Question
Prime Number Generator Description and Specifications Create a program to list the prime numbers between two numbers supplied in a text file. A number is
Prime Number Generator
Description and Specifications
Create a program to list the prime numbers between two numbers supplied in a text file. A number is considered prime if it is greater than 1 and has no factors between 2 and the square root of the number rounded up, inclusive. Write a Primes.cpp source file and accompanying Primes.h header file that finds all the prime numbers within a range specified and outputs the results to a second text file named primes.txt. Write a driver program Main.cpp that obtains the range from a text file range.txt and then calls the appropriate function to find the prime numbers.
Primes.cpp
Primes.cpp should find all the prime numbers within a specified range and output the results to primes.txt. To accomplish this, you will need to #incluce the cmath library to access the sqrt function and the iostream library for file access functionality.
Primes.h Header files allow you to put some code in a separate file in order to speed up compile time, keep your code more organized, and separate interface from implementation. You must always use an include guard for header files. There is an article on include guards on Wikipedia if you would like more information on this topic. The header file for this program should contain the prototypes for the following functions:
//determines whether given number is prime bool isPrime (int num); //find and write to a file all the prime numbers in the specified range, //calls isPrime to accomplish void findPrimes (int lower, int upper, const char file_name[]);
Main.cpp
A driver program may also be called a testing program. Your driver source code will read in the range from range.txt and then will call the findPrimes function to find and print the prime numbers. Then it will print a confirmation to the screen Prime numbers from x to y have been printed to range.txt where x and y is the range
Compiling with Header files or Multiple Source Files
To compile a program using multiple files, each file must be compiled, then the entire program must be linked into one executible:
g++ -c Primes.cpp g++ -c Main.cpp g++ -o Main.exe Main.o Primes.o
Necessary Input Files
range.txt
Sample Output
Compile and run your program
C:\Users\DocBrown\lab7.exe Prime numbers between 1 and 20 have been printed to primes.txt
Contents of Primes.txt should be:
Below are the primes from 1 to 20 2 3 5 7 11 13 17 19
Step by Step Solution
There are 3 Steps involved in it
Step: 1
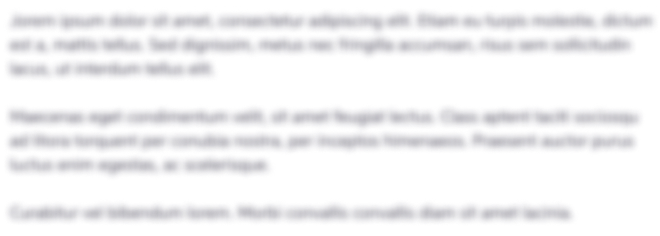
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started