-+-+-+-+-+-+-+-+- 4|?|?|?|?|?|II|||?|?|?|?|?| +-+-+-+-+-+-+ 31?|?|?I*1## |?|?|?|?|?| +-+-+-+-+-+-+ 21??|?|IIII|||?|?|?|?|?| 1|?|?|?I*1#1 #01#1?|?|?|?|?| +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, O=Quit Hill #Trap *=Gold Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, Q=Quit Hill #Trap *=Gold Total Command Sequences - 3 (S) Total Commands = 26 [C] Total Golds = 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -41 Total Command Sequences = 5 [S] Total Commands 35 [C] Total Golds = 0 [G] out of 4 Total score = [G] x 50 - [S] x 5 - [C] x 1 = -60 Example Sequence : MMLMMMRMMRMRMLLL Enter command sequence => MLMLLMM Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => RMLM Mars Rover Mapper Mars Rover Mapper +-+-+-+-+-+-+-+-+ 51?|????|| |?|?|?|?|?! +-+-+-+-+- 41?|?|?|?|? !!! |?|?|?|?|?1 +-+-+-+ 31?|?|?|?|#I#1 |?|?|?|?|?1 +-+-+-+-+-+ 21???? 1 ?|?|?|?|? +-+-+-+- 11?|??|? ?|?|?|?1 +-+-+-+- 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M=Move, O=Quit Hill #-Trap *=Gold 51?|?|?|?|?|II|||?|?|?|?|?| +-+-+ +-+-+-+-+-+-+-+-+-+-+-+ 4|?|?|IIIII | ?|?|?|?|?1 +-+-+-+ 31?|?|I*T#I#1 |?|?|?|?|?1 +-+-+-+-+-+ 21?l? |?|?|?|?|?1 11?1?1 |?|?|?|?|?1 +-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, =Quit Hill #Trap *=Gold Total Command Sequences - 4 [S] Total Commands 30 [C] Total Golds = 0 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -50 Total Command Sequences - 6 [S] Total Commands 42 (C) Total Golds = 2 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = 28 Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => RRMLM Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => LMLLMLMRMMMMMMRRMLMMLM Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51? ? | LIVIL 17171 Mars Rover Mapper +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 517121 #171717171 4|?| #1 II????1 +-+-+-+ -+-+-+ 31?11 I???? 1 ?|? +-+-+-+-+-+ 41?1111 +-+- 31?#1 +-+- 21?18 +-+- 11?1?11 I*?|? +-+-+ IV I?? 1?|?|?|? 21?1# +-+ 11?1?1 1 1 1 1 1 1 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M=Move, Q=Quit Hill #Trap *=Gold Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, O=Quit Hill #Trap *=Gold Total Command Sequences = 7 [S] Total Commands - 64 [C] Total Golds - 2 [G] out of 4 Total score = [G] x 50 - [S] x 5 - [C] x 1 = 1 Total Command Sequences = 9 [S] Total Commands = 83 [C] Total Golds = 3 (G) out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = 22 Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => LLMRMMRMMRM Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => LMLM Mars Rover Mapper Mars Rover Mapper +-+-+-+ 51?? +-+-+ 4|?| #1 1#1#1?1 +-+-+-+ 51?|?| +-+-+- I#1 #1#1 1?I?! 1*1??13 31? v |?|?|?| 31?1 21?1 |?|??| 21?1 11?1?11 +-+-+-+ 1iiiii 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 11?1? +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 111111 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M=Move, Q=Quit Hill #Trap *=Gold Mission: Get all the golds!!, Do not get trapped!! L=Turn Left, R=Turn Right, M-Move, Q=Quit Fhill #Trap Gold Total Command Sequences - 8 [S] Total Commands - 75 [C] Total Golds = 2 [G] out of 4 Total Score = [G] x 50 - [S] x 5 - [C] x 1 = -15 Total Command Sequences = 10 [S] Total Commands = 87 [C] Total Golds = 4 [G] out of 4 Total score = [G] x 50 - [S] x 5 - [C] x 1 = 63 Congratz, Mission ACCOMPLISHED!! Example Sequence: MMLMMMRMMRMRMLLL Enter command sequence => LLMRMRMM Do you want to see the Map of Mars? => Y Welcome to Mars! +-+-+-+-+-+-+-+-+-+-+-+-+-+-+-+ 51 #111 +-+-+-+ 4#1 #1 1 2 3 4 5 6 7 8 9 0 1 2 3 4 5 Do you want to explore Mars again? => Y