Question
Printing a string backwards can be done iteratively or recursively. To do it recursively, think of the following specification: If s contains any characters (i.e.,
Printing a string backwards can be done iteratively or recursively. To do it recursively, think of the following specification:
If s contains any characters (i.e., is not the empty string)
- print the last character in s
- print s' backwards, where s' is s without its last character
File Backwards.java contains a program that prompts the user for a string, then calls method printBackwards to print the string backwards. Save this file to your directory and fill in the code for printBackwards using the recursive strategy outlined above.
here is backwards that we were given to work with
// ****************************************************************** // Backwards.java // // Uses a recursive method to print a string backwards. // ****************************************************************** import java.util.Scanner;
public class Backwards { //-------------------------------------------------------------- // Reads a string from the user and prints it backwards. //-------------------------------------------------------------- public static void main(String[] args) { Scanner scan = new Scanner(System.in); String msg;
System.out.print("Enter a string: "); msg = scan.next(); System.out.print(" The string backwards: "); printBackwards(msg); System.out.println(); } //-------------------------------------------------------------- // Takes a string and recursively prints it backwards. //-------------------------------------------------------------- public static void printBackwards(String s) {
// Fill in code
} }
please use beginners level skills we are only on the recursion chapter of computer science and I would be able to understand the code. if you have any questions please ask
Step by Step Solution
There are 3 Steps involved in it
Step: 1
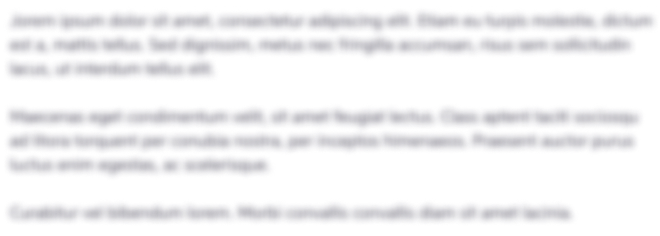
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started