PLZ USE C++ , 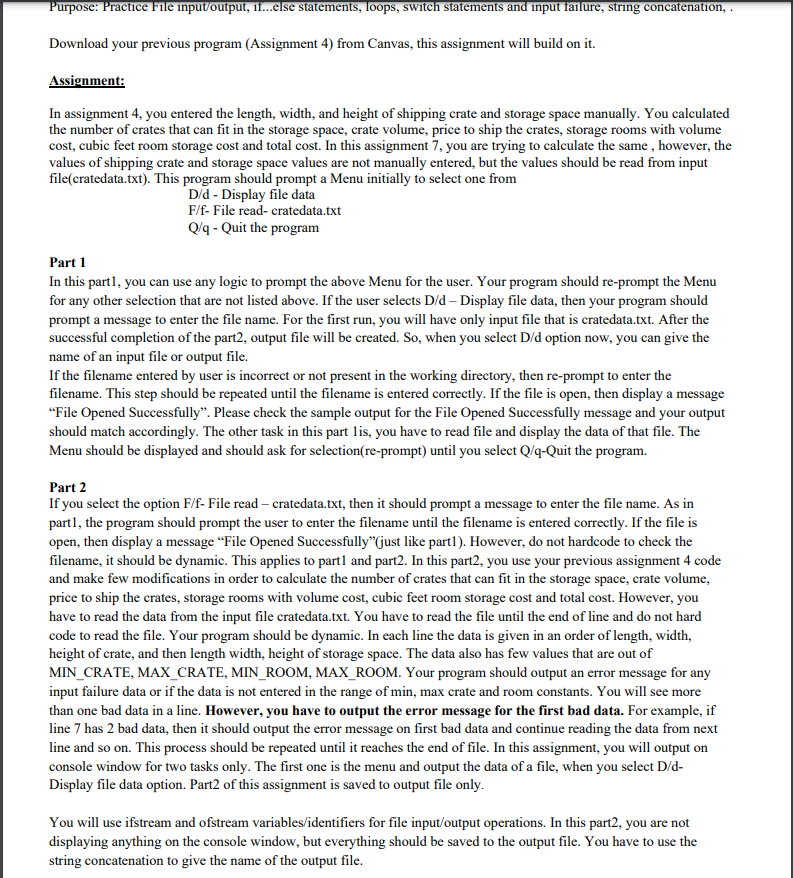
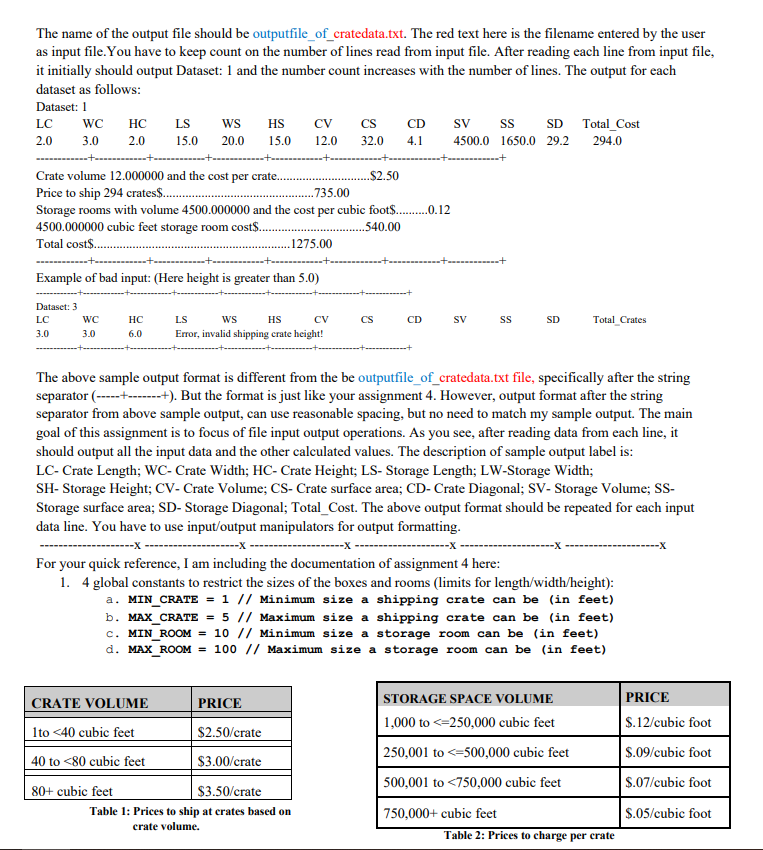
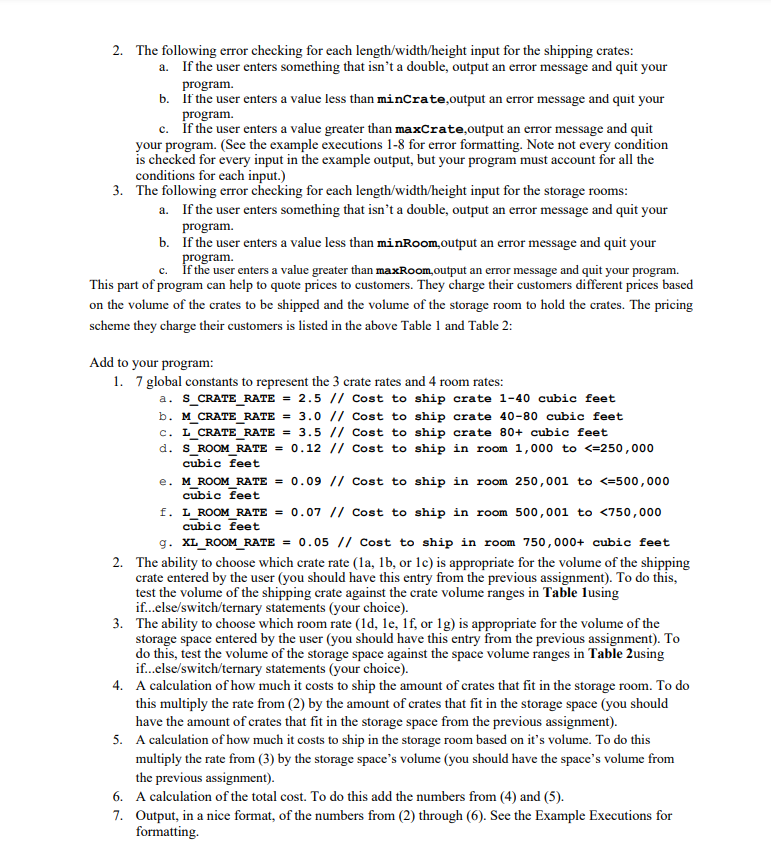
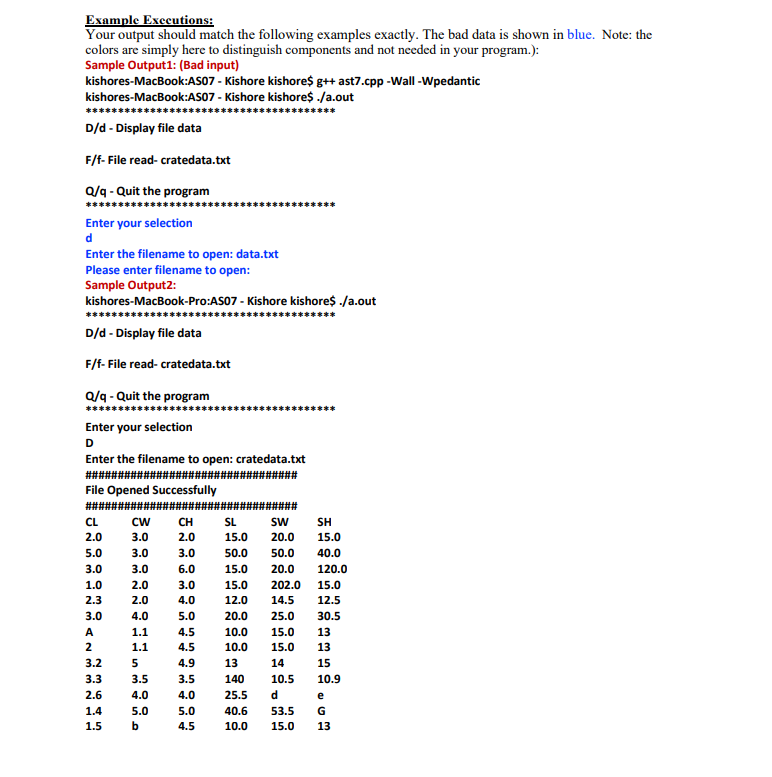
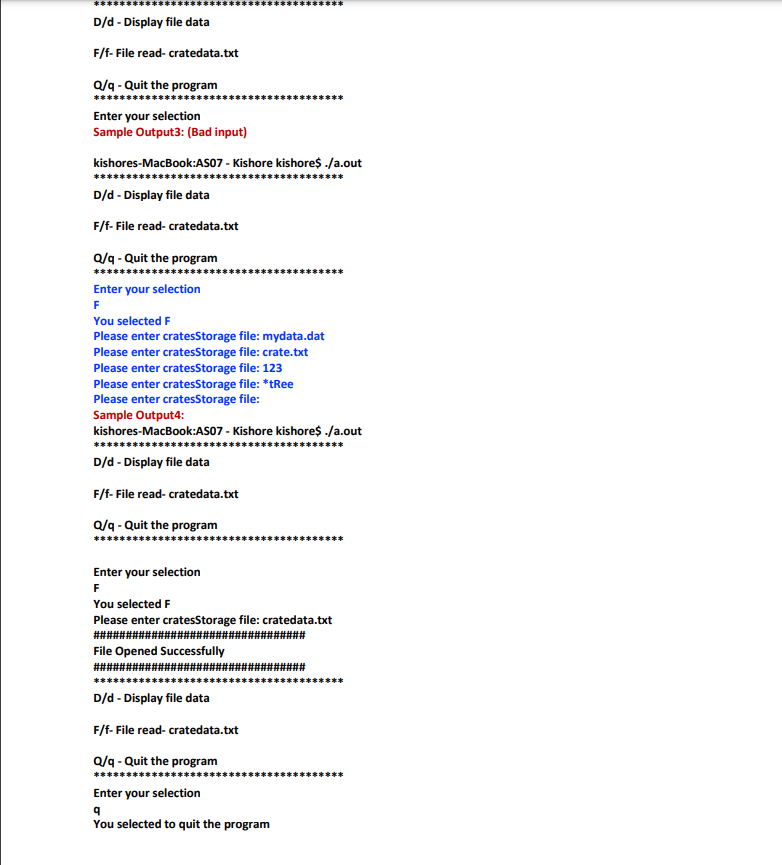
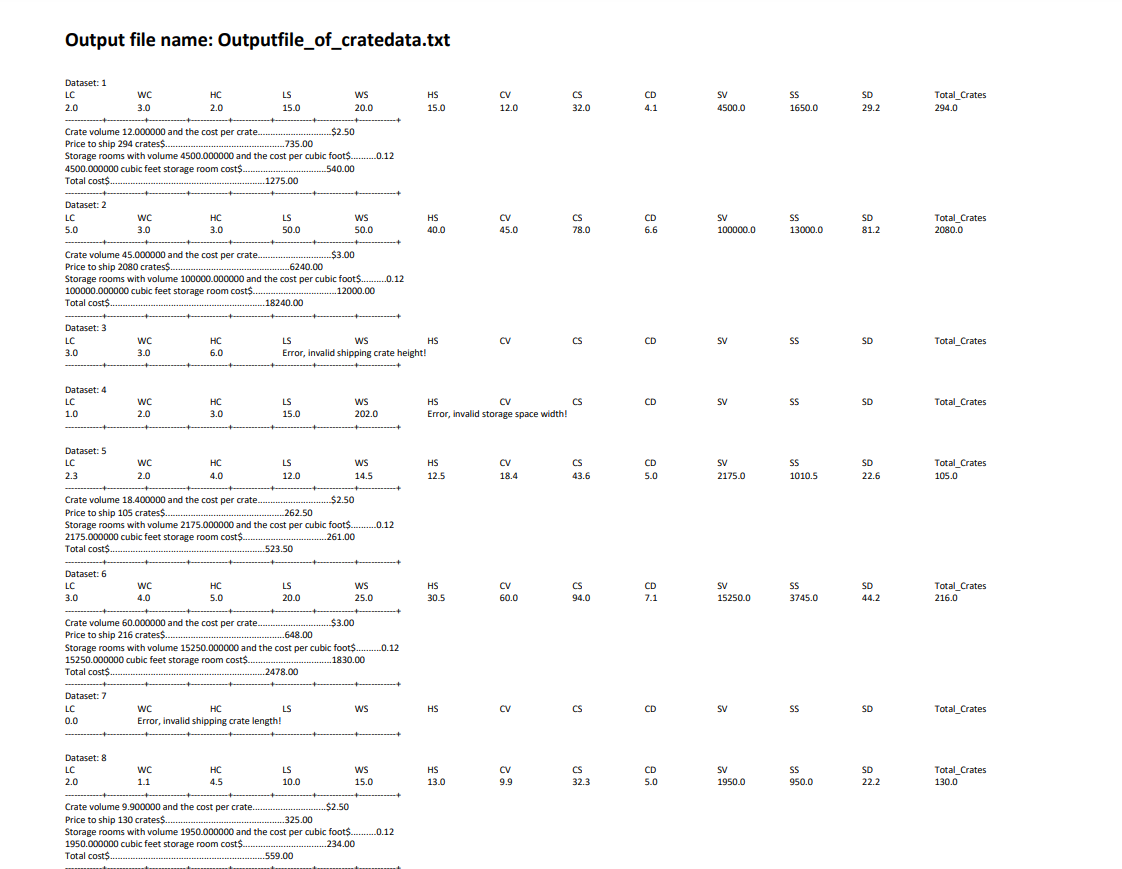
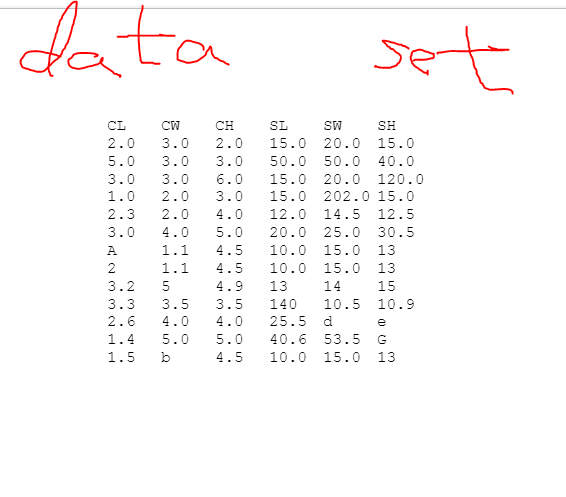
Purpose: Practice File input/output, if...else statements, loops, switch statements and input failure, string concatenation, Download your previous program (Assignment 4) from Canvas, this assignment will build on it. Assignment: In assignment 4, you entered the length, width and height of shipping crate and storage space manually. You calculated the number of crates that can fit in the storage space, crate volume, price to ship the crates, storage rooms with volume cost, cubic feet room storage cost and total cost. In this assignment 7, you are trying to calculate the same , however, the values of shipping crate and storage space values are not manually entered, but the values should be read from input file(cratedata.txt). This program should prompt a Menu initially to select one from D/-Display file data F/f- File read- cratedata.txt Q/q-Quit the program Part 1 In this partl, you can use any logic to prompt the above Menu for the user. Your program should re-prompt the Menu for any other selection that are not listed above. If