Question
Problem Formulation Definition: Polymorphism is the ability of an object to take on many forms. The most common use of polymorphism in OOP occurs when
Problem Formulation
Definition: Polymorphism is the ability of an object to take on many forms. The most common use of polymorphism in OOP occurs when a parent class reference is used to refer to a child class object. Any Java object that can pass more than one IS-A test is considered to be polymorphic.
This time your task is to extend Assets application even further to implement the following two features:
User interface that would allow creation of various Asset objects (of classes defined in A8, i.e. Switch, Server, Router, etc) by means of using menu with predefined actions and user input;
Building up collection of Assets where all assets are linked with respect to their child-parent relationship.
The first feature assumes creation of a two-level menu, with the first level being responsible for collection manipulation actions, for example:
1. Add a new asset 2. Remove an existing asset 3. Display an existing asset 4. Quit
Note: Each menu item processed by a separate method of the Driver class. The second level would depend on which action has been selected in the first level menu. For example, adding of an asset might be associated with the following menu:
1. Specify type of asset to be entered 2. (Re-)Run type-specific asset addition process 3. Go to the previous menu
Notes: Specification of asset type has to be performed with user input validation, i.e. only supported asset types have to be allowed. Asset addition process assumes displaying of a set of asset type-specific prompts that would allow user to populate all required asset attributes. For example, consider the following interactive session (user input is emphasized with italic font shape) for server-type asset:
Enter ID of the asset (or "quit" to go to the previous menu): 105 Enter Name of the asset (or "quit" to go to the previous menu): ESXi-25 Enter Vendor of the asset (or "quit" to go to the previous menu): HP Enter Model of the asset (or "quit" to go to the previous menu): Proliant ML350 G6 Enter S/N of the asset (or "quit" to go to the previous menu): USE3256V23 Enter ID of the parent asset or -1 if asset doesn't have a parent (or "quit" to go to the previous menu): -1 Enter the type operating system running on the server: Vmware ESXi v6.0 U2 Enter the number of installed NICs: 3 Is this server virtualized [yes/no] (or "quit" to go to the previous menu): no
The last 3 questions are highlighted in blue to emphasize that they are only asked for server-type assets. After answering all 9 questions, the second-level menu has to be redisplayed to allow user to make corrections (if needed) or jump to the upper level menu.
The second feature assumes storing all entered assets in a collection where child-parent relationship is used to construct a two-level tree of all assets:
Assets listed on the parent level do not have parents but may have children
Assets listed on the child level are associated with the same parent.
Here is an example of such tree:
Asset{ID=101, type=SERVER, name='ESXi-25', vendor='HP', model='Proliant ML350 G6', sn='USE3256V23', PID=-1} Asset{ID=501, type=PSU, name='Primary PSU of ESXi-25', vendor='HP', model='HSTNS-PL18', sn='1245002933', PID=101} Asset{ID=502, type=PSU, name='Secondary PSU of ESXi-25', vendor='HP', model='HSTNS-PL18', sn='1245002940', PID=101} Asset{ID=201, type=SWITCH, name='Main switch', vendor='Cisco', model='C2960S-48LPS-L', sn='FOC1512Z0KJ', PID=-1}
Please see my code so far.
Asset.java
import java.util.*; import java.io.*;
public class Asset { // defining private attributes private String type; private int ID; private String name; private String serial; private int PID;
// default constructor public Asset() { this.type = ""; this.ID = 0; this.name = ""; this.serial = ""; this.PID = -1; // Asset needs to be assigned a parent else it gets a parent id value of -1 }
public Asset(String type, int ID, String name, String serial, int PID){ this.type = type; this.ID = ID; this.name = name; this.serial = serial; this.PID = PID; // Asset needs to be assigned a parent else it gets a parent id value of -1 }
// Setter method for type public void setType(String type) { this.type = type; }
// Setter method for ID public void setId(int id) { this.ID = id; } // Setter method for name public void setName(String name) { this.name = name; } // Setter method for serial public void setSerial(String serial) { this.serial = serial; } // Setter method for PID public void setPID (int pid) { this.PID = pid; }
// Getter method for type public String getType() { return this.type; }
// Getter method for id public int getId() { return this.ID; }
// Getter method for name public String getName() { return this.name; }
// Getter method for serial public String getSerial() { return this.serial; }
// Getter method for PID public int getPID() { return this.PID; }
// Method to return the Asset details @Override public String toString() { String str = "Type: " + this.type + " " + "ID: " + this.ID + " "+ "Name: " + this.name+ " "+ "Serial: " + this.serial+ " " + "PID: " + this.PID + " " ;
return str; } }
Switch.java
import java.util.*; import java.io.*;
public class Switch extends Asset { // Defining attributes int numOf_100M; // number of 100Mb ethernet interfaces int numOf_1G; // number of 1Gb ethernet interfaces int numOf_10G; // number of 10Gb ethernet interfaces int numOf_SFP; // number of SFP modules String OS; // operating system. including vendor, version, and other details String level; // one from the following list "L2", "L3", "L4"
