Question
*******************PP3.c #include #include #include #include Student.h #include Course.h #include vector_student.h char GetAction(const char *prompt); void ListStudents(Course course); int main() { char *msg; //this is the
*******************PP3.c
#include
char GetAction(const char *prompt); void ListStudents(Course course);
int main() { char *msg; //this is the actual course record (stored in the activation record of main) //there is only ONE course, so having main keep the struct //works fine. Course course; int probCount; Student studentWithHighestGPA;
InitCourse(&course); //create course struct, see code in Course.c
const char EXIT_CODE = 'X'; const char PROMPT_ACTION[80] = "Add, Probation, List, High GPA, Deans, Remove or eXit (a,p,l,h,d,r,x): "; char userAction; char first[50], last[50]; /ame of student double gpa; //gpa of student // Loop until the user enters the exit code. userAction = GetAction(PROMPT_ACTION); while (userAction != EXIT_CODE) { switch (userAction) { case 'A': printf(" Enter first name: "); fgets(first, 50, stdin); first[strlen(first) - 1] = '\0'; // Remove trailing newline
printf("Enter first name: "); fgets(last, 50, stdin); last[strlen(last) - 1] = '\0'; // Remove trailing newline
printf("Enter gpa: "); scanf("%lf", &gpa); AddStudent(&course, InitStudent(first, last, gpa)); //pass address of course struct break; case 'P': probCount = CountProbation(course); printf("Probation count: %d ", probCount); break; case 'L': ListStudents(course); break; case 'H': studentWithHighestGPA = FindStudentHighestGPA(course); msg = toString(&studentWithHighestGPA); printf("highest gpa: %s", msg); break; case 'D': printf("number of dean list %d", PrintDeansList(course)); break; case 'R': if (CourseSize(course)
// Get the action the user wants to perform char GetAction(const char *prompt) { char answer[50]; char firstChar; firstChar = '?'; printf(" %s ", prompt); fgets(answer, 50, stdin); while (strlen(answer) == 1) { fgets(answer, 50, stdin); } firstChar = toupper(answer[0]); return firstChar; }
*******************Course.c
#include
void InitCourse(Course *course) { course->numStudents = 0; course->roster = (vector *)malloc(sizeof(vector));//this is an address for students vector_create(course->roster, 0); //create some space for vector of studetnts }
//pass THE ADDRESS of the course struct to this functon, because we want to //update values in the course struct IN main activation record void AddStudent(Course *course, Student student) { vector *v = course->roster; //pointer to roster student vector vector_push_back(v, student); //puts the student struct at end of student vector course->numStudents++; //pointer to int numStudents return; }
// List all students void ListStudents(Course course) //pass by value the course struct { int nElements; int i; char *msg; /ote: course.roster is an address to the vector struct of students vector *v = course.roster; //get address of class roster (this is a pointer) nElements = vector_size(course.roster); if (nElements > 0) { printf(" "); for (i = 0; i
int CourseSize(Course course) { return course.numStudents; }
////////////////////////YOU COMPLETE THE FOLLOWING FUNCTIONS//////// int CountProbation(Course course) { int probationCount = 0; Student *s; //loop through students /* Type your code here */ return probationCount; }
Student FindStudentHighestGPA(Course course) { vector *v = course.roster; Student *topStudent = v->elements; //first student address int indexOfTopStudent = 0; //loop through students /* Type your code here */
return ; //fix this to return ONLY the student struct, not a pointer }
// print all Students with a GPA of at least 3.5, and return number of students on dean's list int PrintDeansList(Course course) { int numDeansList = 0; vector *v = course.roster; //pointer to Student struct Student s; //the actual Student struct (not a pointer to it) //loop through students //Type your code here
return numDeansList; }
Course DropStudent(char *studentLastName, Course course) { vector *v = course.roster; //pointer to Student struct Student s; //Type your code here return course; }
**********************Course.h
#ifndef COURSE_H_ #define COURSE_H_
#include "Student.h" #include "vector_student.h"
typedef struct Course_struct { vector *roster; //pointer to vector of Student structs int numStudents; } Course;
void InitCourse(Course *course); void AddStudent(Course *course, Student student); int CourseSize(Course course);
//these are the fucntion you will write //do this in Course.c int CountProbation(Course course); Student FindStudentHighestGPA(Course course) ; int PrintDeansList(Course course); Course DropStudent(char *studentLastName, Course course);
#endif
***************student.c
#include
#include "Student.h"
Student InitStudent(char *first, char *last, double gpa) { Student student; strcpy(student.first, first); strcpy(student.last, last); student.gpa = gpa; return student; }
