Question
Problem Build a simple calculator that allows the user to specify a sequence of arithmetic operations (add, subtract, multiply, divide) and perform each of them
Problem
Build a simple calculator that allows the user to specify a sequence of arithmetic operations (add, subtract, multiply, divide) and perform each of them on two operands. The user enters 1 for addition, 2 for subtraction, 3 for multiplication, and 4 for division. If the user enters 5, the program exits.
The program must work as in the following sample execution.
Sample Execution
Here is a sample execution.
= RESTART: /Users/mh6624pa/Documents/Classes/ics 140/Summer 2017/Labs/L9.py = Enter 1 to add
Enter 2 to subtract
Enter 3 to multiply
Enter 4 to divide
Enter 5 to exit
Enter a value between 1 and 5 0
Enter a value between 1 and 5 6
Enter a value between 1 and 5 1 I will add.
Enter the numbers 4, 2 4 + 2 = 6.
Enter 1 to add
Enter 2 to subtract
Enter 3 to multiply
Enter 4 to divide
Enter 5 to exit
Enter a value between 1 and 5 2 I will subtract.
Enter the numbers 6, -8 6 - -8 = 14
Enter 1 to add
Enter 2 to subtract
Enter 3 to multiply
Enter 4 to divide
Enter 5 to exit
Enter a value between 1 and 5 3 I will multiply.
Enter the numbers 3, -5 3 * -5 = -15
Enter 1 to add
Enter 2 to subtract
Enter 3 to multiply
Enter 4 to divide
Enter 5 to exit
Enter a value between 1 and 5 4 I will divide.
Enter the numbers 2, 3 2 / 3 = 0.6666666666666666
Enter 1 to add
Enter 2 to subtract
Enter 3 to multiply
Enter 4 to divide
Enter 5 to exit
Enter a value between 1 and 5 5 I will exit >>>
Design and Coding
Proceed to program design and development.
3.1 Design
Here is part of the implementation and design. Complete the code. You could download Lab 9.zip from the D2L site to get this document as well as the starter program.
1. Create a list named operations that lists the four operations and the exit option. The entries must correspond to the displays shown in the sample execution.
operations = ["add", ....]
2. Create a method for each of the four arithmetic operations. Here is a model.
def add(number1, number2):
return number1 + number2
Code the other three with appropriate names.
3. Create a function named getOperation with no parameters. It should have the
following Python code in it.
for index in range(len(operations)):
print("Enter", str(index + 1), "to", operations[index]) opcode = eval(input("Enter a value between 1 and 5 ")) while opcode < 1 or opcode > 5:
opcode = eval(input("Enter a value between 1 and 5 ")) return opcode
4. Create a function named process with no parameters. Part of the Python code for this is given below. Convert the pseudocode to Python to complete the function.
while True:
opcode = getOperation() operation = operations[opcode - 1] if opcode == 5:
print("I will", operation) break number1, number2 = eval(input("I will " + operation + ". Enter the numbers ")) if opcode is 1
call the add function with number1 and number2 as params and print the result else if opcode is 2
call the subtract function with number1 and number2 as params and print the result else if opcode is 3
call the multiply function with number1 and number2 as params and print the result else if opcode is 4
call the divide function with number1 and number2 as params and print the result
5. Call the function process.
**********
# complete the following list operations = ["add", "subtract", "multiply", "divide"] def add(number1, number2): return number1 + number2
# define the other arithmetic functions here
# function header for getOperation with no parameters for index in range(len(operations)): print("Enter", str(index + 1), "to", operations[index]) opcode = eval(input("Enter a value between 1 and 5 ")) while opcode < 1 or opcode > 5: opcode = eval(input("Enter a value between 1 and 5 ")) return opcode
# function header for process with no parameters while True: opcode = getOperation() operation = operations[opcode - 1] if opcode == 5: print("I will", operation) break number1, number2 = eval(input("I will " + operation + ". Enter the numbers ")) ''' if opcode is 1: call the add function with number1 and number2 as params and print the result else if opcode is 2: call the subtract function with number1 and number2 as params and print the result else if opcode is 3: call the multiply function with number1 and number2 as params and print the result else if opcode is 4: call the divide function with number1 and number2 as params and print the result '''
Step by Step Solution
There are 3 Steps involved in it
Step: 1
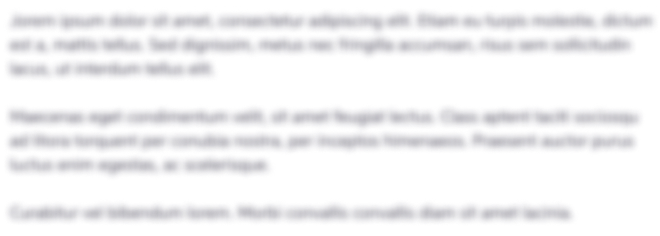
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started