Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Problem For this lab you will complete a program to play a very simplified version of the card game cribbage!. The game is played with
Step by Step Solution
There are 3 Steps involved in it
Step: 1
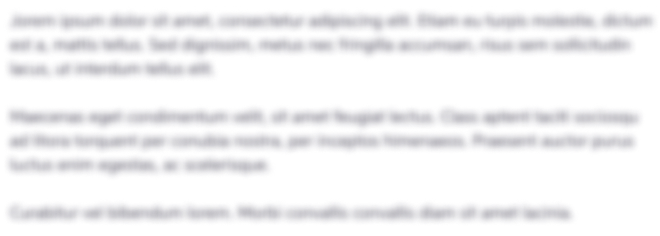
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started