problem 5.test.js const { normalizeCoord } = require('./solutions'); /** * * 1. 43.7955,-79.3496 NOTE: no space * 4. [-79.3496, 43.7955] NOTE: the space, as well
problem 5.test.js
const { normalizeCoord } = require('./solutions');
/**
* * 1. "43.7955,-79.3496" NOTE: no space
* 4. "[-79.3496, 43.7955]" NOTE: the space, as well as lat/lng values are reversed
*/
describe('Problem 5 - normalizeCoord() function', function () {
test('valid lat, lng coord returns proper format', function () {
let coord = '43.7955,-79.3496';
expect(normalizeCoord(coord)).toBe('(43.7955, -79.3496)');
});
describe('lat validation', function () {
test('coord with lat at 90 returns proper format', function () {
let coord = '90,-79.3496';
expect(normalizeCoord(coord)).toBe('(90, -79.3496)');
});
test('coord with lat at -90 returns proper format', function () {
let coord = '-90,-79.3496';
expect(normalizeCoord(coord)).toBe('(-90, -79.3496)');
});
test('coord with invalid lat < -90 throws', function () {
let coord = '-90.01,-79.3496';
expect(() => normalizeCoord(coord)).toThrow();
});
test('coord with invalid lat > 90 throws', function () {
let coord = '90.01,-79.3496';
expect(() => normalizeCoord(coord)).toThrow();
});
});
describe('lng validation', function () {
test('coord with valid lng at 180 returns proper format', function () {
let coord = '43.7955,180';
expect(normalizeCoord(coord)).toBe('(43.7955, 180)');
});
test('coord with valid lng at -180 returns proper format', function () {
let coord = '43.7955,-180';
expect(normalizeCoord(coord)).toBe('(43.7955, -180)');
});
test('coord with invalid lng < -180 throws', function () {
let coord = '43.7955,-180.00001';
expect(() => normalizeCoord(coord)).toThrow();
});
test('coord with invalid lng > 180 throws', function () {
let coord = '43.7955,180.00001';
expect(() => normalizeCoord(coord)).toThrow();
});
});
describe('alternate [lat, lng] format', function () {
test('coord with alternate layout format can be parsed correctly', function () {
let coord = '[-79.3496, 43.7955]';
expect(normalizeCoord(coord)).toBe('(43.7955, -79.3496)');
});
test('coord with alternate layout validates lng correctly', function () {
let coord = '[-181, 43.7955]';
expect(() => normalizeCoord(coord)).toThrow();
});
test('coord with alternate layout validates lat correctly', function () {
let coord = '[-79.3496, 91]';
expect(() => normalizeCoord(coord)).toThrow();
});
});
});
soloution.js
/*******************************************************************************
* Problem 5: parse a geographic coordinate
*
* Coordinates are defined as numeric, decimal values of Longitude and Latitude.
* A example, the Seneca College Newnham campus is located at:
*
* Longitude: -79.3496 (negative number means West)
* Latitude: 43.7955 (positive number means North)
*
* A dataset includes thousands of geographic coordinates, stored as strings.
* However, over the years, different authors have used slightly different formats.
* All of the following are valid and need to be parsed:
*
* 1. "43.7955,-79.3496" NOTE: no space
* 4. "[-79.3496, 43.7955]" NOTE: the space, as well as lat/lng values are reversed
*
* Valid Longitude values are decimal numbers between -180 and 180.
*
* Valid Latitude values are decimal numbers between -90 and 90.
*
* Parse the value and reformat it into the form: "(lat, lng)"
*
* @param {string} value - a geographic coordinate string in one of the given forms
* @returns {string} - a geographic coordinate formatted as "(lat, lng)"
******************************************************************************/
function normalizeCoord(value) {
//write the code here
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
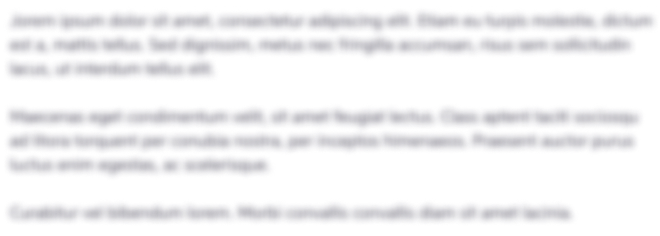
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started