Question
Problem: JavaScript Fundamentals In this exercise, you'll practice with fundamental JavaScript variables and syntax. To complete the exercise, edit the included js/index.js file to add
Problem: JavaScript Fundamentals
In this exercise, you'll practice with fundamental JavaScript variables and syntax.
To complete the exercise, edit the included js/index.js file to add in the code described in the comments.
You can see the results of your work either by opening up the included index.html file in a browser and viewing the output in the Developer Console, or by running the script directly using Node.js:
Starter js file
'use strict';
/** Basic Types */
//Define a new variable `motto` for the value "The iSchool is my school"
//Log out the motto
//Define a variable `mottoLength` that stores the length of the motto.
//Use the `.length` property
//Log out the length.
//Use the `indexOf()` String method to see if the word "cool" is in the string.
//See http://www.w3schools.com/jsref/jsref_obj_string.asp for String methods
//Log out a _boolean_ whether it is or not.
//Use a String method to replace the word "iSchool" in the `motto` variable with
//the words "Information School". The new value should be re-assigned to the
//`motto` variable.
//Log out the updated motto.
//Calculate the ratio between the length of the updated motto and the length of
//the old (which you had saved in a variable!). Log out this ratio as a
//percentage with two decimal places of precision (e.g., `"123.45%"`).
//You can use the `.toFixed()` Number method to specify the precision.
/** Arrays **/
//Create an array `numbers` that contains these 10 numbers:
// 1 4 1 5 9 2 6 5 3 5
//Log out the array.
//Use bracket notation to change the `4` in the array to a `4.2`.
//Log out the updated array.
//Add the number 3 to the END of the array.
//Log out the updated array.
//Find the median (middle) value of the numbers in the array.
//Hint: sort() the array, then access the middle index of the sorted values.
//You can use the `Math.floor()` function to round to a whole number.
//Log out the median value.
/** Objects **/
//Create a variable `rect` that represents a rectangle. This should be an Object
//with properties:
// `x` (coordinate) of 30, `y` of 50, `width` of 100, `height` of 50
//Log out the rectangle object
//Log out the x- and y- coordinates of the rectangle (its location). Your output
//should have the format `"X, Y"`.
//Set the rectangle's height to be the square root of its width. (Use the
//`Math.sqrt()` function).
//Use *dot notation* to access the properties!
//Log out the rectangle's area. Use *dot notation* to access the properties!
//Create a variable `circle` that represents a circle. This should be an object
//with properties:
// `cx` (center-x-coordinate) of 34,
// `cy` of 43,
// `radius` equal to the LAST value in the (sorted) `numbers` array.
//Log out the circle
//Create an array `shapes` that represents a list of shapes. The array should
//contain the rectangle and the circle objects defined above.
//Log out the variable. Be sure to inspect it in the developer console!
//Add a new ANONYMOUS object (e.g., one passed in directly without its own
//variable name) representing a right triangle to the `shapes` array.
//The triangle should have a `base` of 33 and a `height` of 44.
//Log out the updated shapes array.
//Log out the triangle's `hypotenuse` property (don't calculate it, just log out
//the current property value!). What do you get?
//Assign the triangle inside the `shapes` array a 'hypotenuse' property of `55`.
//Log out the `shapes` array again.
//Visually check: what happens if you inspect the previously logged array in the
//Chrome developer console?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
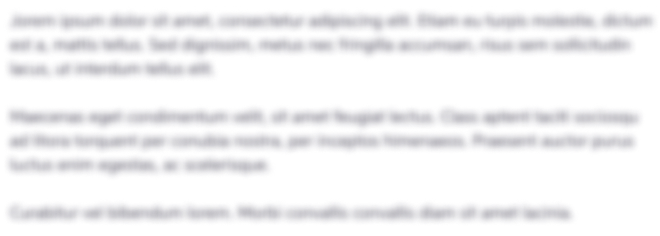
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started