void GetLastName(char *studentName, Student student) {/ote: last name returned by address in parameter list strcpy(studentName, student.last); }
double GetGPA(Student student) { return student.gpa; }
**************student.h
#ifndef STUDENT_H_ #define STUDENT_H_
typedef struct Student_struct { char first[20]; char last[20]; double gpa; } Student;
Student InitStudent(char *first, char *last, double gpa); void GetLastName(char *studentName, Student student); double GetGPA(Student student);
#endif
**************************student_vector.c
#include
// Initialize vector with specified size void vector_create(vector* v, unsigned int vectorSize) { int i; if (v == NULL) return; v->elements = (Student*)malloc(vectorSize * sizeof(Student)); v->size = vectorSize; for (i = 0; i size; ++i) { strcpy(v->elements[i].first, ""); strcpy(v->elements[i].last, ""); v->elements[i].gpa = 0.0; } }
// Destroy vector void vector_destroy(vector* v) { if (v == NULL) return; free(v->elements); v->elements = NULL; v->size = 0; }
// Resize the size of the vector void vector_resize(vector* v, unsigned int vectorSize) { int oldSize; int i; if (v == NULL) return; oldSize = v->size; v->elements = (Student*)realloc(v->elements, vectorSize * sizeof(Student)); v->size = vectorSize; for (i = oldSize; i size; ++i) { strcpy(v->elements[i].first, ""); strcpy(v->elements[i].last, ""); v->elements[i].gpa = 0.0; } }
// Return pointer to element at specified index Student* vector_at(vector* v, unsigned int index) { if (v == NULL || index >= v->size) return NULL; return &(v->elements[index]); }
// Insert new value at specified index void vector_insert(vector* v, unsigned int index, Student value) { int i; if (v == NULL || index > v->size) return; vector_resize(v, v->size + 1); for (i = v->size - 1; i > index; --i) { v->elements[i] = v->elements[i-1]; } strcpy(v->elements[i].first, value.first); strcpy(v->elements[i].last, value.last); v->elements[i].gpa = value.gpa; }
// Insert new value at end of vector void vector_push_back(vector* v, Student value) { vector_insert(v, v->size, value); }
// Erase (remove) value at specified index void vector_erase(vector* v, unsigned int index) { int i; if (v == NULL || index >= v->size) return; for (i = index; i size - 1; ++i) { v->elements[i] = v->elements[i+1]; } vector_resize(v, v->size - 1); }
// Return number of elements within vector int vector_size(vector* v) { if (v == NULL) return -1; return v->size; }
//pretty print char *toString(Student *s) { char info[100], *result; //why is returning info a mistake? sprintf(info, "First Name: %s \tLast Name: %s \tGPA: %.2lf ", s->first, s->last, s->gpa); result = (char *)malloc(strlen(info) + 1); strcpy(result, info); return result; }
*******************student_vector.h
#ifndef VECTORCOURSE_H #define VECTORCOURSE_H
#include "Student.h"
// struct and typedef declaration for Vector ADT typedef struct vector_struct { Student* elements; //this is a pointer to a Student struct unsigned int size; } vector;
// interface for accessing Vector ADT
// Initialize vector void vector_create(vector* v, unsigned int vectorSize);
// Destroy vector void vector_destroy(vector* v);
// Resize the size of the vector void vector_resize(vector* v, unsigned int vectorSize);
// Return pointer to element at specified index Student* vector_at(vector* v, unsigned int index);
// Insert new value at specified index void vector_insert(vector* v, unsigned int index, Student value);
// Insert new value at end of vector void vector_push_back(vector* v, Student value);
// Erase (remove) value at specified index void vector_erase(vector* v, unsigned int index);
// Return number of elements within vector int vector_size(vector* v);
//pretty print char *toString(Student *s);
#endif
1. int CountProbation(Course course); Student are put on probation if their GPA is below 2.0. Complete Course.c by implementing the CountProbation() function, which print the students that are on probation and returns the number of students on probation. 2. Student FindStudentHighestGPA(Course course); Complete Course.c by implementing the Find StudentHighestGPA() function, which returns the Student (actually the Student struct) with the highest GPA in the course. Assume that no two students have the same highest GPA. 3. int PrintDeansList(Course course); Students make the Dean's list if their GPA is 3.5 or higher. Complete Course.c by implementing the PrintDeansList() function, which prints a list of students with a GPA of 3.5 or higher and also returns the number of students on the dean's list. 4. Course DropStudent(char *studentLastName, Course course); Complete Course.c by implementing the DropStudent() function, which removes a student (by last name) from the course roster. If the student is not found in the course roster, no student should be dropped. HINT: review the functions in vector_student.c very carefullyStep by Step Solution
There are 3 Steps involved in it
Step: 1
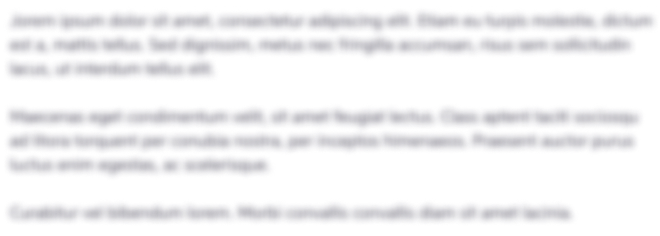
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started