Question
Problem 2 Deliverables: problem2functions.h and problem2functions.cc Functions Test all of your code on a Linux lab computer. All source files submitted must compile and run
Problem 2 Deliverables: problem2functions.h and problem2functions.cc Functions
Test all of your code on a Linux lab computer. All source files submitted must compile and run on a Linux lab computer of the instructors choice. Submissions that do not compile on the Linux workstation will receive no compilation or execution/correctness points.
You should submit only the following individual files to the assignment: problem2funcitons.h, problem2functions.cc
Write a function named Distance that takes four double arguments for the x and y coordinates of two points (x1, y1, x2, y2), and returns the distance between those points as a double. If the function is called with the coordinates of one point only, the second points coordinates should default to the origin (0, 0).
Recall, the formula to compute the distance, d, between two points is:
Example function calls:
Distance(3, 4) should return 5 (the distance between the point (3, 4) and the point (0, 0))
Distance(-1, 3, 11, -2) should return 13 (the distance between the point (-1, 3) and the point (11, -2))
Write a function named OnCircle that takes the x and y coordinates of the center of a circle as the first two parameters, the radius of the circle as the third parameter, and the x and y coordinates of a point as the fourth and fifth parameters (all doubles). The function should return -1 if the point lies inside of the circle, 0 if the point lies on the circle, and 1 if the point lies outside of the circle.
Example calls:
OnCircle(0, 0, 5, 1, 1) should return -1 because the point (1, 1) lies inside the circle centered at (0, 0) with radius 5
OnCircle(0, 0, 5, 3, 4) should return 0 because the point (3, 4) lies on the circle centered at (0, 0) with radius 5
OnCircle(0, 0, 5, 3, 5) should return 1 because the point (3, 5) lies outside the circle centered at (0, 0) with radius 5
Write a function named OnLine that takes the x and y coordinates (all doubles) of three points (x1, y1, x2, y2, x3, y3). The function should return true if the three points lie on a straight line, and false if they do not.
Example calls:
OnLine(0, 0, 1, 3, 2, 6) should return true because the three points (0, 0) (1, 3) and (2, 6) lie on a straight line
OnLine(2, 3.2, -1, 4.2, 8, 1.2) should return true because the three points (2, 3.2) (-1, 4.2) and (8, 1.2)
lie on a straight line
OnLine(-1.8, -1.5, 3, 1, -3.1, -7) should return false because the three points (-1.8, -1.5) (3, 1) and
(-3.1 -7) do not lie on a straight line
Specifications:
All three function prototypes should be included in problem2functions.h
All three functions should be implemented in problem2functions.cc
Initial Testing:
A makefile and some minimal unit tests have been included in problem2tests.zip. You are encouraged to create more rigorous tests. To run the tests provided, create a directory containing only your problem2functions.h file, your problem2functions.cc file, and the files extracted from the attached problem2tests.zip. Then type:
make testDistance make testOnCircle make testOnLine
Points: style: 1 point clean compilation: 1 point Distance passes instructors unit tests: 1 point OnCircle passes instructors unit tests: 1 point OnLine passes instructors unit tests: 2 points
makefile
# makefile for problem 2
#
# $@ target
# $< first prerequisite
# $^ all prerequisites
flags = -std=c++17 -Wall -I .
