Question
plz help with his code, cant find the solution on c++ This assignment tests the concepts of Classes Objective Fully implement and test two classes.
plz help with his code, cant find the solution on c++
This assignment tests the concepts of
Classes
Objective
Fully implement and test two classes.
Problem
You have been hired by a bank to create a program that will process transaction logs.
The banks transactions logs are in the format:
Header row is first line of the file, line starts with a # character
#CustomerNumber Customer Name
Each row after that is a transaction
Traction is Char Char DoubleFirst column is
S = Savings
C = Checking
B = Both savings an checking
Second column is action
D = Deposit
W = Withdrawal
I = Interest rate change (APR changed, no change to balances, interest rate is already divided by number of compoundings). However, it will have to be divided by 100 in your interest formula.
P = Interest posted to account
X = Transfer money from the FIRST COLUMN account to the other
For Example, if the log line has: C X 50.00 This transaction transfers $50 from Checking into Savings
Third column is the amount of money or interest rate that the transaction acts on. If the action is P, this number will be 0, since it is not used.
If insufficient funds to complete a withdraw or transfer, $10 is subtracted from the account instead. This means that accounts can go negative!
Requirements
Reading from file must use read_transaction_log
Output should match the provided example.
Classes are requiredA class for Account
this object will store as private data fields
balance
annual percentage rate (interest)
Minimum Actions
Deposit
Withdraw
Transfer
Post Interest
Set the annual percentage rate
Get the balance
A class for BankCustomerthis object will store as private data fields
Savings account (using Account)
Checking account (using Account)
Customer Number
Customer Name
Minimum Actions:
Get customer name
Get customer number
Get Savings balance
Get Checking balance
processTransationLog
Filename of log should be passed to this function
Function should return the number of transactions processed
Your classes should NOT output anything to stdout under any circumstance or ask for any input. All interaction with the user should be handled in your main driver.
Main Driver
Your main function will create an object of your BankCustomer class. A prompt will ask for a file name that will be used as the transaction log. This file name will be sent to read_transaction_log. You will output the final results. Request only a single a filename via cin, no other input should be needed.
Provided files:
read_transaction_log.h: read_transaction_log should make the act of reading the file easier. See header for documentation.
read_transaction_log.cpp: Implementation of read_transaction_log
DELIVERABLES:
All header and cpp files except read_transaction_log.h and read_transaction_log.cpp.
Your file with your main function must be included.
Other Requirements
Output should match the provided example.
Your code must not add any global variables
For full credit your code should have zero compiler warnings.
Usage of any STL containers will affect grade detrimentally.
Avoid all STL includes other than string.
Only submit the file(s) listed in deliverables, submitting object files, IDE project files, and executables will impact grade.
Dont forget to document your code, see course outline for details.
Usage of topics from future chapters will result in no credit
read_transaction_log includes exception handing to give you friendly errors if you run into file reading issues during your testing. You do NOT need to do any exception handling in your project. You may assume that the filename given by the user exists and is readable. You may also assume the file is in the valid format.
Example output
############################################################# Welcome to Banking Transactions Audit v1.0 by Please provide the filename of the transaction log. Filename: log1 Name: Wendy Lee Number: 1001 Transactions Count: 8 Checking Balance: $97.36 Savings Balance: $166.57 -------------------------------------- Welcome to Banking Transactions Audit v1.0 by Please provide the filename of the transaction log. Filename: log2 Name: Marty Saver Number: 1016 Transactions Count: 6 Checking Balance: $0.00 Savings Balance: $1088.81 -------------------------------------- Welcome to Banking Transactions Audit v1.0 by Please provide the filename of the transaction log. Filename: log3 Name: Bill Gates Number: 1003 Transactions Count: 65 Checking Balance: $3329.16 Savings Balance: $3725.64 -------------------------------------- Welcome to Banking Transactions Audit v1.0 by Please provide the filename of the transaction log. Filename: log4 Name: Mark Smith Number: 1004 Transactions Count: 5 Checking Balance: $90.00 Savings Balance: $190.00 -------------------------------------- Welcome to Banking Transactions Audit v1.0 by Please provide the filename of the transaction log. Filename: log5 Name: Wendy Lee Number: 1001 Transactions Count: 6 Checking Balance: $78.36 Savings Balance: $51.58
