Question
Problem description: An Internet service provider has three different subscription packages for its customers. Package Charges: Package Price per month Maximum hours for package Price
Problem description:
An Internet service provider has three different subscription packages for its customers. Package Charges:
Package | Price per month | Maximum hours for package | Price per hour over maximum |
Package A | $9.95 | 10 | $2.00 |
Package B | $13.95 | 20 | $1.00 |
Package C | 19.95 | unlimited | Not applicable |
Your program will consist of 2 classes: DukesISP.java and ISPCharge.java.
ISPCharge.java will handle the calculations for how much to charge given the package chosen the hours used and will calculate the tax on these charges at the rate of 5.5%.
DukesISP.java will contain a single main() method and will handle all user interaction. Here you will prompt the user for which package should be selected and the number of hours used. This information will be sent via the constructor to ISPCharge. DukesISP will get the total cost and tax from ISPCharge and will print a bill stating the package code, the number of hours actually used, with the cost broken down by maximum hours, and additional hours and costs. The format is specified below under "Output".
In addition, if the user has chosen either package A or B, the bill should display a message how much money the user could have saved under either of the other two plans. This savings should be displayed as the non-taxed amount. If there would be no savings or if the user is currently using Package C, then don't display a message.
Prerequisites
- You understand the material in Chapter 5 of "Think Java".
- You have downloaded ISPCharge.javaLinks to an external site..
Program Behavior
Your application must do these things:
- DukesISP: Prompt for the letter of the customer's package, A, B, or C and read in the value as specified below under "Output".
- You must allow for either upper case or lower case letters. 'A' and 'a' are equivalent.
- If a package other than A, B, or C is specified, display the error message specified below under "Output." Reprompt the user and reread a value from the user. The second prompt should be the same as the first.
- If a package other than A, B, or C is specified a second time, display an error message and exit the program.
- DukesISP: Prompt for the number of hours of usage. This number may be whole hours or parts of hours expressed as a floating point (real) number.
- If a negative number is entered (0 is ok) display the error message specified below under "Output." Reprompt the user and reread a value. The second prompt should be the same as the first.
- If something other than a positive (or 0) number is entered a second time, display an error message and exit the program.
- Once the user has entered all required values, instantiate an ISPCharge object with these values. DukesISP will call methods from the ISPCharge object to get the values to print. These include:.
- the total cost for the package and the hours used based on the chart above (Package charges). As is common these days, you should interpret the hourly charge as standing for hours or any part of an hour. 2.05 hours, for example, should be billed at 3 hours.
- the tax on the total cost calculated using a 5.5% rate.
- Calculate the total bill and display the amounts as specified below.
- Compare the total charge with the charge for the other higher packages (compare A to B and C; compare B to C) and display the amount saved, if any, of moving to the higher package.
Output The following output is required. These are not suggestions.
- Display the heading: "DukesISP Billing"
- Display a blank line.
- Prompt for the input values. Note: your input will be on the same line as the prompt.
- Your first input prompt must be the String: "Enter the package code (A, B, or C): "
- Your second input prompt must be the String: "Enter the number of hours used: "
- Error messages. If either of the first two values are invalid, display the message: "Your entry, " + badentryValue + "is invalid. Please try again." where badentryValue is the erroneous value that was entered by the user. You should then display the original prompt on a new line and get the user's response.
- After reading all of the input values, display a blank line and the bill as follows. The bold values are calculated values as described above. All monetary amounts should be displayed with the currency symbol ($ for US) and decimal point (2 decimal places). The hours should be displayed to 3 decimal places. All lines should be printed at the left-hand edge (do not indent).
DukesISP Billing [blank line] Package: packageCode Hours Used: hours [blank line] Base Charge: base Base Hours: baseHours (omit this line for Package C) Additional Hours: hoursOver (hours - baseHours. Omit this line for Package C) Total Charge: charge Tax: taxCharge Pay this Amount: totalBill
- If the user is using package A or B and they could save money on package B or C, display a blank line and then the following message as appropriate. (Display two messages if two packages will provide savings.) If there is no savings or the savings would be on a lower base cost package, do not display the message. All lines should be printed at the left-hand edge. Do not indent.
[blank line] You could have saved savings with package packageCode. Call 1-888-555-1234 for more information.
- Here are a series of sample outputs for various scenarios, including the values entered by the user for each package along with error handling: pa03-output-examples.txtLinks to an external site.