// Default constructor public Switch(String type, int ID, String name, String serial, int PID, int numOf_100M, int numOf_1G, int numOf_10G, int numOf_SFP, String oS, String level) { super(type, ID, name, serial, PID); this.numOf_100M = numOf_100M; this.numOf_1G = numOf_1G; this.numOf_10G = numOf_10G; this.numOf_SFP = numOf_SFP; OS = oS; this.level = level; } // Setter method for number of 100Mb ethernet interfaces public void setNumOf_100M(int numOf_100M) { this.numOf_100M = numOf_100M; } // Setter method for number of 1Gb ethernet interfaces public void setNumOf_1G(int numOf_1G) { this.numOf_1G = numOf_1G; } // Setter method for number of 10Gb ethernet interfaces public void setNumOf_10G(int numOf_10G) { this.numOf_10G = numOf_10G; } // Setter method for number of SFPs public void setNumOf_SFP(int numOf_SFP) { this.numOf_SFP = numOf_SFP; } // Setter method for OS public void setOS(String oS) { OS = oS; } // Setter method for level public void setLevel(String level) { this.level = level; } // Getter method for number of 100Mb ethernet interfaces public int getNumOf_100M() { return numOf_100M; } // Getter method for number of 10Gb ethernet interfaces public int getNumOf_1G() { return numOf_1G; }
// Getter method for number of 10Gb ethernet interfaces public int getNumOf_10G() { return numOf_10G; } // Getter method for number of SFPs public int getNumOf_SFP() { return numOf_SFP; }
// Getter method for OS public String getOS() { return OS; }
// Getter method for level public String getLevel() { return level; }
// Method overrides or adds details to the switch asset @Override public String toString() { return "Switch: numOf_100M: " + numOf_100M + " numOf_1G: " + numOf_1G + " numOf_10G: " + numOf_10G + " numOf_SFP: " + numOf_SFP + " OS: " + OS + " level: " + level + " " + super.toString(); }
}
Router.java
import java.util.*; import java.io.*;
public class Router extends Asset { //Defining attributes String application; // one from the following list "Home", "Core", "Infrastructure", etc int numOfNICs; // number of network interfaces String OS; // operating system. including vendor, version, and other details boolean hasConsole; // flag that indicates presence of management console
// Default constructor public Router(String type, int ID, String name, String serial, int PID, String application, int numOfNICs, String oS, boolean hasConsole) { super(type, ID, name, serial, PID); this.application = application; this.numOfNICs = numOfNICs; OS = oS; this.hasConsole = hasConsole; }
// Setter method for application public void setApplication(String application) { this.application = application; }
// Setter method for number of NICs public void setNumOfNICs(int numOfNICs) { this.numOfNICs = numOfNICs; } // Setter method for OS public void setOS(String oS) { OS = oS; }
// Setter method for hasConsole public void setHasConsole(boolean hasConsole) { this.hasConsole = hasConsole; } // Getter method for application public String getApplication() { return application; } // Getter method for number of NICs public int getNumOfNICs() { return numOfNICs; } // Getter method for OS public String getOS() { return OS; } // Getter method for hasConsole public boolean isHasConsole() { return hasConsole; } // Method overrides or adds details to the route asset @Override public String toString() { return "Router: Application: " + application + " numOfNICs: " + numOfNICs + " OS: " + OS + " hasConsole: " + hasConsole + " " + super.toString(); } }
Server.java
import java.util.*; import java.io.*;
public class Server extends Asset { //Defining attributes String OS; // same as with the Router int NumOfNICs; // same as with the Router boolean isVirtualized; // flag that shows whether or not this server is a Virtual Machine running on some virtualization platform // Default constructor public Server(String type, int ID, String name, String serial, int PID, String oS, int numOfNICs, boolean isVirtualized) { super(type, ID, name, serial, PID); OS = oS; NumOfNICs = numOfNICs; this.isVirtualized = isVirtualized; } // Setter method for OS public void setOS(String oS) { OS = oS; } // Getter method for OS public String getOS() { return OS; }
// Setter method for number of NICs public void setNumOfNICs(int numOfNICs) { NumOfNICs = numOfNICs; } // Getter method for number of NICs public int getNumOfNICs() { return NumOfNICs; } // Setter method for is virtualized? public void setVirtualized(boolean isVirtualized) { this.isVirtualized = isVirtualized; } // Getter method for is virtualized? public boolean isVirtualized() { return isVirtualized; }
// Method overrides or adds details to the server asset @Override public String toString() { return "Server: OS: " + OS + " NumOfNICs: " + NumOfNICs + " isVirtualized: " + isVirtualized + " " + super.toString(); } }
Driver.java
import java.util.*; import java.io.*;
public class Driver { public static void main(String[] args) {
// Stores Server1 asset Asset server1 = new Server("Data Server", 1002, "SkyWalker Server","WF25D785FC", 100, "Windows NT", 20, true); server1.setId(200); ///changing the id // Prints Server1 asset System.out.println(server1.toString());
// Stores Server2 asset Asset server2 = new Server("Web Server", 1003, "Juniper Server","WF85G359IC", 100, "Oracle Linux 7", 10, true); server2.setId(201); ///changing the id // Prints Server1 asset System.out.println(server2.toString());
// Stores Router2 asset Asset router2 = new Router("Smart WiFi Dual Band Gigabit Router", 9856, "NETGEAR Nighthawk X4S - AC2600", "SNR25OP984NJ", -1, "Infrastructure", 2, "Nas OS", true); // Prints Router2 asset System.out.println(router2.toString());
// Stores Switch asset Asset Switch = new Switch("Fast Ethernet Smart Managed Switch", 4823, "NETGEAR ProSAFE FS526T", "SNR57OC458GL", -1, 20, 21, 25, 40, "Windows NT", "L3"); // Prints Switch asset System.out.println(Switch.toString()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
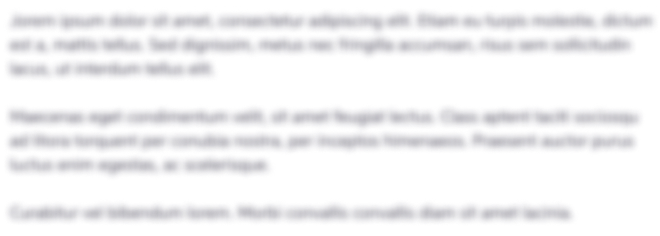
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started