problem2functions.o : problem2functions.cc problem2functions.h
g++ $(flags) -c $<
testDistance : testDistance.cc problem2functions.o
g++ $(flags) $^ -o $@
./$@
testOnCircle : testOnCircle.cc problem2functions.o
g++ $(flags) $^ -o $@
./$@
testOnLine : testOnLine.cc problem2functions.o
g++ $(flags) $^ -o $@
./$@
clean :
rm problem2functions.o testDistance testOnCircle testOnLine
testDistance.cc
// minimal tests of the Distance function
#include
using std::cout;
using std::endl;
#include"problem2functions.h"
int main() {
if ( Distance(3, 4) == 5 )
cout << "Passed Distance(3, 4) test" << endl;
else
cout << "Failed Distance(3, 4) test, function returned "
<< Distance(3, 4) << ". Expected 5" << endl;
if ( Distance(0.5, -1.2, 0.5, 1.8) == 3 )
cout << "Passed Distance(1, -1, 3, 2) test" << endl;
else
cout << "Failed Distance(0.5, -1.2, 0.5, 1.8) test, function returned "
<< Distance(0.5, -1.2, 0.5, 1.8) << ". Expected 3" << endl;
if ( Distance(-7.1, 2, -1, 2) == 6.1 )
cout << "Passed Distance(-7, 2, -1, 2) test" << endl;
else
cout << "Failed Distance(-7.1, 2, -1, 2) test, function returned "
<< Distance(-7.1, 2, -1, 2) << ". Expected 6.1" << endl;
if ( Distance(3, 8, 3, 8) == 0 )
cout << "Passed Distance(3, 8, 3, 8) test" << endl;
else
cout << "Failed Distance(3, 8, 3, 8) test, function returned "
<< Distance(3, 8, 3, 8) << ". Expected 0" << endl;
if ( Distance(0.5, 2.1, -11.5, 7.1) == 13 )
cout << "Passed Distance(0.5, 2.1, -11.5, 7.1) test" << endl;
else
cout << "Failed Distance(0.5, 2.1, -11.5, 7.1) test, function returned "
<< Distance(0.5, 2.1, -11.5, 7.1) << ". Expected 13" << endl;
return 0;
}
testOnCircle.cc
// limited tests for the OnCircle function
#include
using std::cout;
using std::endl;
#include"problem2functions.h"
int main() {
if ( OnCircle(0, 0, 3, -3, 0) == 0 )
cout << "Passed OnCircle(0, 0, 3, -3, 0) test" << endl;
else
cout << "Failed OnCircle(0, 0, 3, -3, 0) test" << endl;
if ( OnCircle(0.73, -5.2, 5, 3.73, -1.2) == 0 )
cout << "Passed OnCircle(0.73, -5.2, 5, 3.73, -1.2) test" << endl;
else
cout << "Failed OnCircle(0.73, -5.2, 5, 3.73, -1.2) test" << endl;
if ( OnCircle(-8.1, 2.7, 13, 4, 7.7) == 1 )
cout << "Passed OnCircle(-8.1, 2.7, 13, 4, 7.7) test" << endl;
else
cout << "Failed OnCircle(-8.1, 2.7, 13, 4, 7.7) test" << endl;
if ( OnCircle(-8.1, 2.7, 13, -20, 7.7) == -1 )
cout << "Passed OnCircle(-8.1, 2.7, 13, -20, 7.7) test" << endl;
else
cout << "Failed OnCircle(-8.1, 2.7, 13, -20, 7.7) test" << endl;
return 0;
}
testOnLine.cc
// limited tests for the OnLine function
#include
using std::cout;
using std::endl;
#include"problem2functions.h"
int main() {
if ( OnLine(0, 0, 3, -3, 9, -9) )
cout << "Passed OnLine(0, 0, 3, -3, 9, -9) test" << endl;
else
cout << "Failed OnLine(0, 0, 3, -3, 9, -9) test" << endl;
if ( OnLine(2, 3.2, -1, 4.2, 8, 1.2) )
cout << "Passed OnLine(2, 3.2, -1, 4.2, 8, 1.2) test" << endl;
else
cout << "Failed OnLine(2, 3.2, -1, 4.2, 8, 1.2) test" << endl;
if ( OnLine(-1.8, -1.5, 3, 1, -3.1, -7) )
cout << "Failed OnLine(-1.8, -1.5, 3, 1, -3.1, -7) test" << endl;
else
cout << "Passed OnLine(-1.8, -1.5, 3, 1, -3.1, -7) test" << endl;
if ( OnLine(5.12, -12, 5.12, -3.6, 5.12, 14.22) )
cout << "Passed OnLine(5.12, -12, 5.12, -3.6, 5.12, 14.22) test" << endl;
else
cout << "Failed OnLine(5.12, -12, 5.12, -3.6, 5.12, 14.22) test" << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
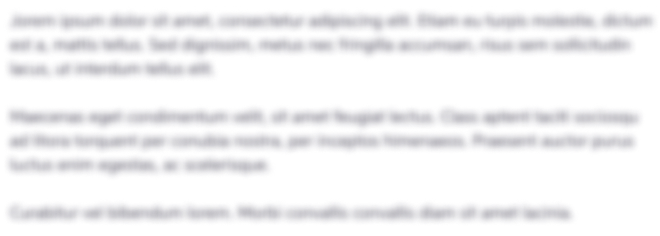
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started