Additional Program Requirements
- You must have a minimum of two classes, and these must be named DukesISP.java and ISPCharge.java. DukesISP will hold the main method and ISPCharge will represent the charges.
- All input and output will be done in the main() method of DukesISP. This code will use the methods in the ISPCharge object, which will handle all of the calculations.
- You must use the ISPCharge.java file that is provided here, and you must fill out all of the listed methods. Method headers must not be changed.
- You may additional classes if you want and you may add methods to ISPCharge if you want.
- You may use the Keyboard class from Lab9 instead of the Scanner class if you want as well.
- ISPCharge will represent the charge for one customer. It will store the package and hours, then use these values to calculate the various parts of the bill.
- You must use the variable names given in bold above. If you create additional variables, name them appropriately (see the Style GuideLinks to an external site.).
- You must use named constants (final) as appropriate.
- You must display any error messages directly after the incorrect input. For example, if the user enters an incorrect package code, you must immediately display the error message and reprompt for input.
- Your program must include an acknowledgment section acknowledging help received from your professor or other reference sources.
- If you received no help your acknowledgment section should have a statement to that effect.
- Your acknowledgement section must adhere to the format specified in the Style GuideLinks to an external site. . Failure to include the acknowledgment statement as specified in the Style Guide will result in a 25% reduction in your grade for PA3.
- Your program must conform to standard Java programming standards and the additional standards for this class as specified in the Style GuideLinks to an external site..
Procedure
- Understand the problem. Make sure that you understand how the package costs will be determined, how tax will be calculated, how to consider and display the alternative billings, and how the user interface should appear. You have 2 classes that will work together to produce the final result. Don't be tempted to put this all in a single class (or method).
- Create "stubs" in ISPCharge and build a driver to test each method in isolation.
- Design each of the methods before you code any of them. What do you need to do, for example, to calculate the charge for package A? What must you do to compare the charges?
- Implement each method.
- Test each of these methods using your driver before you try to incorporate them in the final working program.
- Design and implement DukesISP. What must happen first, second or third? Don't worry about error checking or the comparison part until you have it working with "expected" data and can produce a basic bill.
- Test each part thoroughly as you are building it.
- Pay attention to the interface. Make sure that your output conforms to the specifications above. Ask questions if there is any ambiguity.
- Document as you are going along. The methods in ISPCharge should be updated to reflect the actual code you implement. Document your steps in the main method.
- Above all, begin early. Students run into trouble by waiting too long to start the program.
- Make sure that you follow the requirements precisely. Don't add additional "flourishes". You will be downgraded.
ISPCharge.java /** * Put your class documentation here. See the Style Guide for format. */ public class ISPCharge { // declare your finals here. They should be public and static // instance variable declarations private char packageCode; private double hours; /** * Explicit value constructor. * * @param pkg The code for the package, A, B, or C * @param hours The number of hours this month */ public ISPCharge( char pkg, double hrs ) { } /**************************** public methods **************************/ /** * Decides which package to apply and returns the correct cost. * * @return The charges for this month. */ public double calcCost() { // calculate the cost using the private methods below return -1.0; // stub return, replace this } /** * Calculates the tax on the passed charge. * * The rest is left for you to fill in.... */ public double calcTax() { // put the calcTax method here return -1.0; // stub return, replace this } /** * Describes this charge. It should include the package for this * charge and the hours. For example; * * Package A (5 hours) * * the rest is left for you to fill in.... */ public String toString() { // put the toString method here return ""; // stub return, replace this } /*************************** private methods **************************/ /** * Calculates the charges for package A. * * The rest is left for you to fill in.... */ private double calcA() { // put the calcA method here return -1.0; // stub return, replace this } /** * Calculates the charges for package B. * * The rest is left for you to fill in.... */ private double calcB() { // put the calcB method here return -1.0; // stub return, replace this } /** * Calculates the charges for package C. * * The rest is left for you to fill in.... */ private double calcC() { // put the calcC method here return -1.0; // stub return, replace this } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
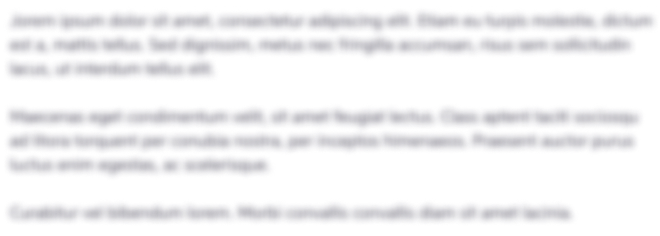
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started