Question
Problem 1 You are given four java source files: a class Question (see Question.java), a subclass ChoiceQuestion (see ChoiceQuestion.java) and two testing programs (see QuestionDemo1.java
Problem 1 You are given four java source files: a class Question (see Question.java), a subclass ChoiceQuestion (see ChoiceQuestion.java) and two testing programs (see QuestionDemo1.java and QuestionDemo2.java). Answer all questions based on the following inheritance hierarchy of Question types:
super class: Question
Sub classes: FillInQuestion, ChoiceQuestion, NumericQuestin, FreeResponseQuestion
Subclass of choiceQuestion: MultiChoiceQuestion
(a) Add a class NumericQuestion to the question. In this class, you need to define its own private member variable answer, but its type should be double. In inheritance, member variables cannot be overridden like member methods. The answer member variable in the NumericQuestion subclass is hiding the member variable answer in the Question superclass. You need to override the checkAnswer method based on the following logic: if the response and the expected answer differ by no more than 0.01, accept the response as correct. (b) Modify the checkAnswer method of the Question class so that it does not take into account different spaces or upper/lowercase characters. For example, the response "JAMES gosling" should match an answer of "James Gosling". (c) Add a method addText to the Question superclass to add a provided String type argument to the question text. Modify addChoice method in the ChoiceQuestion class that calls addText in the superclass rather than storing an array list of choices. The member variable choices in the sub class ChoiceQuestion need to be removed. (d) Provide toString methods for the Question and ChoiceQuestion classes to replace their display methods. This is a much better implementation since toString method can be called automatically when the contents of the objects of these two classes are needed.
** A question with a text and an answer. */ public class Question { private String text; private String answer; /** Constructs a question with empty question and answer. */ public Question() { text = ""; answer = ""; } /** Sets the question text. @param questionText the text of this question */ public void setText(String questionText) { text = questionText; } /** Sets the answer for this question. @param correctResponse the answer */ public void setAnswer(String correctResponse) { answer = correctResponse; } /** Checks a given response for correctness. @param response the response to check @return true if the response was correct, false otherwise */ public boolean checkAnswer(String response) { return response.equals(answer); } /** Displays this question. */ public void display() { System.out.println(text); } }
/** A question with multiple choices. */ public class ChoiceQuestion extends Question { private ArrayListchoices; /** Constructs a choice question with no choices. */ public ChoiceQuestion() { choices = new ArrayList (); } /** Adds an answer choice to this question. @param choice the choice to add @param correct true if this is the correct choice, false otherwise */ public void addChoice(String choice, boolean correct) { choices.add(choice); if (correct) { // Convert choices.size() to string String choiceString = "" + choices.size(); setAnswer(choiceString); } } public void display() { // Display the question text super.display(); // Display the answer choices for (int i = 0; i < choices.size(); i++) { int choiceNumber = i + 1; System.out.println(choiceNumber + ": " + choices.get(i)); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
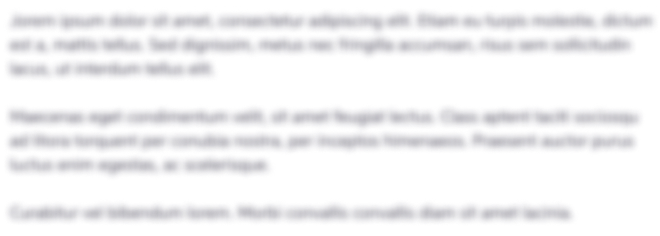
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started