Question
Port the Java assignment to Scheme. Use Dr Racket as your IDE (remember to set the language to R5RS). Do not hard code lengths -
Port the Java assignment to Scheme. Use Dr Racket as your IDE (remember to set the language to R5RS). Do not hard code lengths - I can use any tree that complies with the rules to test your code.
A few "hints":
1) There is something missing from the uploaded slides - comparing strings is not the same as comparing integers:
(= 5 5) is for integers
(equal? "ABC" "ABC") is for strings
2) I mentioned in class that "begin" was bad form. That is true. But it's necessary for this assignment.
3) Remember that the last expression in a scheme function is the return value - there is no explicit "return" keyword.
You can submit with any file extension - text, .rkt, .scm,
Below is the sample tree from the Java assignment:
(define tree '("S" ( ("-" ("A" 3333) ("A" 4444)) ("W" ( ("+" ("R" 0) ("R" 1)) ("+" ("R" 1) ("R" 2)) ("+" ("R" 2) ("R" 3)) ("+" ("R" 3) ("R" 4)) ("+" ("R" 4) ("R" 5))) (("-" ("R" 0) ("R" 1)) ("-" ("R" 1) ("R" 2)) ("-" ("R" 2) ("R" 3)) ("-" ("R" 3) ("R" 4)) ("-" ("A" 1000) ("A" 2000)))))))
--------------------------------------------------------------------------------------
NOTE - the "W" node is a little different than the Java code - there are two lists of nodes, but no "S" indicators on them.
Java Assignment :
import java.util.*; public class Visitor { public static void Visit(Node n) { DumpNode(n,0); } private static String DumpOperand(Operand a) { if (a.GetType() == 'R') return "R" + ((RegisterOperand) a).GetRegister(); else return "$" + ((AddressOperand) a).GetAddress(); } private static int DumpWhile(WhileNode n, int counter) { int tmp = counter; counter = DumpList(n.conditions,counter); System.out.println(counter + ": bne $00000000"); counter++; counter = DumpList(n.statements,counter); System.out.println(counter + ": jmp $" + tmp);counter++; return counter; } private static int DumpTwoOperandNode(TwoOperandNode n, int counter) { String operatorStr; switch (n.GetType()) { case '+' : operatorStr = "add";break; case '-' : operatorStr = "sub";break; case '*' : operatorStr = "mul";break; case '/' : operatorStr = "div";break; default: operatorStr = "ERROR!";break; } System.out.println(counter + ": " + operatorStr + " " + DumpOperand(n.operand1) + "," + DumpOperand(n.operand2)); return counter+1; } private static int DumpNode(Node n, int counter) { if (n.GetType() == 's') return DumpList((StatementsNode)n,counter); if (n.GetType() == 'w') return DumpWhile((WhileNode)n,counter); if ((n.GetType() == '+') || (n.GetType() == '-') || (n.GetType() == '*') || (n.GetType() == '/')) return DumpTwoOperandNode((TwoOperandNode)n,counter); return 0; } private static int DumpList(Queue ns, int counter) { if (ns.isEmpty()) return counter; counter = DumpNode(ns.remove(),counter); return DumpList(ns,counter); } private static int DumpList(StatementsNode sn, int counter) { return DumpList(sn.children,counter); } }
-----
Rewrite the program in scheme using the sample tree given above
Step by Step Solution
There are 3 Steps involved in it
Step: 1
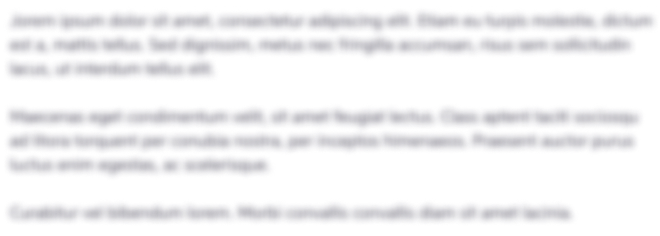
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started