Question
Priority Queue Lab Write a Java class called Game to represent a video game. The Game object should have three instance variables: two String objects
Priority Queue Lab
Write a Java class called Game to represent a video game. The Game object should have three instance variables: two String objects named name and console, and a Date object named date. These instance variables are to have private access modifiers. You will need to write the appropriate getter and setter methods to allow another class or object to access and modify the data values. Game should have a parameterized constructor which takes a String parameter: aName, a Date parameter: aDate, and a String parameter: aConsole (in that order). In accordance with good programming practice, you are to override the toString method inherited from Object. The toString method should return in the following format:
Name-delim-Date-delim-Console
When using the date instance variable in the toString method, use Dates getTime() method to get dates value.
Write a Java class called Lab5. Lab5 has no instance variables and contains only a single method: sortGames which reads an input file containing text. Use a Scanner object to read the contents of the input file. sortGames takes a File parameter: anInFile, a File parameter: anOutFile, and a SortBy parameter: aSort (in that order). (When importing the Date class, make sure to import java.util.Date)The given text file will contain game information, where each line of the file contains the information for a single game. The properties of each game will be separated by a specific string of characters -delim-. For example, a line of text would look like:
Final Fantasy XI: Rise of the Zilart-delim-1073000981498-delim-PS2
Where the first part of the String is the name of the game, the second the date the game was released, and the third the console the game was released for.
The sortGames method should read the contents of anInFile line by line, making each line into Game object. It should then create a PQEntry object where the value is the created Game object and the key is one of the Game objects instance variables. The created PQEntry object should then be placed in a Priority Queue object. To make a line of text into a Game object, utilize the following two methods:
split, which is a method in the String class. split takes a single String parameter and splits the String object from which it was called into a String array, using the given String parameter to determine where to break apart the host String
The Date constructor, which takes a long variable and creates a date which represents the number of milliseconds since the standard base time known as "the epoch", namely January 1, 1970, 00:00:00 GMT.
After parsing the entire input file, use a PrintWriter object to write the String value of each Game object in the Priority Queue to anOutFile.
Lastly, SortBy is an enumerated type. An enumerated type is a special data type that enables for a variable to be a set of predefined constants. The variable must be equal to one of the values that have been predefined for it. The SortBy enumerated type contains three values: NAME,DATE,CONSOLE. A variable of type SortBy can only be one of those three values. To define a new enumerated type variable, use:
SortBy toSort = SortBy.NAME;
To compare enumerated types, use the == operator. For example:
if(toSort == SortBy.NAME){}
would evaluate to true. In sortGames, use the given enumerated type to determine which of Games instance variables should be the Key used for PQEntry. If toSort is equal to SortBy.NAME, then when creating a PQEntry object, the key should be the Game objects name instance variable and the value should be the Game object itself.
NOTE: To implement this method, you may find yourself defining a Priority Queue before initializing it. Because you may initialize the defined Priority Queue with different data types depending on the value of the SortBy parameter, you wont be able to specify the data types of the Priority Queue when you define it. This will cause a compiler warning which we can safely ignore.
NOTE TWO: You will need to create Comparator objects to be used when initializing new priority queues. Use the Comparator classes on Blackboard as templates.
When you have completed your implementation of both the Game and the Lab5 classes, use the provided test cases to test your work. When complete, submit your Game and the Lab5 classes to Blackbaord. I advise implementing and testing your Game class before starting your Lab5 class. The test cases for Lab5 will not pass if you do not have a working Game class. If you create a testing class for your own use, you dont need to submit that.