the user selects D/d - Display file data, then your program should prompt a message to enter the file name. For the first run, you will have only input file that is cratedata.txt. After the successful completion of the part2, output file will be created. So, when you select D/d option now, you can give the name of an input file or output file. If the filename entered by user is incorrect or not present in the working directory, then re-prompt to enter the filename. This step should be repeated until the filename is entered correctly. If the file is open, then display a message File Opened Successfully. Please check the sample output for the File Opened Successfully message and your output should match accordingly. The other task in this part lis, you have to read file and display the data of that file. The Menu should be displayed and should ask for selection(re-prompt) until you select Q/q-Quit the program. Part 2 If you select the option F/f-File read cratedata.txt, then it should prompt a message to enter the file name. As in part1, the program should prompt the user to enter the filename until the filename is entered correctly. If the file is open, then display a message File Opened Successfully(just like partl). However, do not hardcode to check the filename, it should be dynamic. This applies to part1 and part2. In this part2, you use your previous assignment 4 code and make few modifications in order to calculate the number of crates that can fit in the storage space, crate volume, price to ship the crates, storage rooms with volume cost, cubic feet room storage cost and total cost. However, you have to read the data from the input file cratedata.txt. You have to read the file until the end of line and do not hard code to read the file. Your program should be dynamic. In each line the data is given in an order of length, width, height of crate, and then length width, height of storage space. The data also has few values that are out of MIN_CRATE, MAX_CRATE, MIN_ROOM, MAX_ROOM. Your program should output an error message for any input failure data or if the data is not entered in the range of min, max crate and room constants. You will see more than one bad data in a line. However, you have to output the error message for the first bad data. For example, if line 7 has 2 bad data, then it should output the error message on first bad data and continue reading the data from next line and so on. This process should be repeated until it reaches the end of file. In this assignment, you will output on console window for two tasks only. The first one is the menu and output the data of a file, when you select D/d- Display file data option. Part2 