Question
#pragma once #include typedef int ListItem; #define INVALID_LIST_ITEM (-1) // // TODO !!! // /* Use this header to declare your classes. Do the actual
#pragma once
#include
typedef int ListItem; #define INVALID_LIST_ITEM (-1)
// // TODO !!! //
/* Use this header to declare your classes. Do the actual function implementation in Solution.cpp. It's not really necessary but it's a good habit for larger projects. Ideally we'd put each class in its own header and source but these should be pretty simple and short. */
class List { // // TODO !!! //
/* This is the abstract class from which our list implementations will derive. You should add a constructor, destructor, and the following functions: unsigned int GetSize() - returns the size of the list bool AppendItem(ListItem) - adds the passed-in item to the end of the list. returns whether the append succeeded bool PrependItem(ListItem) - adds the passed-in item to the beginning of the list. returns whether the prepend succeeded ListItem GetItemAt(unsigned int) - returns the list item at the passed-in index or INVALID_LIST_ITEM for a bad index void DeleteItemAt(unsigned int) - deletes the list item at the passed-in index void Print() - prints out each item in the list from start to finish. - this is the only function in List with an actual implementation. - It should print out "I'm a list. My size is #", where '#' is the size of the list, followed by a newline. *** Note that your function signatures might require certain other keywords such as const, virtual (hint, hint), etc. *** *** In this and the following classes, make things public, protected, or private as appropriate. *** */ };
class ArrayList { // // TODO !!! //
/* ArrayList derives from List and implements it using a static array. The constructor should take an unsigned int specifying the capacity of the array. AppendItem and PrependItem should fail if the array is full. Print should call the base Print function and then print the contents of the list in the format "\t[0, 1, 2, 3]" ('\t' is the tab character in case there's some other crazy, C++ way to do that and you're not familiar with my terminology). Even though ListItem is typedef'd to be easy to change, you can assume for the print function that it's going to stay int. */ };
class LinkedList { // // TODO !!! //
/* LinkedList derives from List and implements it using a linked list. The constructor takes no arguments. AppendItem and PrependItem really shouldn't fail unless you run out of memory or an asteroid destroys the earth. Print should call the base Print function and then print the contents of the list in the format "\t[0, 1, 2, 3]" ('\t' is the tab character in case there's some other crazy, C++ way to do that and you're not familiar with my terminology). Even though ListItem is typedef'd to be easy to change, you can assume for the print function that it's going to stay int. */ };
--------------------------------------------
#include "Solution.h"
// // TODO !!! //
/* Put your actual implemetation in this file. See Solution.h for details. */
---------------------------------
#include
// // TODO !!! //
/* This is a VERY simple test. Feel free to add more tests if you like but this isn't part of the assignment. I only care about Solution.h and Solution.cpp. */
int main() { ArrayList a1 = ArrayList(10); ArrayList a2 = ArrayList(5); LinkedList l1 = LinkedList();
List *lists[] = { &a1, &a2, &l1, nullptr }; int index = 0; List *list = lists[0]; while(list != nullptr) { printf("GetSize: %d ", list->GetSize());
printf("AppendItem %s ", list->AppendItem(5) ? "succeeded" : "failed"); printf("AppendItem %s ", list->AppendItem(3) ? "succeeded" : "failed"); printf("AppendItem %s ", list->PrependItem(7) ? "succeeded" : "failed"); printf("GetSize: %d ", list->GetSize()); printf("AppendItem %s ", list->AppendItem(0) ? "succeeded" : "failed"); printf("AppendItem %s ", list->PrependItem(6) ? "succeeded" : "failed"); printf("AppendItem %s ", list->AppendItem(9) ? "succeeded" : "failed"); printf("AppendItem %s ", list->PrependItem(8) ? "succeeded" : "failed"); printf("GetSize: %d ", list->GetSize()); printf("GetItemAt: %d ", list->GetItemAt(4)); printf("GetItemAt: %d ", list->GetItemAt(8)); list->Print();
list = lists[++index]; }
printf("press enter to exit "); getc(stdin);
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
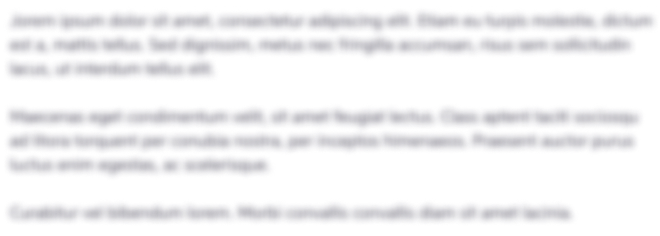
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started