Question
Problem Description: Calculate final grade and corresponding letter grade For this Lab, you will have to write a java program that asks the user to
Problem Description: Calculate final grade and corresponding letter grade For this Lab, you will have to write a java program that asks the user to enter homework grade, then check if the entered grade is valid (between 0 and 100) for homework and midterm, and (between 0 and 200) for finalexam. The program will calculate the final grade of the student, using the input the formula: Final Grade= ((Final_Exam/200)*50)+(Midterm_Exam*25%)+(Homework*25%) A letter will be calculated based on finalGrade, if student passes class value should be 'P', if not value should be 'F'. If the input grade is not valid, end program with proper message. Please follow these guidelines and ask your TA for help if needed. You may also collaborate with your classmates if needed. Step 1: Getting Started Create a class called Lab3. Use the same setup for setting up your class and main method as you did in previous labs and assignments. Be sure to name your file Lab3.java. Assignments Documentation: At the beginning of each programming assignment you must have a comment block with the following information: /*------------------------------------------------------------------------- // AUTHOR: your name. // FILENAME: title of the source file. // SPECIFICATION: your own description of the program. // FOR: CSE 110- Lab #3 // TIME SPENT: how long it took you to complete the assignment. //-----------------------------------------------------------*/ Step 2: Declaring Variables Copy the code provided below, make sure you understand it and it has no errors. Remember to take care of indentation. // All "import" lines must be before class import java.util.Scanner; // class name should match the file name public class Lab3 { // we must have a main method to run the program public static void main(String[] args){ // declaring variables: double finalexamGrade = 50.0; double midtermGrade = 50.0; double homeworkGrade = 0.0; double finalGrade = 0.0; // a character called letterGrade char letterGrade = ' '; // a variable scan of type Scanner Scanner scan = new Scanner(System.in); Step 3: User input and validation Write a segment of code where you will ask the user to provide value to homeworkGrade variable defined in previous section. // Print a statement to the user asking for // student's homework grade //--> // Use the Scanner class to ask the user inputs //for 'homeworkGrade','midtermGrade' and 'finalexamGrade'. // --> Now it's time to validate user input. It's logical to assume that user might have entered a wrong grade value; that is a negative number or higher than limit. It is the responsibility of a good programmer to take care of these cases by validating input taking from user. In this lab we will check (using if statement) if the grade entered is valid, if so (true-condition) we will continue calculating the final grade, if not (else statement) we will print a proper exit message to tell the user about his mistake. if(/* put condition here */){ // Case of True condition (correct input) //Continue this part from "Step 4" below } else { // Case of false condition (wrong input) //Continue this part from "Step 6" below } Step 4: Calculate "finalGrade" Now that we made sure our input is fine, this part of code will be inside the "if-close" from previous section. We can calculate the final grade using the formula shown above. //write the formula to calculate the final grade //and store the result in the finalGrade variable //--> Step 5: "if statement" - finding grade letter and Checking the grade In this section we will use if statement to find the appropriate grade letter. If the student has a final score of more than or equal 50, letter should be 'P' to indicate he passed the class. If not (else), letter should be 'F' to indicate he is failed the class. Write your code in this section, go back to step 3 above for if-else syntax. Your condition will look like: finalGrade >= 50. //Checking the grade if(/*letterGrade is 'p'*/){ // Tell user that student have passed //--> } else if(/*letterGrade is 'F'*/){ // Tell user that student have failed //--> } Step 6: Proper exit message for wrong input Now we need to write code inside " else" statement from part 3. Print a string to the user explaining why his input was wrong. //Use System.out. println to explain what happened to the user. // Make sure your output looks similar to sample output below //--> NOTE: Keep track of your curly braces (blocks) in your code, each open brace should be closed. Your code must end with three closing curly braces like this: }// end of else statement }// end of main }// end of class Sample Output Below is an example of what your output should roughly look like when this lab is completed. Sample Run 1: Enter homework grade: 100 Enter midterm grade: 76 Enter final grade: 80 Student Passed the class Sample Run 2: Enter homework grade: 30 Enter midterm grade: 40 Enter final grade: 35 Student Failed the class Sample Run 3: Enter homework grade: 150 Enter midterm grade: 122 Enter final grade: 132 Invalid input. Homework and midterm grades should be between 0 and 100 and the final grade should be between 0 and 200
Step by Step Solution
There are 3 Steps involved in it
Step: 1
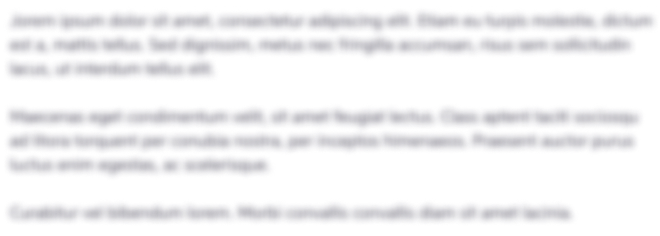
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started