of this assignment is saved to output file only. You will use ifstream and ofstream variables/identifiers for file input/output operations. In this part2, you are not displaying anything on the console window, but everything should be saved to the output file. You have to use the string concatenation to give the name of the output file. The name of the output file should be outputfile_of_cratedata.txt. The red text here is the filename entered by the user as input file. You have to keep count on the number of lines read from input file. After reading each line from input file, it initially should output Dataset: 1 and the number count increases with the number of lines. The output for each dataset as follows: Dataset: 1 WC LS ws HS CV CS CD SV SS SD Total Cost 3.0 2.0 15.0 20.0 15.0 12.0 32.0 4500.0 1650.0 29.2 294.0 HC LC 2.0 4.1 Crate volume 12.000000 and the cost per crate. $2.50 Price to ship 294 crates.... .735.00 Storage rooms with volume 4500.000000 and the cost per cubic foot...........0.12 4500.000000 cubic feet storage room cost$.. 540.00 Total cost$..... 1275.00 Example of bad input: (Here height is greater than 5.0) Dataset: 3 LC WC 3.0 3.0 LS WS HS CV CS CD SV SS SD HC 6.0 Total Crates Error, invalid shipping crate height! The above sample output format is different from the be outputfile_of_cratedata.txt file, specifically after the string separator (----+-------+). But the format is just like your assignment 4. However, output format after the string separator from above sample output, can use reasonable spacing, but no need to match my sample output. The main goal of this assignment is to focus of file input output operations. As you see, after reading data from each line, it should output all the input data and the other calculated values. The description of sample output label is: LC-Crate Length; WC- Crate Width; HC-Crate Height; LS- Storage Length; LW-Storage Width; SH- Storage Height; CV-Crate Volume; CS-Crate surface area; CD-Crate Diagonal; SV-Storage Volume; SS- Storage surface area; SD- Storage Diagonal; Total_Cost. The above output format should be repeated for each input data line. You have to use input/output manipulators for output formatting. ----X -X For your quick reference, I am including the documentation of assignment 4 here: 1. 4 global constants to restrict the sizes of the boxes and rooms (limits for length/width/height): a. MIN_CRATE = 1 // Minimum size a shipping crate can be (in feet) b. MAX_CRATE = 5 // Maximum size a shipping crate can be (in feet) C. MIN_ROOM = 10 // Minimum size a storage room can be (in feet) d. MAX_ROOM = 100 // Maximum size a storage room can be (in feet) CRATE VOLUME PRICE Ito