LOG.CPP #include "read_transaction_log.h"
void read_transaction_log::setFilename(const std::string& s) { if (fileopen) { fin.close(); fileopen = false; } filename = s; }
customer read_transaction_log::openFile() { char discard; customer file_customer{}; if (!fileopen) { fin.open( filename.c_str(), std::ifstream::in); if ( !fin.fail() ) { fin >> discard >> file_customer.number; //Read customer name which may have a space getline(fin, file_customer.name); if ( !fin.good() ) throw std::runtime_error("Input file corruption detected!"); fileopen = true; } else { throw std::runtime_error("Unable to open/read file!"); } } return file_customer; }
transaction read_transaction_log::getNextTransaction() { if (!fileopen) throw std::runtime_error("File must be opened first, see openFile().");
if ( fin ) { transaction item{};
while ( fin && !isalpha(fin.peek()) ) fin.ignore();
if ( fin && isalpha(fin.peek()) ) { fin >> item.on >> item.action >> item.amount; if ( !fin.good() ) throw std::runtime_error("Input file corruption detected!"); //Got transaction return item; } } throw std::runtime_error("Transaction log issue!"); }
bool read_transaction_log::hasTransactions() { if (!fileopen) throw std::runtime_error("File must be opened first, see openFile().");
if ( fin ) { while ( fin && !isalpha(fin.peek()) ) fin.ignore(); if ( fin && isalpha(fin.peek()) ) return true; }
return false; }
LOG.H
#ifndef PROJECT_CLASS1_BANK_READ_TRANSACTION_LOG_H #define PROJECT_CLASS1_BANK_READ_TRANSACTION_LOG_H
#include
/* NOTE:
This file defines/utilizes structs
The main difference between a struct and class is that in a struct, ALL MEMBER items are PUBLIC.
Unless specified otherwise, your instructor does not want you to define structs. However, you are free to work with ones given to you. :) */
/* struct customer Returned by read_transaction_log::openFile() Will contain the customer number and customer name */ struct customer { unsigned long number; std::string name; };
/* struct transaction Returned by read_transaction_log::getNextTransaction() Will contain the account (aka on) (B, C, or S), action (D, W, I, P, or X), and amount of money */ struct transaction { char on; char action; double amount; };
/* read_transaction_log
Opens up a file and processes line by line a transaction log.
Constructor read_transaction_log( std::string& filename ) Precondition: Filename is a local file that EXISTS and is readable, file should be a text file Postcondition: Object is created
Modification Members / Mutator Methods
void setFilename(const std::string& s) { Precondition: Filename is a local file that EXISTS and is readable, file should be a text file Postcondition: If a file was previously open, it is closed. Filename of object is updated.
void openFile() { Precondition: Filename of object is a local file that EXISTS and is readable, file should be a text file Postcondition: File is opened or exception thrown.
transaction getNextTransaction(); Precondition: File is open and transactions are STILL available, exception otherwise Postcondition: Next transaction is read and returned from the file
bool hasTransactions() const; Precondition: File is open Postcondition: Returns true if at least one transaction is still available to be read from the file.
Value Semantics declaration: It safe to use the copy constructor and the assignment operator Dynamic Memory Usage declaration: None explicitly used, however, strings are on the heap Static & Global variable list: none Invariant declaration: fin will be used to maintain seek pointer to transaction log. fileopen will be true only when the file is open. filename will be the name of the file on the filesystem that will be read. Program will abort when a problem is detected in reading the transaction log.
*/ class read_transaction_log { public: //Constructors read_transaction_log() : fileopen(false) {} explicit read_transaction_log( const std::string& s) : fileopen(false), filename(s) {}; explicit read_transaction_log( const char * s) : fileopen(false), filename(s) {}; //Destructor virtual ~read_transaction_log() { if (fileopen) fin.close(); } //Modification Members / Mutator Methods void setFilename(const std::string& s); transaction getNextTransaction(); customer openFile(); bool hasTransactions(); private: bool fileopen; //Keeps track if file is open or not std::ifstream fin; //Object of file std::string filename; //Name of the file that will be read };
#endif //PROJECT_CLASS1_BANK_READ_
Step by Step Solution
There are 3 Steps involved in it
Step: 1
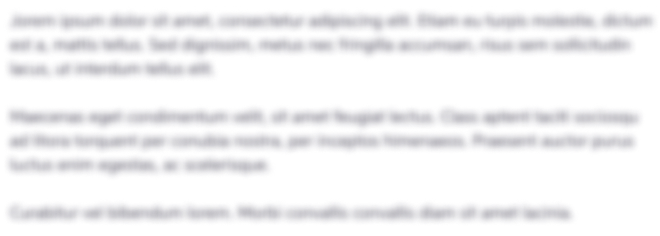
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started