Question
Problem: I am trying to implement a binary search function in Visual Studio 2019 using C#. This is my first program in C# and I
Problem:
I am trying to implement a binary search function in Visual Studio 2019 using C#. This is my first program in C# and I translated it from Java.
In this program, I am to carry out the same 10,000,000 unsuccessful searches for eight different-sized arrays, namely arrays of sizes, 128, 512, 2048, 8192, 32768, 131072, 524288, and 2,097,152. I need to measure each of the three programs and the time it takes to do the 10,000,000 searches for each of the eight arrays. Compare the timings to the theoretical timings the algorithm binary search provides.
Please make corrections to my programs below so that it may successfully debug/run.
Code:
using System; class BinarySearch { int BinarySearch(int arr[], int 1, int r, int x) { if (r >= 1) { int mid = 1 + (r - 1) / 2; if (arr[mid] == x) return mid; if (arr[mid] > x) return BinarySearch(arr, 1, mid - 1, x); return BinarySearch(arr, mid + 1, r, x); } return -1; }
public class Problem5InC# {
public static void main(String[] args) {
BinarySearch obj = new BinarySearch(); int size = 2097152; int arr[] = new int[size]; for (int i = 0; i < size; i++) { arr[i] = 0; } int x = 1; int result = 0; long start = System.currentTimeMillis(); for (int i = 0; i < 10000000; i++) { result = obj.BinarySearch(arr, 0, size - 1, x); } long end = System.currentTimeMillis(); long elapsed_time = end - start; Console.write("elapsed_time"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
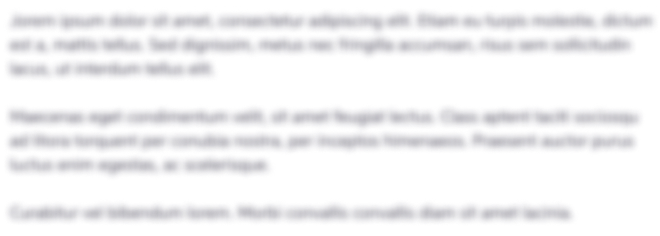
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started