{Given}
package providedClasses;
public interface Entry
K getKey();
V getValue();
}
{Given}
public class PQEntryimplements Entry {
private K key;
private V value;
public PQEntry(K aKey, V aValue) {
key = aKey;
value = aValue;
}
@Override
public K getKey() {
return key;
}
@Override
public V getValue() {
return value;
}
@Override
public String toString() {
return "K: " + key.toString() + " V: " + value.toString();
{GIVEN}
package providedClasses;
import java.util.Comparator;
public class PriorityQueue{
Queue> queue;
Comparatorcomparator;
public PriorityQueue(ComparatoraComparator) {
queue = new Queue>();
comparator = aComparator;
}
public void insert(PQEntryanEntry) {
if(queue.size() == 0) {
queue.enqueue(anEntry);
}
else {
boolean isAdded = false;
for(int i = queue.size(); i > 0; i--) {
PQEntrytempEntry = queue.dequeue();
//If tempEntry key > anEntry key --> evaluates to true
if(comparator.compare(tempEntry.getKey(), anEntry.getKey()) > 0 && isAdded == false) {
queue.enqueue(anEntry);
queue.enqueue(tempEntry);
isAdded = true;
}
else {
queue.enqueue(tempEntry);
}
}
if(isAdded == false) {
queue.enqueue(anEntry);
}
}
}
public PQEntryremove(){
return queue.dequeue();
}
public PQEntrymin(){
return queue.first();
}
public int size() {
return queue.size();
}
public boolean isEmpty() {
return queue.isEmpty();
}
public String toString() {
return queue.toString();
}
//First In First Out FIFO
public class Queue{
private SinglyLinkedListlinkedList;
public Queue() {
linkedList = new SinglyLinkedList();
}
public int size() {
return linkedList.getSize();
}
public boolean isEmpty() {
return linkedList.isEmpty();
}
public E first() {
if(isEmpty() == true) {
return null;
}
else {
return linkedList.getFirst();
}
}
public E dequeue() {
if(isEmpty() == true) {
return null;
}
else {
return linkedList.removeFirst();
}
}
public void enqueue(E anElement) {
linkedList.addLast(anElement);
}
public String toString() {
return linkedList.toString();
}
@SuppressWarnings("hiding")
public class SinglyLinkedList{
//Instance Variables
private Nodehead;
private Nodetail;
private int size;
//Default Constructor
public SinglyLinkedList() {
size = 0;
}
//Getter methods
public int getSize() {
return size;
}
public boolean isEmpty() {
boolean toReturn = false;
if(size == 0) {
toReturn = true;
}
return toReturn;
}
public E getFirst() {
if(isEmpty() == true) {
return null;
}
else {
return head.getElement();
}
}
public E getLast() {
if(isEmpty() == true) {
return null;
}
else {
return tail.getElement();
}
}
public String toString() {
String result = "";
NodeaNode = head;
if(aNode != null) {
result = aNode.toString() + " , " + result;
while(aNode.getNextNode() != null) {
aNode = aNode.getNextNode();
result = aNode.toString() + " , " + result;
}
}
result = "[" + result + "]";
return result;
}
//Setter methods
public void addLast(E anElement) {
//Create a new node with the given element. Because this is the last node in the linked list, we set the value of the new nodes next node
//to null, as there is no next node
NodenewNode = new Node (anElement,null);
//If the linked list is empty we make the new node the head node because it will be both the head and the tail node.
if(isEmpty() == true) {
head = newNode;
}
else {
//If the linked list has nodes in it already, we link the new node to the current tail of our linked list
tail.setNextNode(newNode);
}
//We update the tail and size instance variables
tail = newNode;
size++;
}
public E removeFirst() {
if(isEmpty() == true) {
return null;
}
else {
E toReturn = head.getElement();
head = head.getNextNode();
size--;
if(size == 0) {
tail = head;
}
return toReturn;
}
}
}
public class Node{
//Instance Variables
private G element;
private Nodenext;
//Parameterized Constructor
public Node(G anElement, NodenextNode) {
element = anElement;
next = nextNode;
}
//Getters and setters
public G getElement() {
return element;
}
public void setElement(G anElement) {
element = anElement;
}
public NodegetNextNode(){
return next;
}
public void setNextNode(NodenextNode) {
next = nextNode;
}
public String toString() {
return element.toString();
}
}
}
}
GIVEN
public enum SortBy{
NAME,DATE,CONSOLE
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
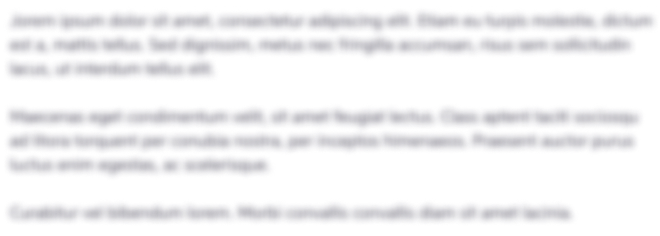
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started