Question
Problem 6: Battleship Blueprint class for Ship objects 10 points total; pair-optional This is one of only two problems in this assignment that you may
Problem 6: Battleship Blueprint class for Ship objects 10 points total; pair-optional
This is one of only two problems in this assignment that you may complete with a partner. See the rules for working with a partner on pair-optional problems for details about how this type of collaboration must be structured.
In the next two problems, you will create blueprint classes for objects that could be used as part of an implementation of the popular game of Battleship. In next weeks problem set, you will complete the implementation of the game!
Rules of the game In our version of Battleship, each player has an 8 x 8 board containing a collection of five ships:
one battleship, of length 4 one cruiser, of length 3 one tanker, of length 3 two patrol boats, each of length 2 The ships are positioned either vertically or horizontally. For example, here is a possible configuration of the board belonging to one of the players:
0 1 2 3 4 5 6 7 0: 1: P 2: P 3: C C C 4: B B B B 5: 6: T T T P P 7: where the B characters represent the battleship, the C characters represent the cruiser, the T characters represent the tanker, and the P characters represent the patrol boats.
The players take turns guessing the locations of their opponents ships by specifying the coordinates (row and column) of a position on their opponents board. If they guess a position occupied by a ship, the position is marked as a hit using the character X. If they guess a position that is not occupied by a ship, the position is marked as a miss using the character -. For example, here is what the board shown above might look like after two hits and three misses:
0 1 2 3 4 5 6 7 0: 1: - P 2: P - 3: C X C 4: B B B X 5: - 6: T T T P P 7: Note that both the battleship and the cruiser have been hit.
If a player hits all of the positions occupied by a given ship, the ship is considered sunk. (Later, we will see that tankers can be sunk more easily!) The winner is the player who is the first to sink all of his opponents ships.
The code for our Battleship program will be divided into a number of different classes, some of which we will provide. You will write one class for this problem and one for Problem 7, and the remainder of the program will be completed next week in Problem Set 3.
The Ship class In this problem, you will create a class called Ship that serves as a blueprint for objects that represent a single ship. The sections below outline the steps that you should take.
(0 points) Create an appropriate class header, and save the class in a file named Ship.java.
Define the fields (2 points) Each Ship object should encapsulate three pieces of state:
the type of ship (a String e.g., Battleship or Cruiser) the length of the ship (i.e., the number of positions that it occupies on the board, stored as an integer) the number of times that the ship has been hit (also an integer). This will be 0 when the ship is first created, and it will increase whenever the ship is hit. For example, here is what a Ship object representing a Cruiser would initially look like in memory:
+------------------+ | +-----+ | +-----------+ | type | ---|--------->| "Cruiser" | | +-----+ | +-----------+ | +-----+ | | length | 3 | | | +-----+ | | +-----+ | | numHits | 0 | | | +-----+ | +------------------+ For now, you only need to define the fields. Make sure to:
use the field names shown above
protect them from direct access by client code.
In the next section, you will write a constructor that assigns values to the fields, and that ensures that only valid values are allowed.
Implement a constructor (2 points) Next, add a constructor that takes two parameters: a string for the ships type, and an integer for the ships length. If the value passed in for the type is null or an empty string, an IllegalArgumentException should be thrown. Similarly, if the value passed in for the length is less than 1, an IllegalArgumentException should be thrown. The numHits field should be explicitly initialized to 0.
Implement the basic accessor methods (2 points) Next, define the following accessor methods:
getType, which returns the string representing the Ship objects type getLength, which returns the integer representing the Ship objects length getNumHits, which returns the integer representing the number of times that the Ship object has been hit getSymbol, which returns the first character of the ships type as a char. For example, if the ships type is "Cruiser", this method should return 'C'. This method will be used when displaying a ship on one of the boards. Make sure that your methods are non-static, because they need access to the fields in the called object. In addition, none of these methods should take an explicit parameter, because all of the information that they need is inside the called object.
Once you have completed parts 14, you can test them using the first client program that weve given you. See below for more detail.
Implement the remaining Ship methods (4 points) Next, add the following methods:
a mutator method called applyHit that takes no parameters and that increments the Ship objects number of hits, increasing it by 1. This is the only mutator method in the class, because the values of the other fields cannot change after they are initialized. You do not need to worry about whether a ship has already been sunk. You can simply increment the field that represents the number of hits.
an accessor method named isSunk that takes no parameters and returns true if the Ship object has been sunk, and false if it has not been sunk. A Ship is sunk if its number of hits is greater than or equal to its length.
a toString method that returns a string representation of the Ship object of the form type of length length (e.g., "Battleship of length 4" or "Cruiser of length 3"). We discuss this type of method in the lecture notes, and we provide an example in our Rectangle class.
Make sure that your methods are non-static, because they need access to the fields in the called object.
Client programs and testing your code To help you in testing your Ship class, we have created two sample client programs:
ShipClient1.java should compile after completion of part 4. If it doesnt, check your method headers. tests the methods from parts 3 and 4 expected output is given here ShipClient2.java should compile after completion of part 5. Do not open it before then! tests the methods from part 5 expected output is given here Put the client programs in the same folder as your Ship.java file. Make sure not to open the clients until the necessary parts have been completed.
In addition to using the client programs, we recommend that you perform additional testing on your own. You can do so by adding code to one of the clients or by adding a main method to the Ship class.
If you are unable to get a given method to compile, make sure to comment out the body of the method (keeping the method header and possibly a dummy return value) so that well still be able to test your other methods.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
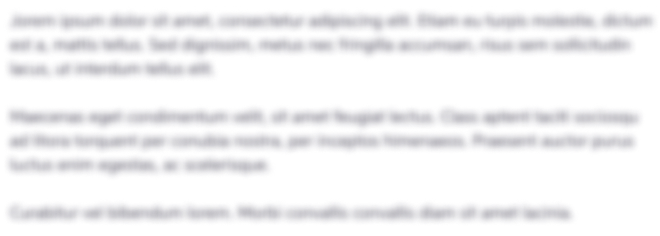
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started