Question
Problem: Given input from the user about a car (MPG, miles to destination, and cost per gallon), determine the cost of the trip. Allow only
Problem: Given input from the user about a car (MPG, miles to destination, and cost per gallon), determine the cost of the trip. Allow only positive numbers.
This is the algorithm:
Display the Introduction
Read in name of traveler as a string
Read in the destination as a string
Read in model of vehicle as a string
Read in MPG as a double
Read in miles to destination as a double
Read in cost per gallon as a double
Determine cost per trip (based on MPG, miles to destination, and cost per gallon)
Print out a chart with the name, destination, etc.
1) Create a method called intro that displays the introduction to the program as in: ============================================================ Trip Calculator This program will request information about your vehicle and
destination. It will compute the number of gallons of gas you
will need for your trip. ============================================================ 2) Create a method called getString that will read in Strings as in: Please enter the name of the traveler: John Please enter the destination: Walt Disney World Please enter the car model: Explorer
The method is sent: "name of the traveler" or "name of the car" or "destination"
The method reads in the String
The method returns the String that was read in
In the main: String name = getString("the name of the traveler");
3) Create a method called getDouble that will read in doubles as in: Please enter the MPG for this car: 14.6 Please enter the miles to destination: 1200
Please enter the cost per gallon: 3.62
The method is sent: "MPG for this car" OR "miles to destination" OR "cost per gallon"
The method reads in the double
If the number is less than or equal to zero, the method asks again and again until a positive number is entered
The method returns the double that was read in
In the main: String name = getDouble("the miles per gallon"); 4) Write a main method to finish the class to include calling the methods that will create the following in the terminal window: ============================================================ Trip Calculator This program will take a given mpg, destination, and miles, will determine the gallons of gas needed for the trip. ============================================================ Please enter the name of the traveler: John Please enter the destination: Walt Disney World Please enter the miles to destination: 1200 Enter the information about the car... Please enter the car model: Explorer Please enter the MPG for this car: 14.6
Please enter the cost per gallon: 3.29 ============================================================ Traveler: John Trip Calculator to: Walt Disney World Miles: 1200.0 Cost Per Gallon: 3.62 Model Car MPG Cost for round trip ============================================================ Explorer 14.6 $270.41
Extra credit option (3 points maximum extra credit) Modify the main method to execute the same calculations for 3 different trips and keep the cost per round trip saved in 3 separate variables. Create another method that takes the resulting costs per round trip of the 3 trips and determines which trip was the least expensive and print a message similar to:
The least expensive trip cost $324.56
Step by Step Solution
There are 3 Steps involved in it
Step: 1
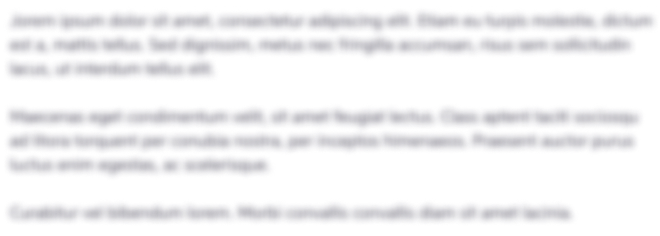
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started