Question
Problem 2: Removing elements from an array In this problem, youll complete another assembly program, found in hw2_remove.s, where you will remove the elements in
Problem 2: Removing elements from an array In this problem, youll complete another assembly program, found in hw2_remove.s, where you will remove the elements in an array of 4-byte integers. This program takes command line arguments for an array. Example 1 command line arguments: 6 2 9 4 beware of the restrictive formatting: (single-digit positive, decimal integers) Starting state for the example register $s0 = 4 (the number of elements in the array) register $s1 = 0x10040000 (address of the start of the array) register $s2 = 2 (The index of the element that we want to remove) Input array (stored in memory) Example: 0x10040000 6 0x10040004 2 0x10040008 9 0x1004000C 4 At the end of your program, the array should remove element of index 2 by moving everything else moved up. 0x10040000 6 0x10040004 2 0x10040008 4 0x1004000C Example 2 command line arguments: 2 6 3 0 0 3 6 2 beware of the restrictive formatting: (single-digit positive, decimal integers) Starting state for the example register $s0 = 8 (the number of elements in the array) register $s1 = 0x10040000 (address of the start of the array) Input array (stored in memory) Example: 0x10040000 2 0x10040004 6 0x10040008 3 0x1004000C 0 0x10040010 0 0x10040014 3 0x10040018 6 0x1004001C 2 At the end of your program, the array should remove element of index 2 by moving everything else up. 0x10040000 2 0x10040004 6 0x10040008 0 0x1004000C 0 0x10040010 3 0x10040014 6 0x10040018 2 0x1004001C
/*******************************MIPS Assembly Program*********************************************************************/
.data
error_string: .asciiz "ERROR: invalid arguments "
.text
main:
# Do not modify this code. It is responsible for
# command line parsing. See further below.
blt $a0,1,error
move $s0, $a0
addiu $t9, $s0, 0
addiu $t7, $s0, 1
sll $t8, $t7, 2
li $v0, 9
move $a0, $t8 # sbrk 4*(num+1)
syscall
move $s1, $v0
addu $s2, $s1, $t8
move $s3, $s1
charloop1:
lw $t0, 0($a1)
lb $t1, 0($t0)
addiu $t1,$t1,-48
sw $t1, 0($s3)
addiu $s3, $s3, 4
addiu $t9, $t9, -1
addiu $a1, $a1, 4
bgt $t9, $zero, charloop1
# $s0 contains the number of elements in the array (the elements are 4-byte integers)
# $s1 contains the address of the array of integers
# Your job is to remove the element at index 2 of the array.
#
# The array is fixed in memory, and we want to remove the element of index 2(starting from 0), assuming array length > 2.
# You must move all subsequent elements left to fill in the hole.
#
# E.g. (here the value in $s0 would be 3, the value in $1 is the address of the first byte of the array)
# values in the array before:
# [1, 2, 3, 4]
#
# values in the array after:
# [1,2,4]
# We do not care what value remains in memory at the last word of the array
# YOUR SOLUTION BELOW
# YOUR SOLUTION ABOVE
exit:
# exit cleanly (these two lines of code are like calling System.exit(0) in Java, exit(0) in C, exit() in Python)
li $v0, 10
syscall
# subroutine for printed an error message
error:
la $a0, error_string
li $v0, 4 # print
syscall
j exit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
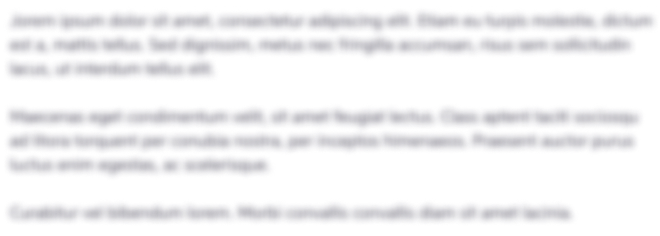
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started